マイロボット配置システム6
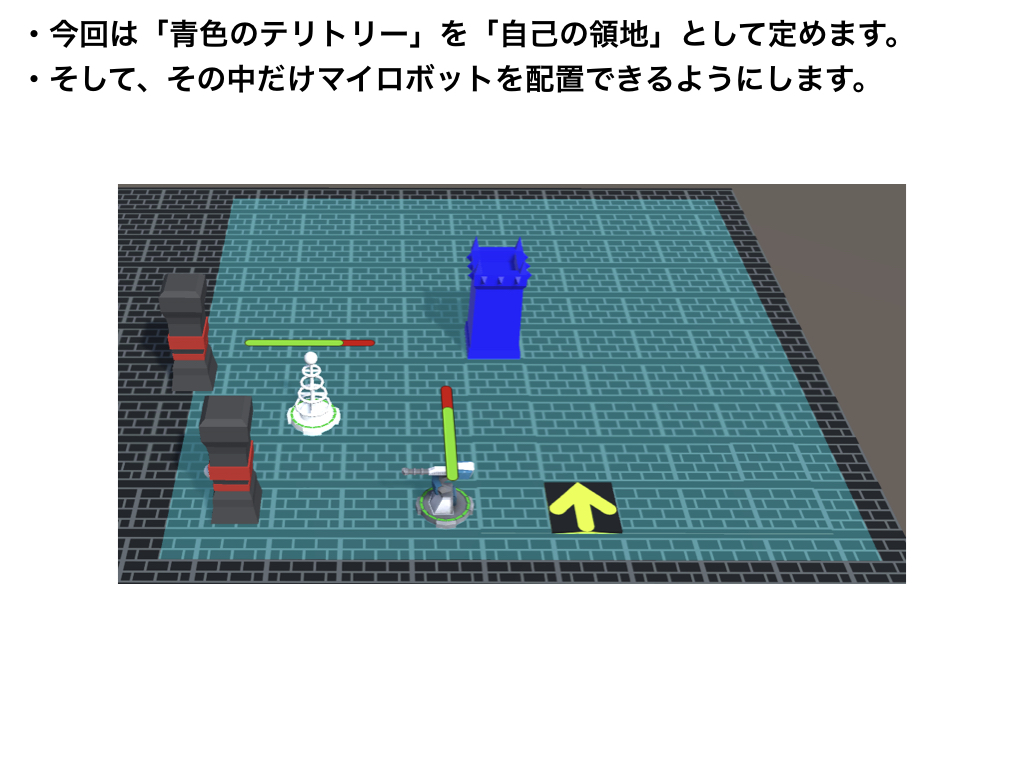
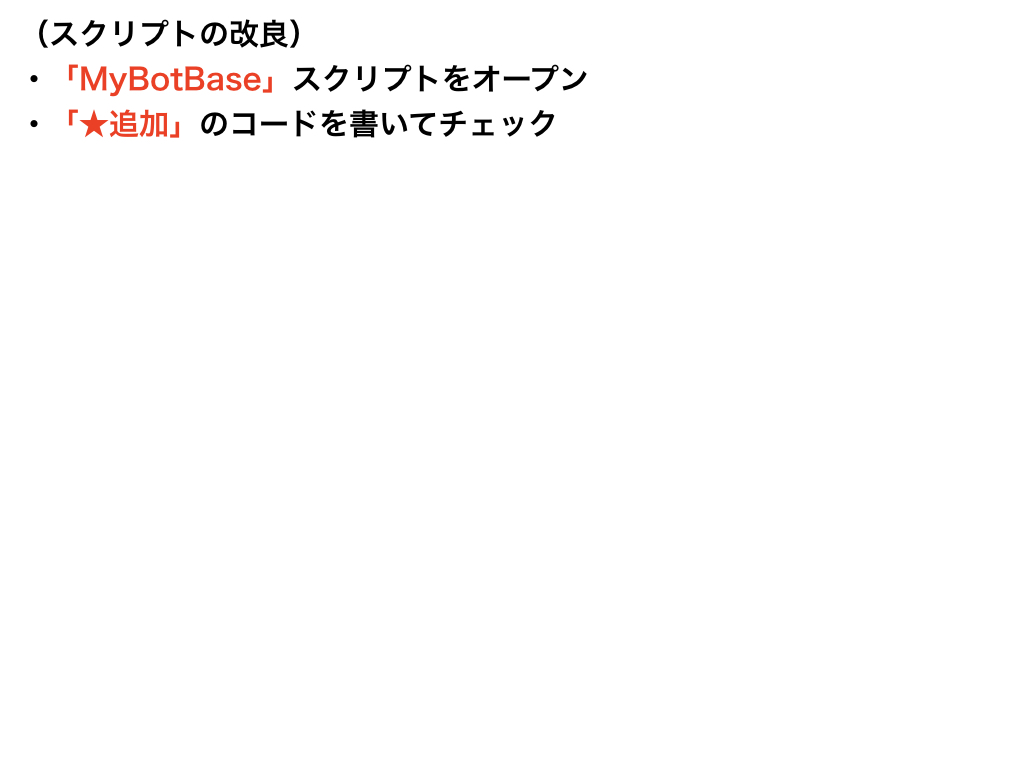
テリトリー内だけ矢印になる
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class MyBotBase : MonoBehaviour
{
private int num = 0;
// ★追加
public Material[] myMaterials;
public bool isSetOK;
// ★追加
private void Start()
{
GetComponent<MeshRenderer>().material = myMaterials[0];
isSetOK = false;
}
void Update()
{
Vector3 pos = transform.position;
if(Input.GetKeyDown(KeyCode.LeftArrow))
{
transform.position = new Vector3(pos.x - 1, 0, pos.z);
}
if (Input.GetKeyDown(KeyCode.RightArrow))
{
transform.position = new Vector3(pos.x + 1, 0, pos.z);
}
if (Input.GetKeyDown(KeyCode.UpArrow))
{
transform.position = new Vector3(pos.x, 0, pos.z + 1);
}
if (Input.GetKeyDown(KeyCode.DownArrow))
{
transform.position = new Vector3(pos.x, 0, pos.z - 1);
}
if (Input.GetKeyDown(KeyCode.RightShift))
{
num += 1;
transform.rotation = Quaternion.Euler(0, 90 * num, 0);
}
}
// ★追加
// (ポイント)
// Trigger系のメソッドを使うので、Is Triggerにチェックが必要!!
