マイロボット配置システム3
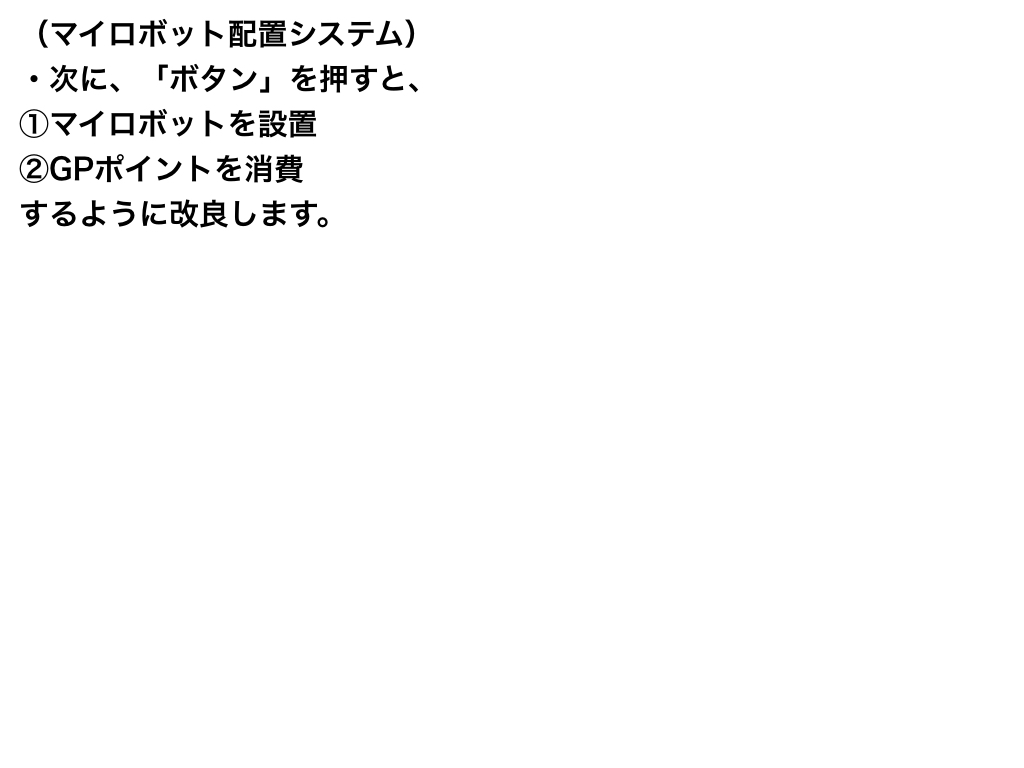
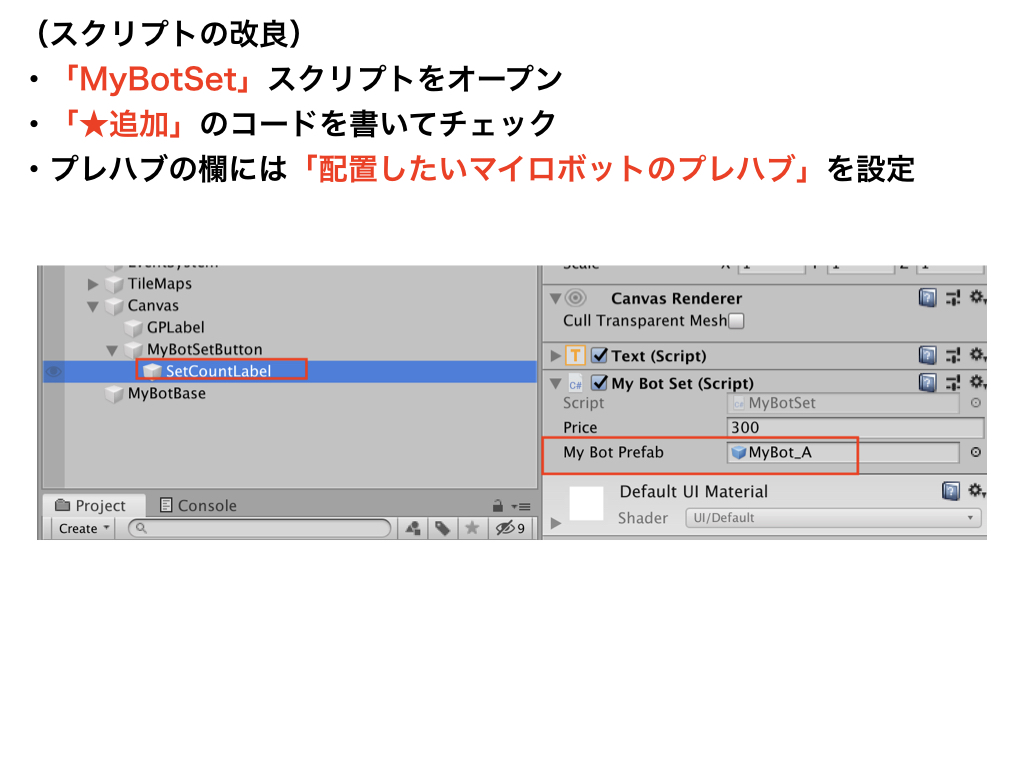
オブジェクトの配置
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class MyBotSet : MonoBehaviour
{
private int setCount;
private Text countLabel;
private int getGPpoint;
private GPManager gpm;
public int price;
// ★追加
public GameObject myBotPrefab;
private GameObject myBotBase;
void Start()
{
setCount = 0;
countLabel = GetComponent<Text>();
countLabel.text = "× " + setCount.ToString("D3");
gpm = GameObject.Find("GPLabel").GetComponent<GPManager>();
// ★追加
myBotBase = GameObject.Find("MyBotBase");
}
void Update()
{
getGPpoint = gpm.gpPoint;
// 配置できるマイロボットの数・・・>「獲得したGPポイント ÷ マイロボットの価格」
setCount = getGPpoint / price;
countLabel.text = "×" + setCount.ToString("D3");
}
// ★追加
public void OnMyBotButtonClicked()
{
// (復習)この条件の意味は?
if (setCount > 0)
{
Instantiate(myBotPrefab, myBotBase.transform.position, myBotBase.transform.rotation);
}
}
}
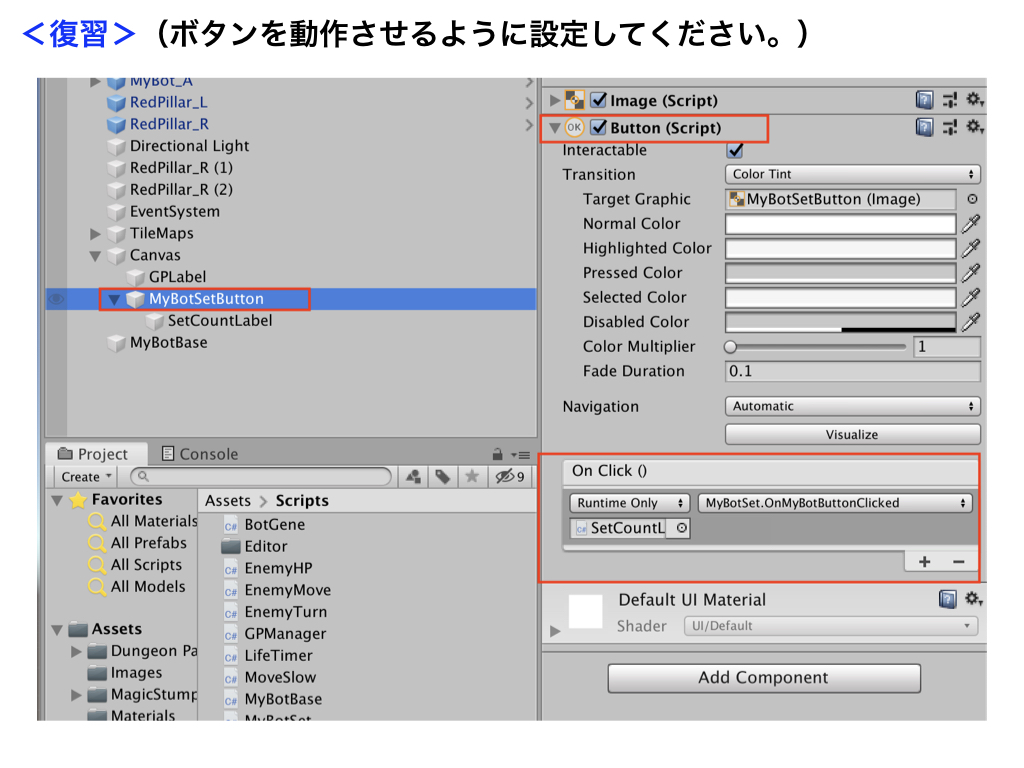
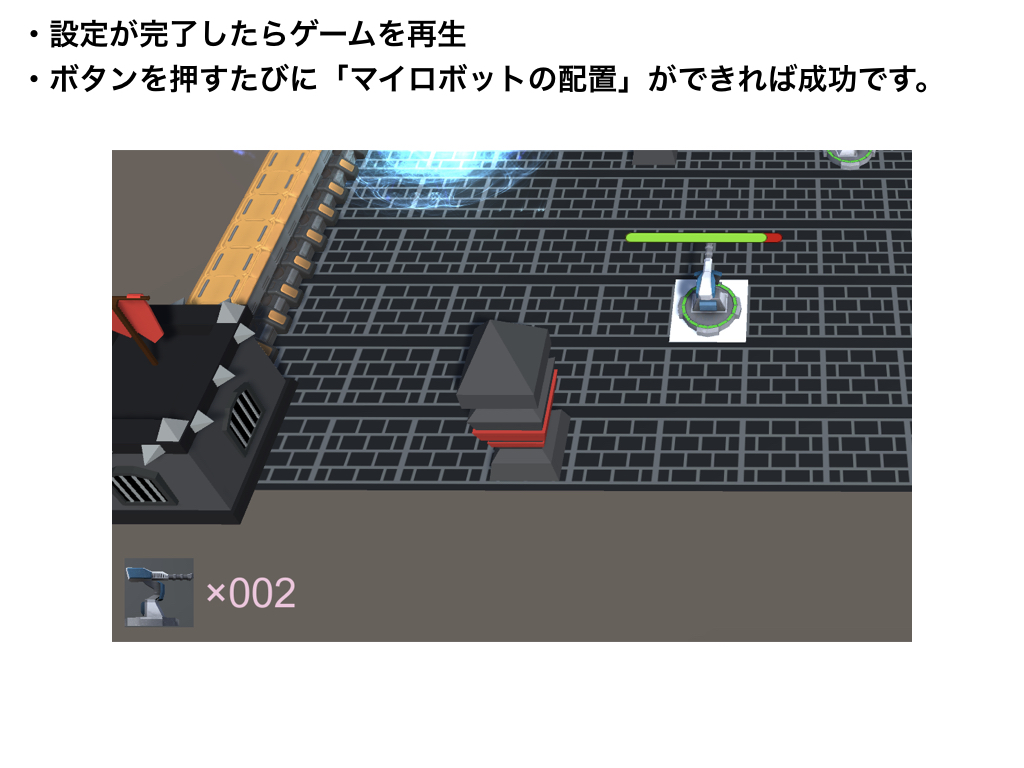
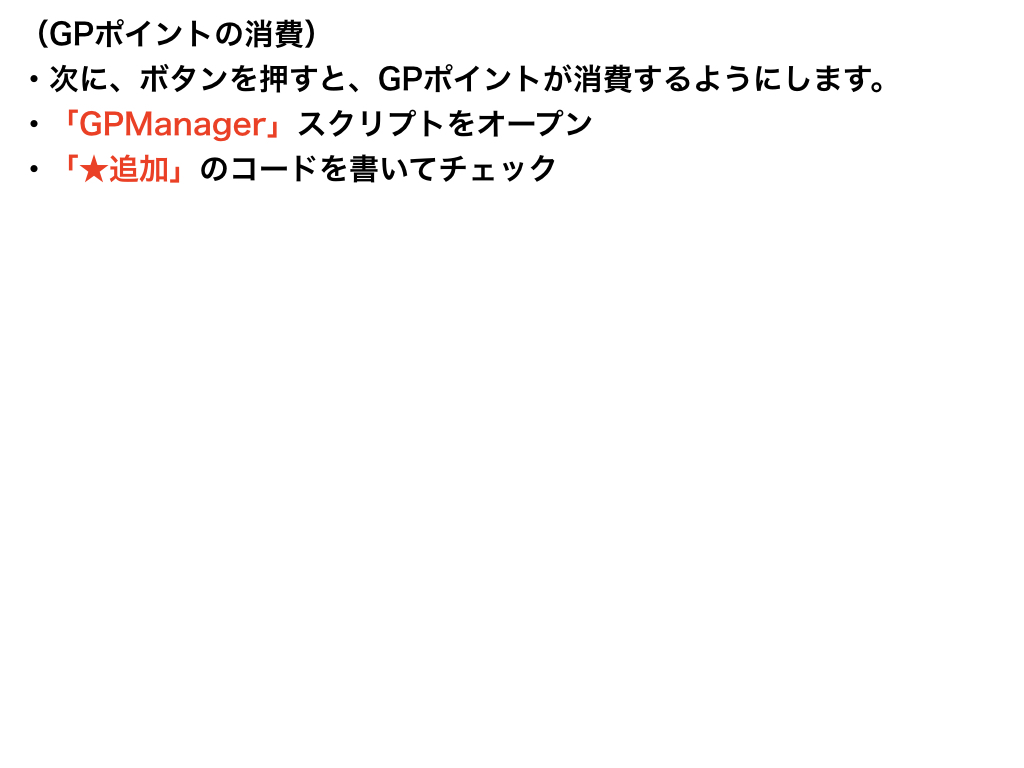
GPポイントの消費
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class GPManager : MonoBehaviour
{
private Text gpLabel;
public int gpPoint;
void Start()
{
gpPoint = 0;
gpLabel = GetComponent<Text>();
gpLabel.text = "GP:" + gpPoint.ToString("D10");
}
public void AddGP(int amount)
{
gpPoint += amount;
gpLabel.text = "GP:" + gpPoint.ToString("D10");
}
// ★追加
public void ReduceGP(int amount)