// 表示が切り替わらない場合には『MyBotBase』オブジェクトの『Box Collider』の『Is Trigger』にチェックが入っているか確認しよう!
private void OnTriggerStay(Collider other)
{
if(other.gameObject.tag == "BlueTeritory")
{
GetComponent<MeshRenderer>().material = myMaterials[1];
isSetOK = true;
}
else if(other.gameObject.tag == "RedTeritory")
{
GetComponent<MeshRenderer>().material = myMaterials[0];
isSetOK = false;
}
}
// ★追加
private void OnTriggerExit(Collider other)
{
if (other.gameObject.tag == "BlueTeritory")
{
GetComponent<MeshRenderer>().material = myMaterials[0];
isSetOK = false;
}
}
}
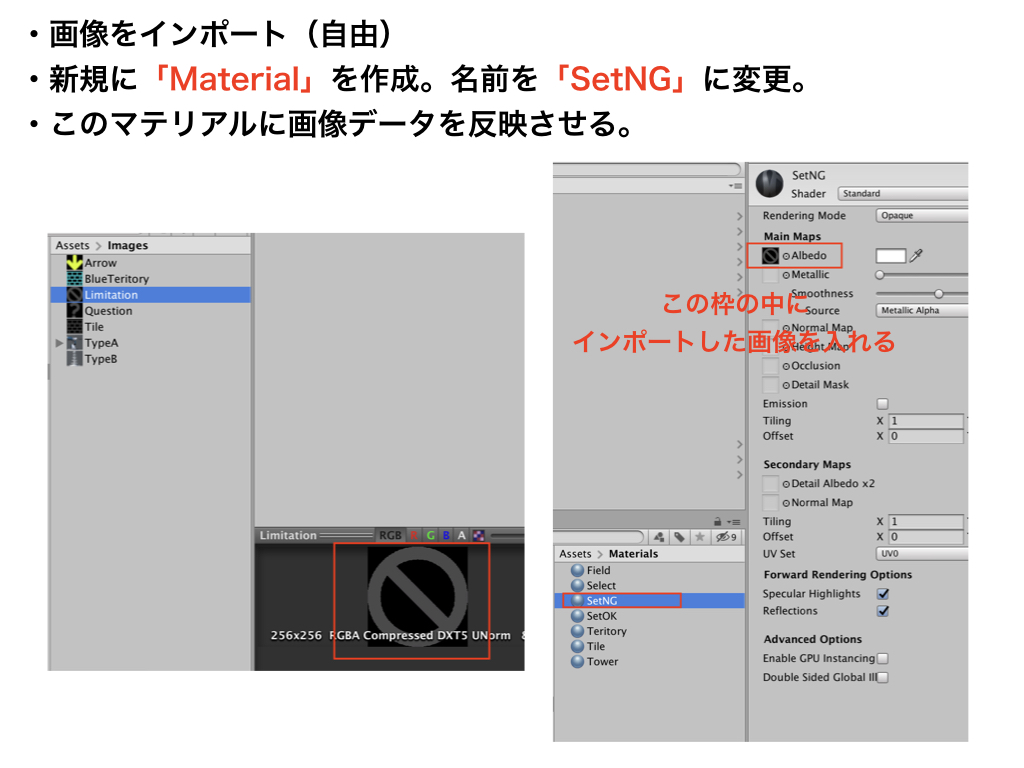
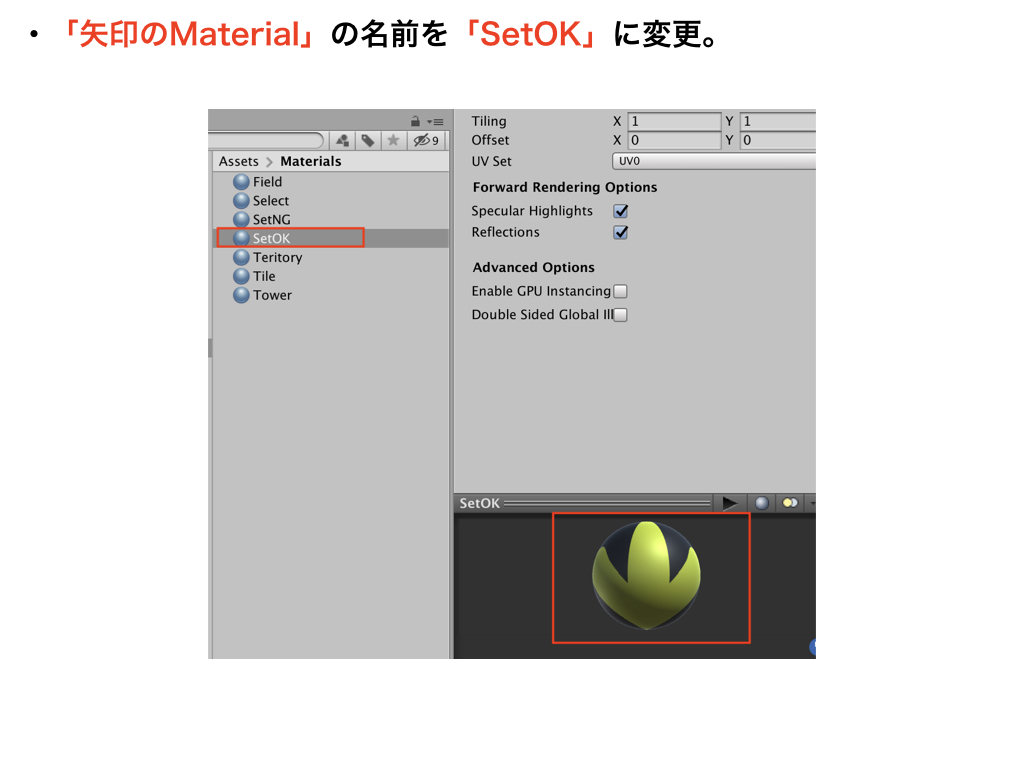
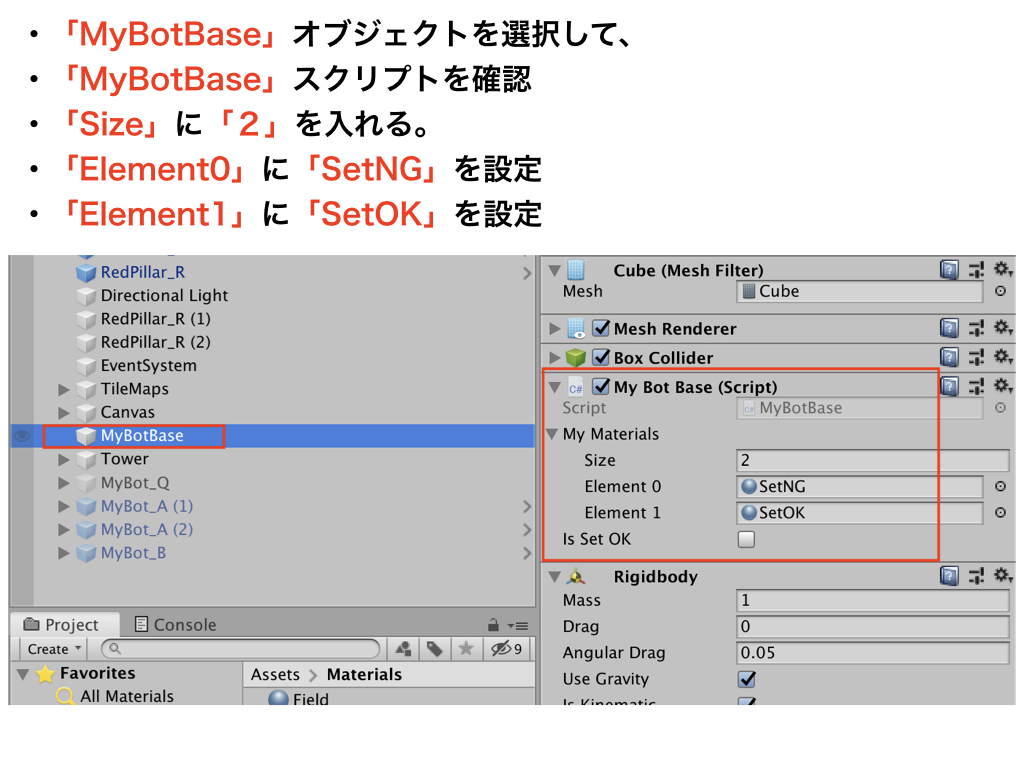
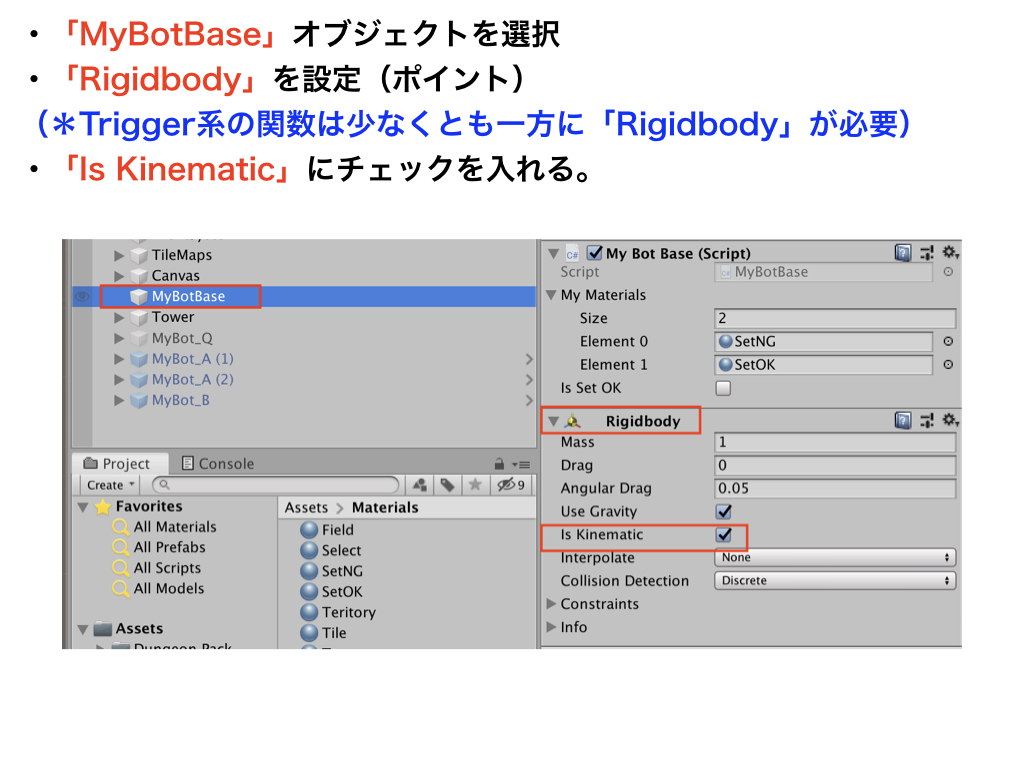
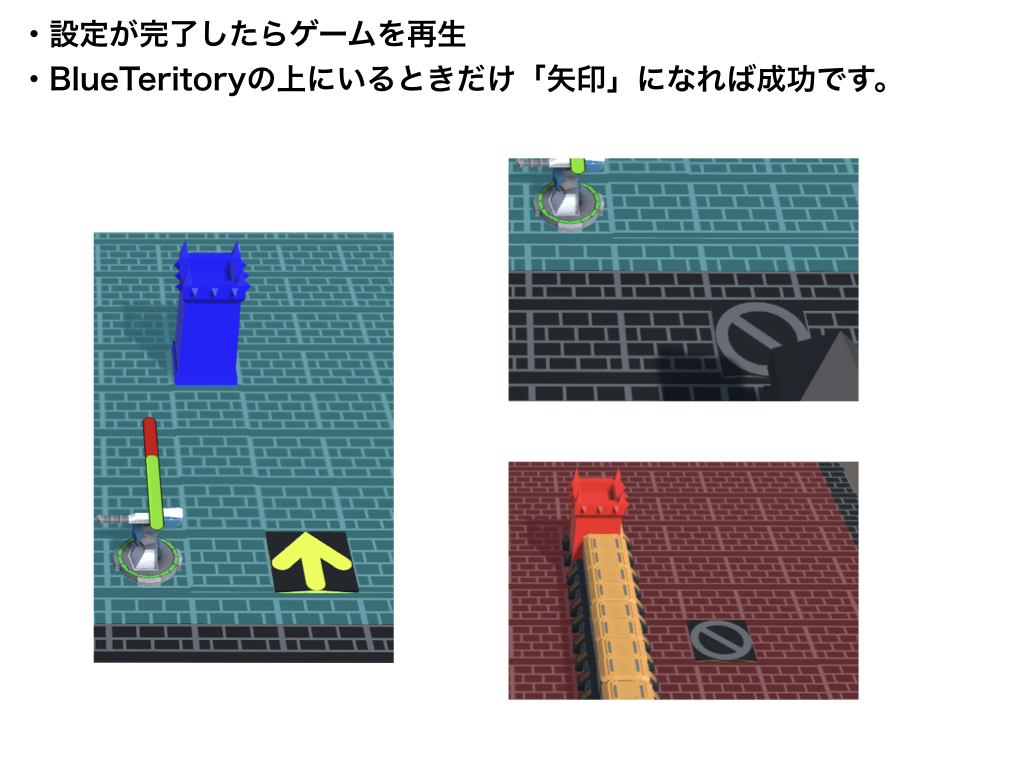
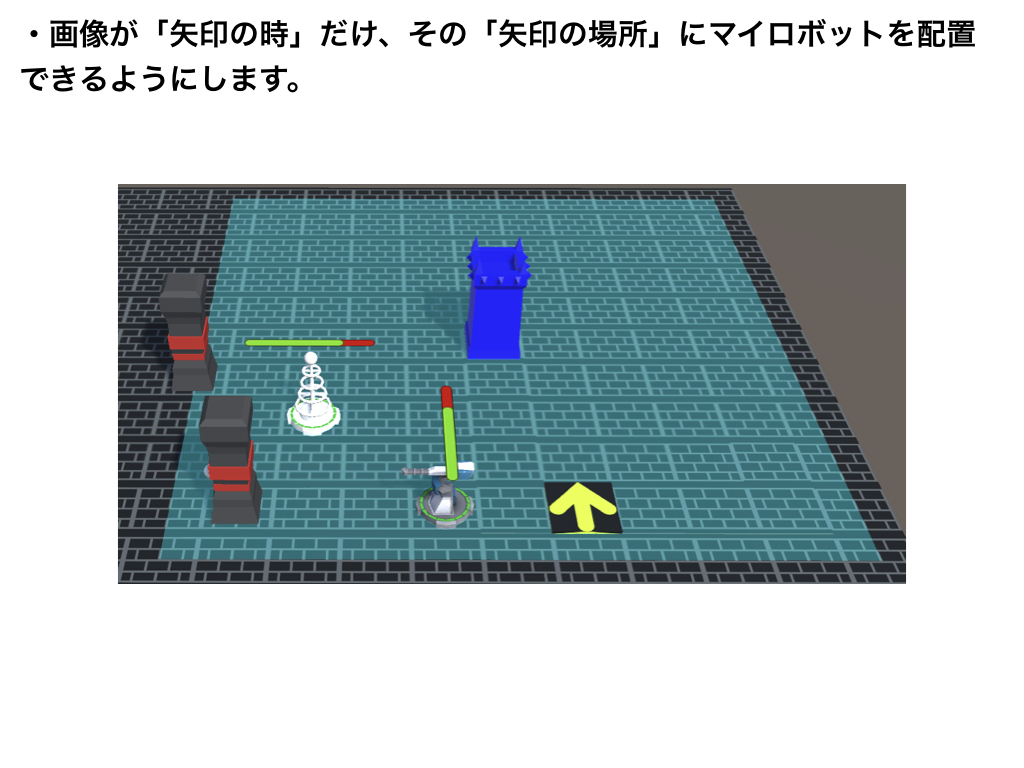
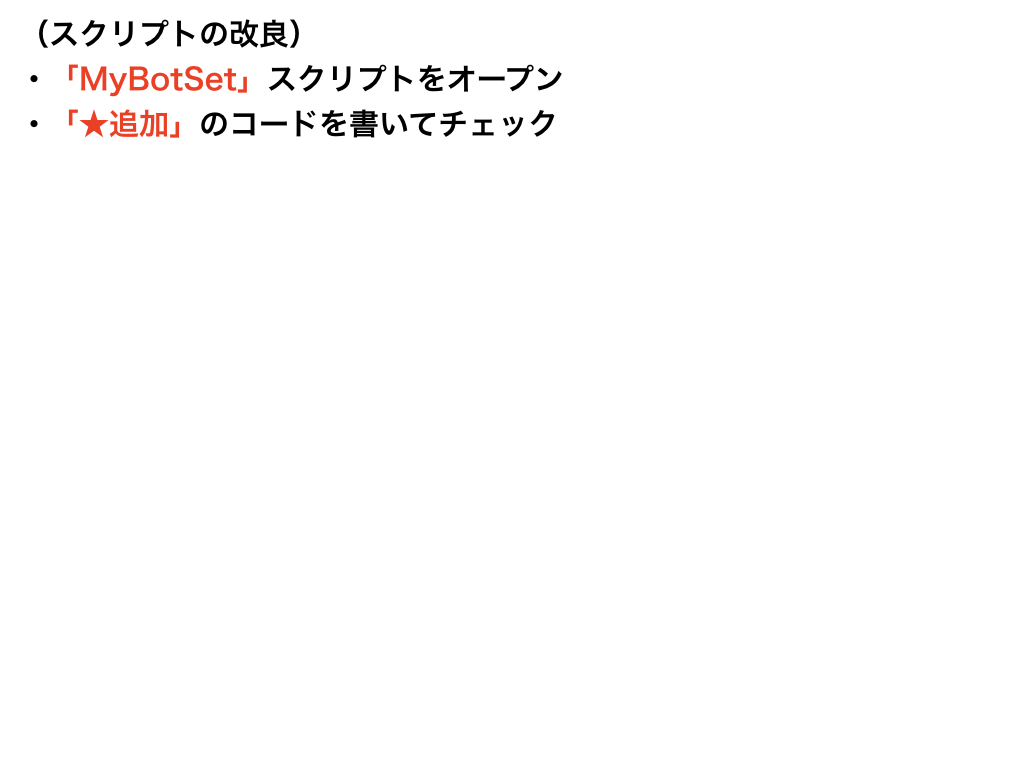
矢印の時だけ配置可能
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class MyBotSet : MonoBehaviour
{
private int setCount;
private Text countLabel;
private int getGPpoint;
private GPManager gpm;
public int price;
public GameObject myBotPrefab;
private GameObject myBotBase;
// ★追加
private bool setOK;
void Start()
{
setCount = 0;
countLabel = GetComponent<Text>();
countLabel.text = "× " + setCount.ToString("D3");
gpm = GameObject.Find("GPLabel").GetComponent<GPManager>();
myBotBase = GameObject.Find("MyBotBase");
}
void Update()
{
getGPpoint = gpm.gpPoint;