{
gpPoint -= amount;
gpLabel.text = "GP:" + gpPoint.ToString("D10");
}
}
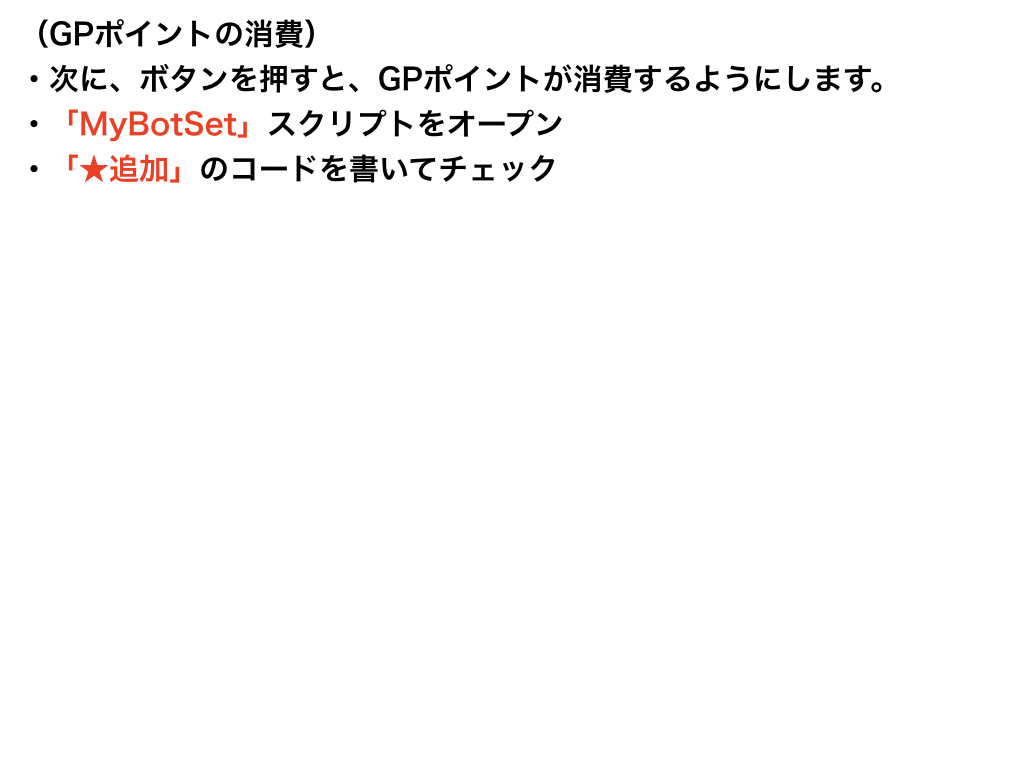
GPポイントの消費
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class MyBotSet : MonoBehaviour
{
private int setCount;
private Text countLabel;
private int getGPpoint;
private GPManager gpm;
public int price;
public GameObject myBotPrefab;
private GameObject myBotBase;
void Start()
{
setCount = 0;
countLabel = GetComponent<Text>();