setCount = getGPpoint / price;
countLabel.text = "×" + setCount.ToString("D3");
// ★追加
setOK = myBotBase.GetComponent<MyBotBase>().isSetOK;
}
public void OnMyBotButtonClicked()
{
// ★追加(『&& setOK == true』を追加する)
if (setCount > 0 && setOK == true)
{
Instantiate(myBotPrefab, myBotBase.transform.position, myBotBase.transform.rotation);
gpm.ReduceGP(price);
}
}
}
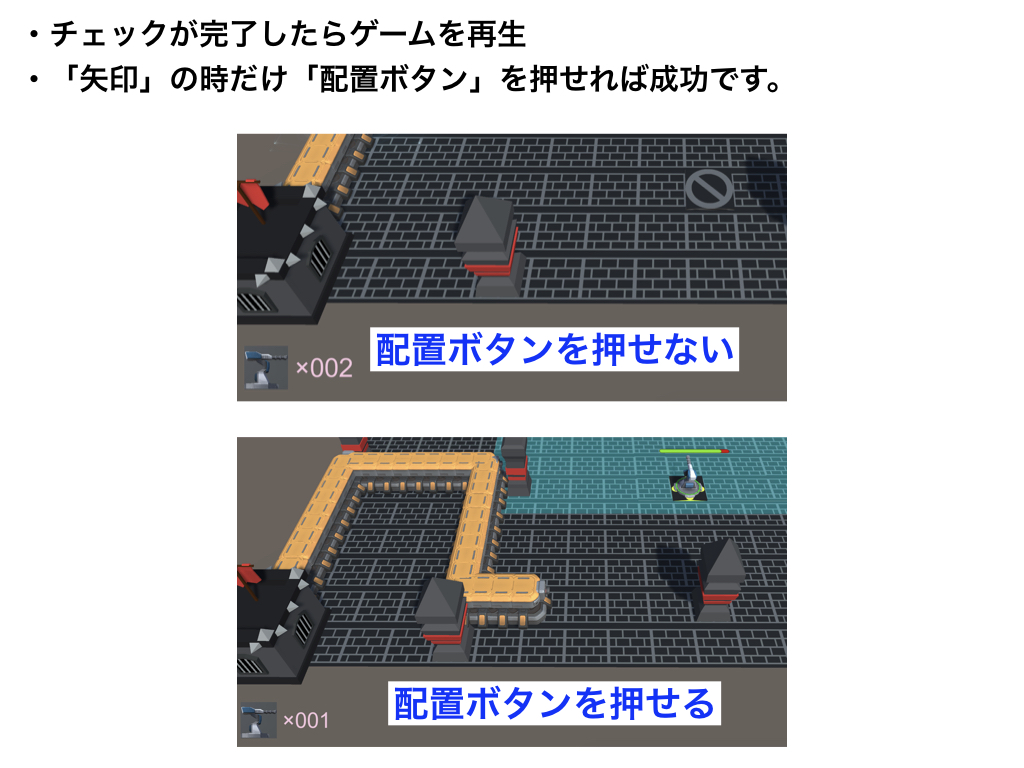
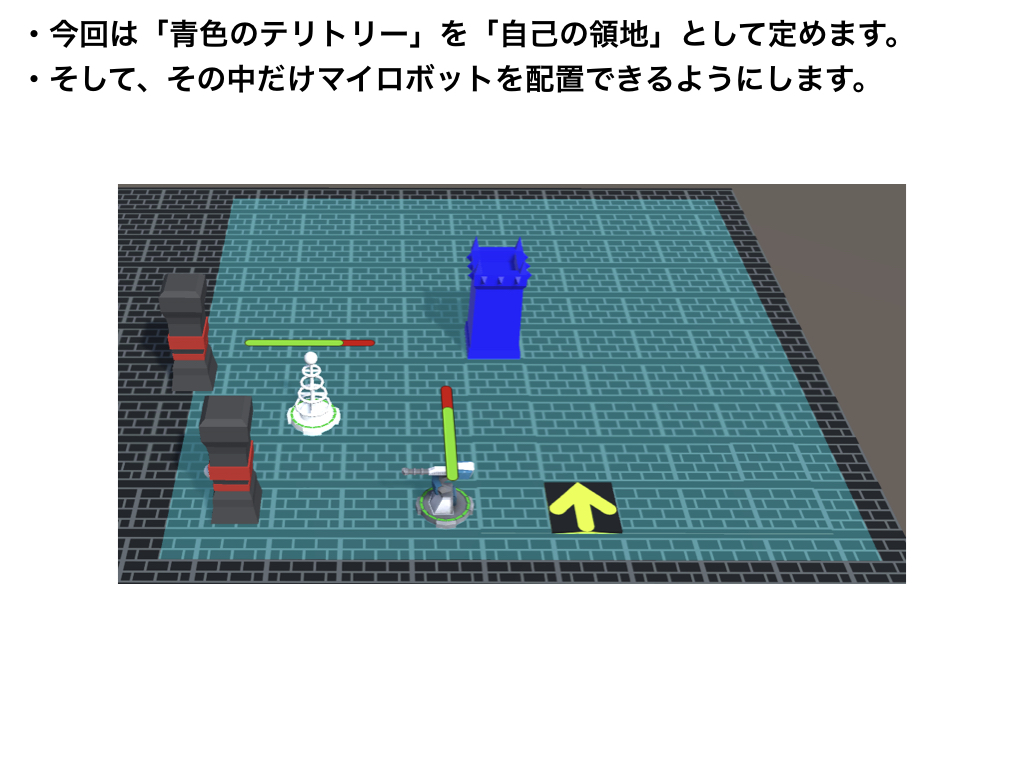
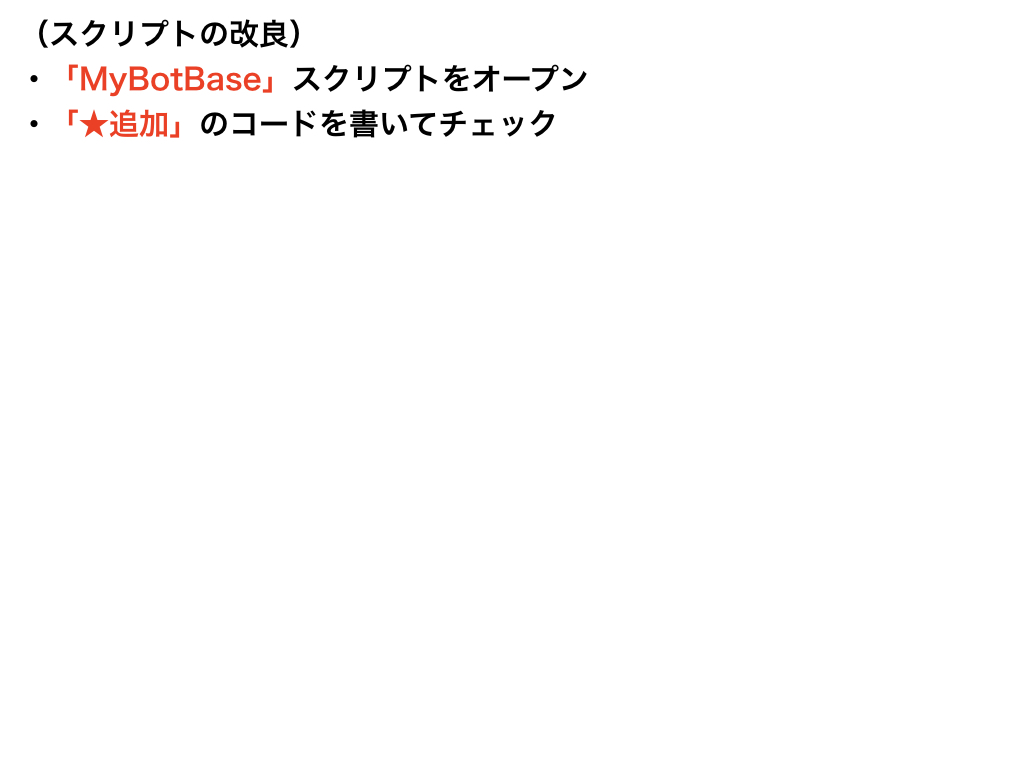
テリトリー内だけ矢印になる
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class MyBotBase : MonoBehaviour
{
private int num = 0;
// ★追加
public Material[] myMaterials;
public bool isSetOK;
// ★追加
private void Start()
{
GetComponent<MeshRenderer>().material = myMaterials[0];
isSetOK = false;
}
void Update()
{
Vector3 pos = transform.position;
if(Input.GetKeyDown(KeyCode.LeftArrow))
{
transform.position = new Vector3(pos.x - 1, 0, pos.z);
}
if (Input.GetKeyDown(KeyCode.RightArrow))
{
transform.position = new Vector3(pos.x + 1, 0, pos.z);
}
if (Input.GetKeyDown(KeyCode.UpArrow))
{
transform.position = new Vector3(pos.x, 0, pos.z + 1);
}
if (Input.GetKeyDown(KeyCode.DownArrow))
{
transform.position = new Vector3(pos.x, 0, pos.z - 1);
}
if (Input.GetKeyDown(KeyCode.RightShift))
{
num += 1;
transform.rotation = Quaternion.Euler(0, 90 * num, 0);
}
}
// ★追加
// (ポイント)
// Trigger系のメソッドを使うので、Is Triggerにチェックが必要!!
// 表示が切り替わらない場合には『MyBotBase』オブジェクトの『Box Collider』の『Is Trigger』にチェックが入っているか確認しよう!
private void OnTriggerStay(Collider other)
{
if(other.gameObject.tag == "BlueTeritory")
{
GetComponent<MeshRenderer>().material = myMaterials[1];
isSetOK = true;
}
else if(other.gameObject.tag == "RedTeritory")
{
GetComponent<MeshRenderer>().material = myMaterials[0];
isSetOK = false;
}
}
// ★追加
private void OnTriggerExit(Collider other)
{
if (other.gameObject.tag == "BlueTeritory")
{
GetComponent<MeshRenderer>().material = myMaterials[0];
isSetOK = false;
}
}
}
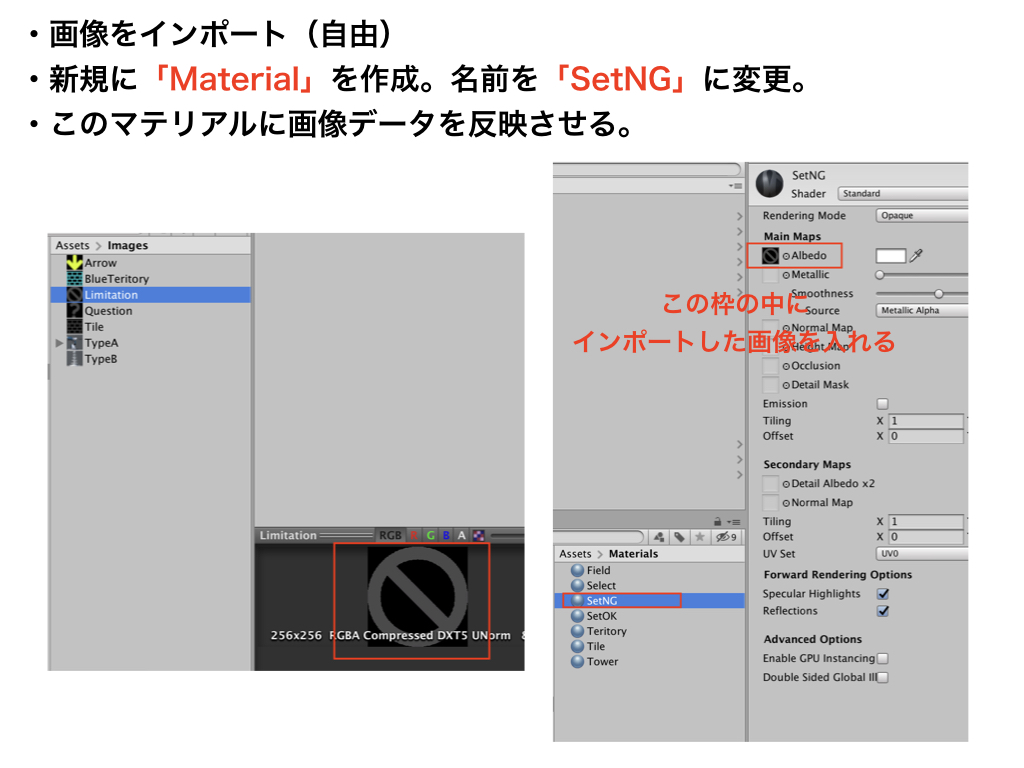
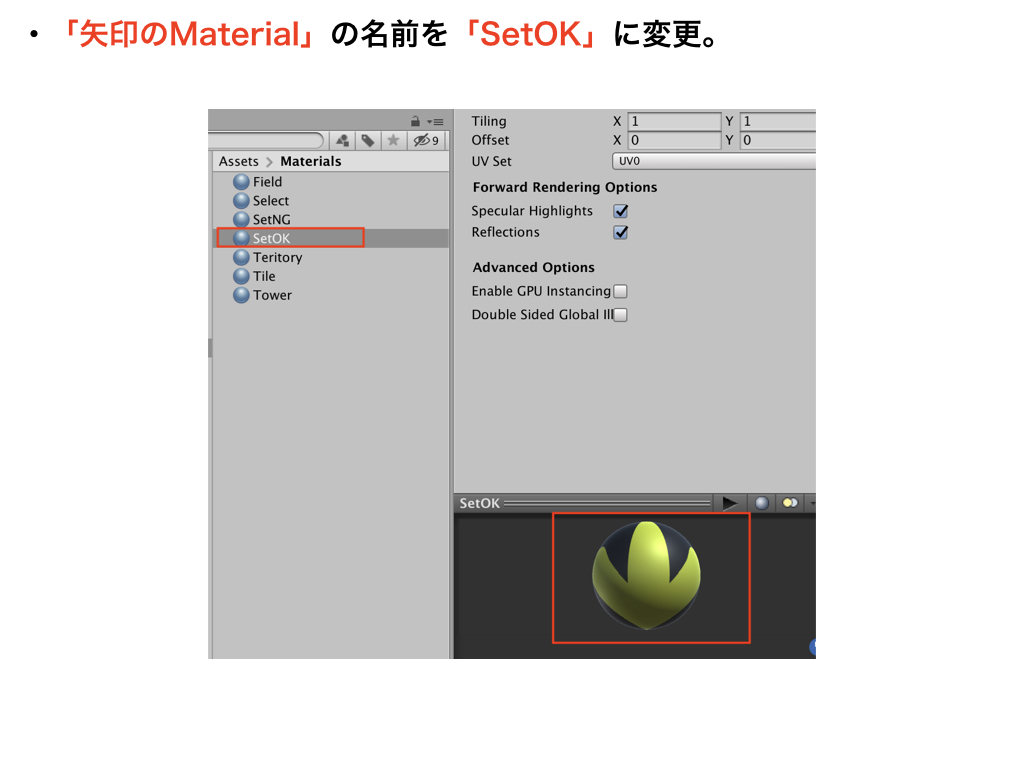
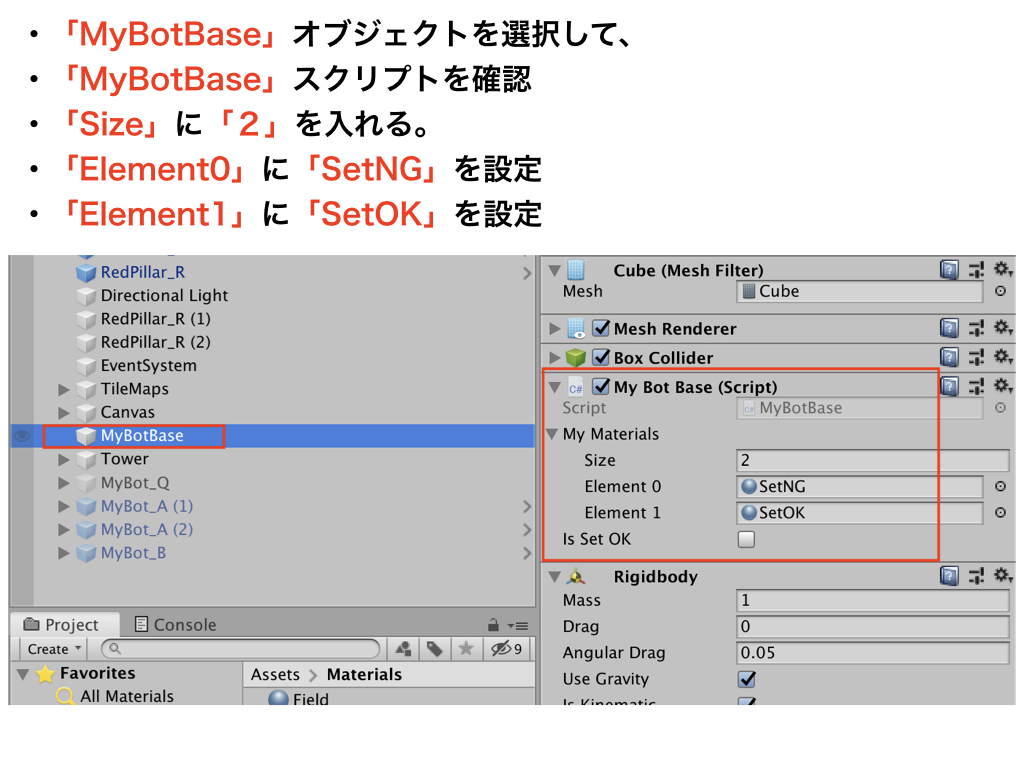
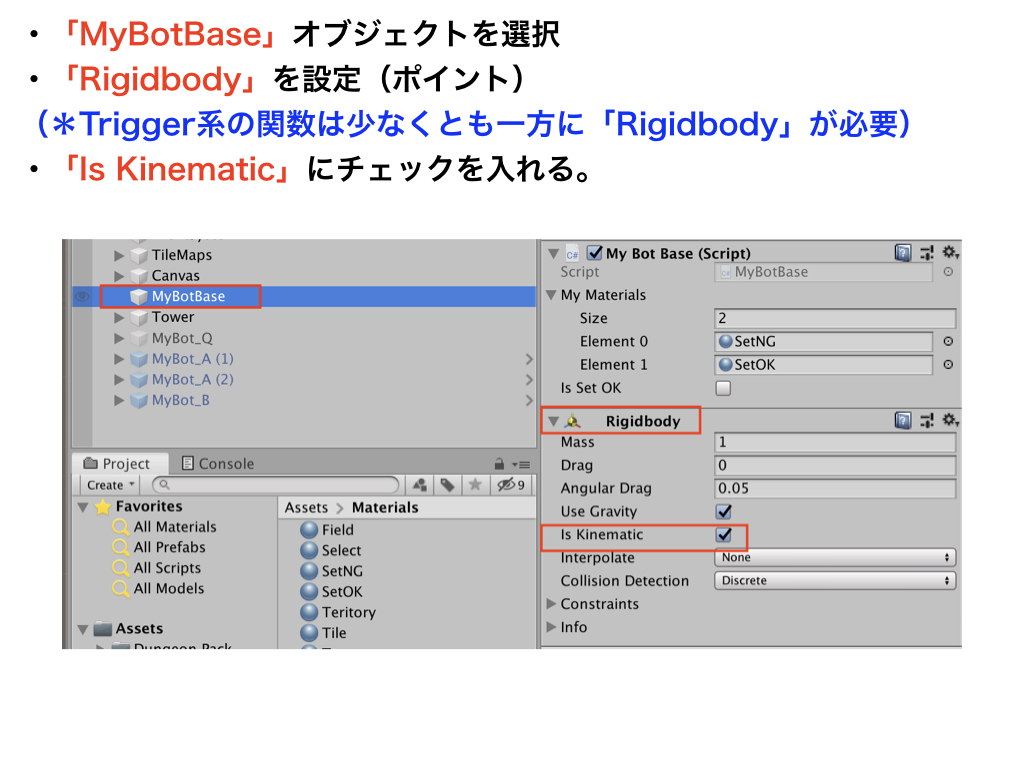
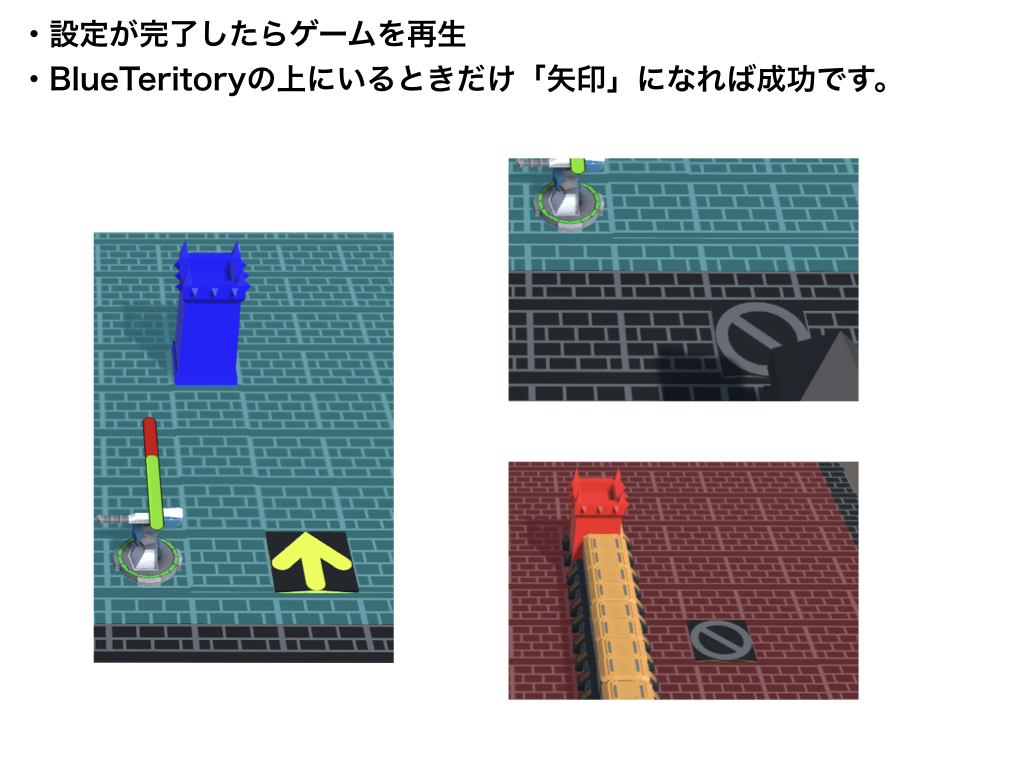
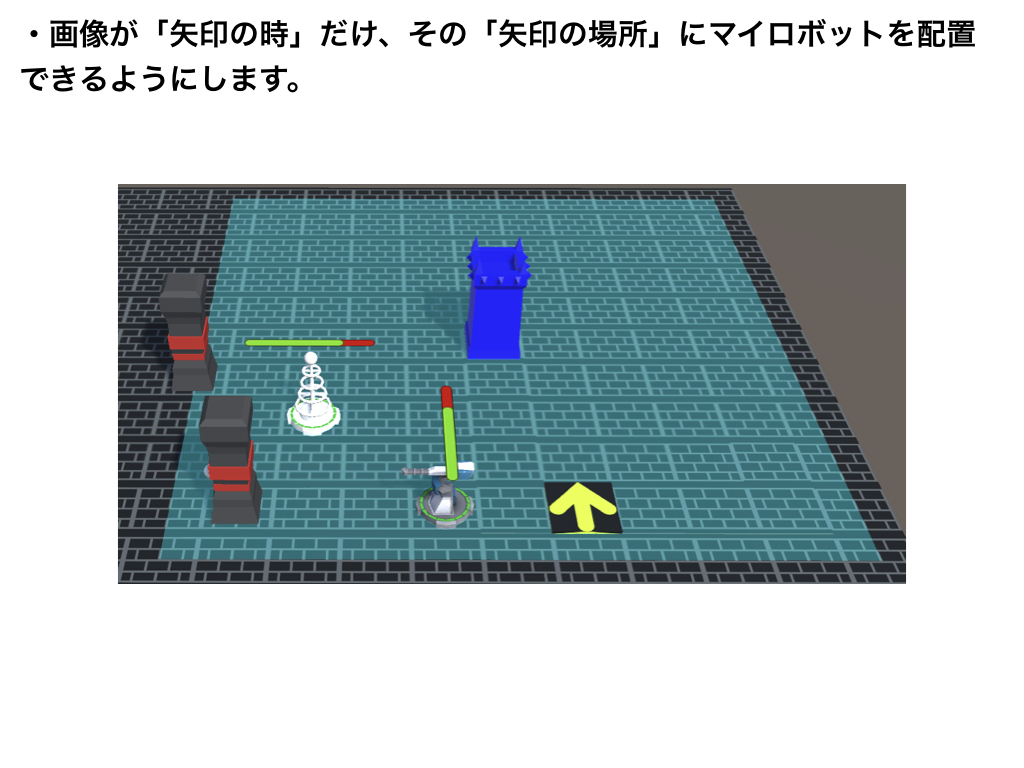
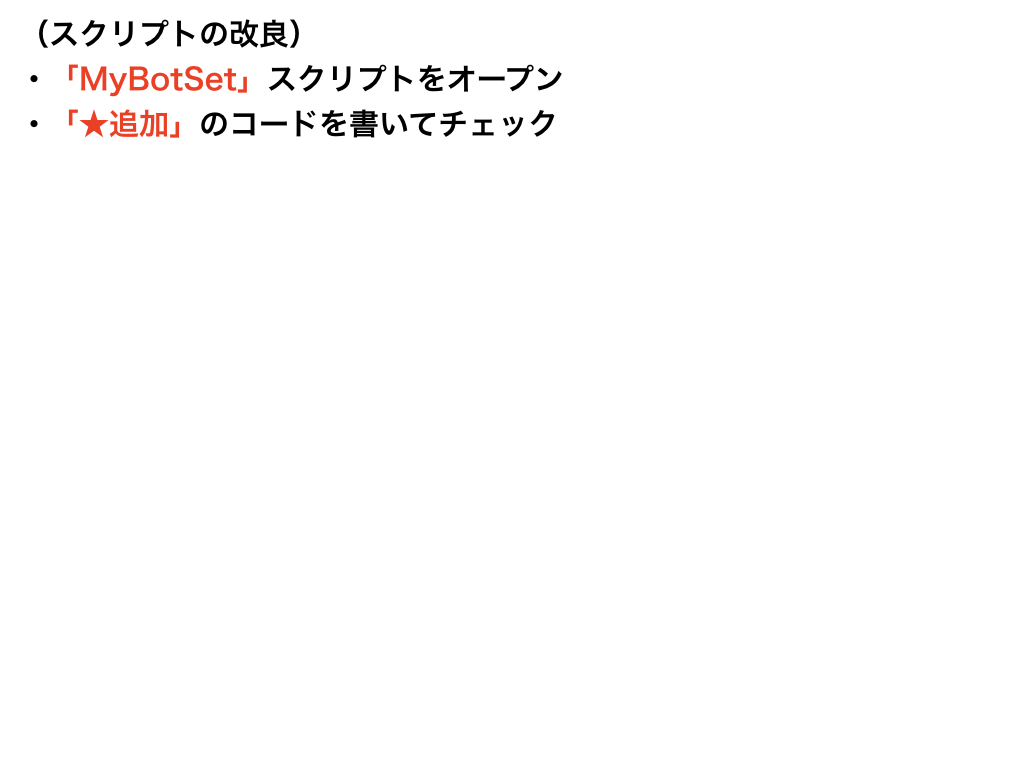
矢印の時だけ配置可能
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class MyBotSet : MonoBehaviour
{
private int setCount;
private Text countLabel;
private int getGPpoint;
private GPManager gpm;
public int price;
public GameObject myBotPrefab;
private GameObject myBotBase;
// ★追加
private bool setOK;
void Start()
{
setCount = 0;
countLabel = GetComponent<Text>();
countLabel.text = "× " + setCount.ToString("D3");
gpm = GameObject.Find("GPLabel").GetComponent<GPManager>();
myBotBase = GameObject.Find("MyBotBase");
}
void Update()
{
getGPpoint = gpm.gpPoint;
setCount = getGPpoint / price;
countLabel.text = "×" + setCount.ToString("D3");
// ★追加
setOK = myBotBase.GetComponent<MyBotBase>().isSetOK;
}
public void OnMyBotButtonClicked()
{
// ★追加(『&& setOK == true』を追加する)
if (setCount > 0 && setOK == true)
{
Instantiate(myBotPrefab, myBotBase.transform.position, myBotBase.transform.rotation);
gpm.ReduceGP(price);
}
}
}
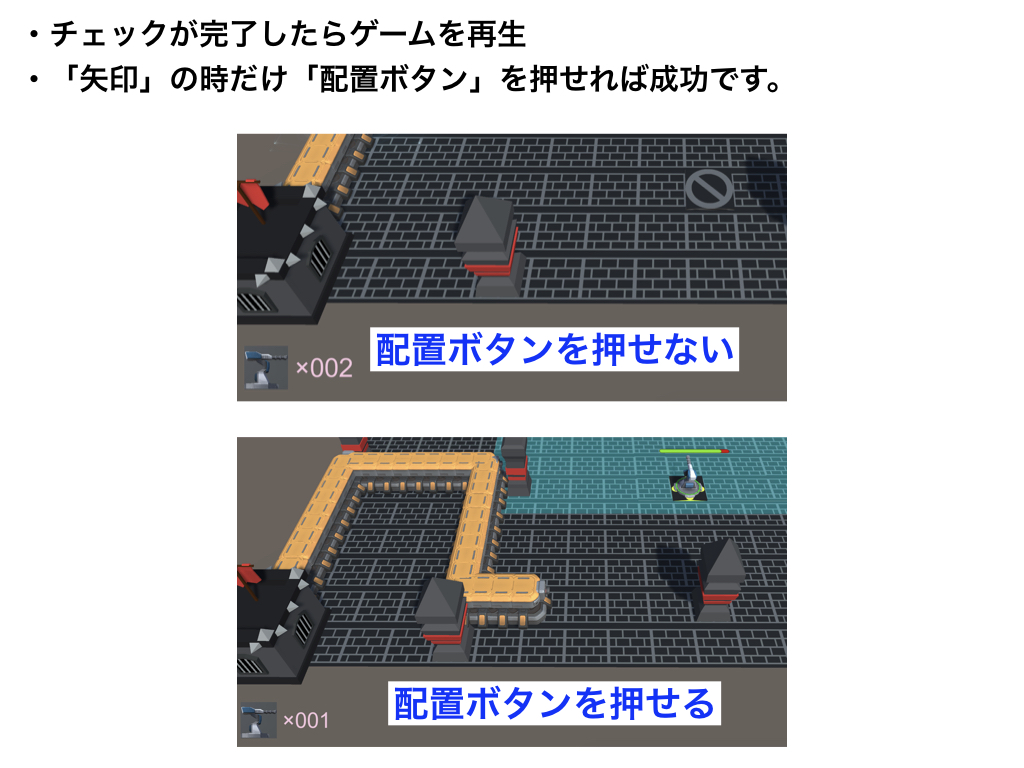
マイロボット配置システム6