countLabel.text = "× " + setCount.ToString("D3");
gpm = GameObject.Find("GPLabel").GetComponent<GPManager>();
myBotBase = GameObject.Find("MyBotBase");
}
void Update()
{
getGPpoint = gpm.gpPoint;
setCount = getGPpoint / price;
countLabel.text = "×" + setCount.ToString("D3");
}
public void OnMyBotButtonClicked()
{
if (setCount > 0)
{
Instantiate(myBotPrefab, myBotBase.transform.position, myBotBase.transform.rotation);
// ★追加
gpm.ReduceGP(price);
}
}
}
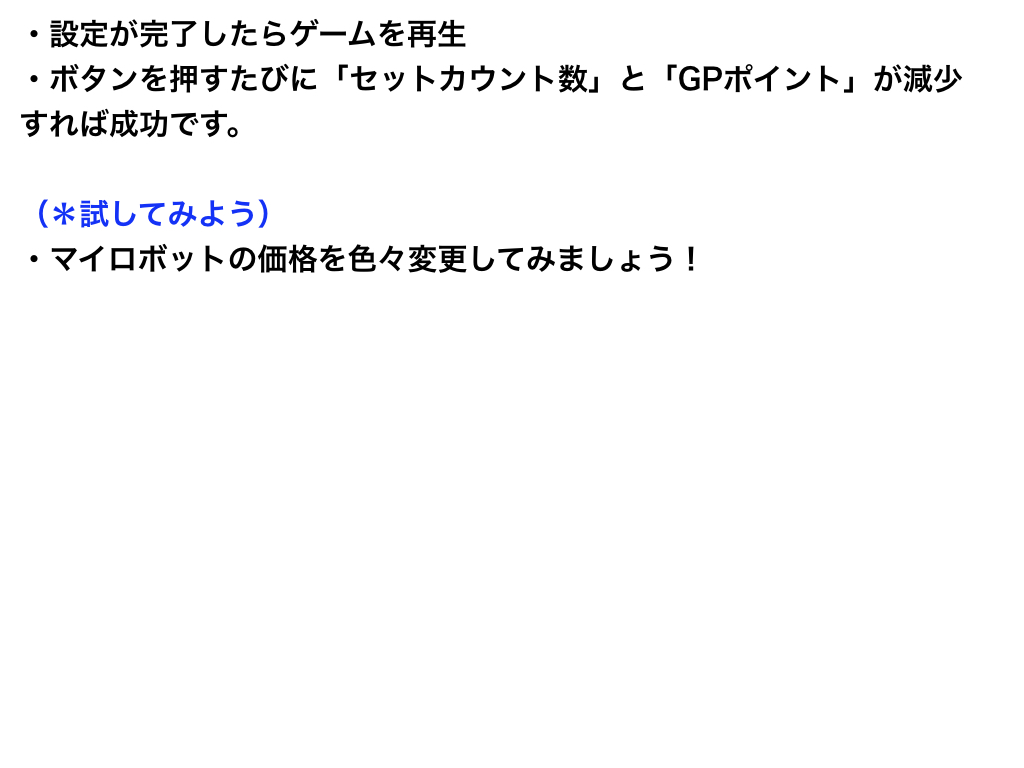
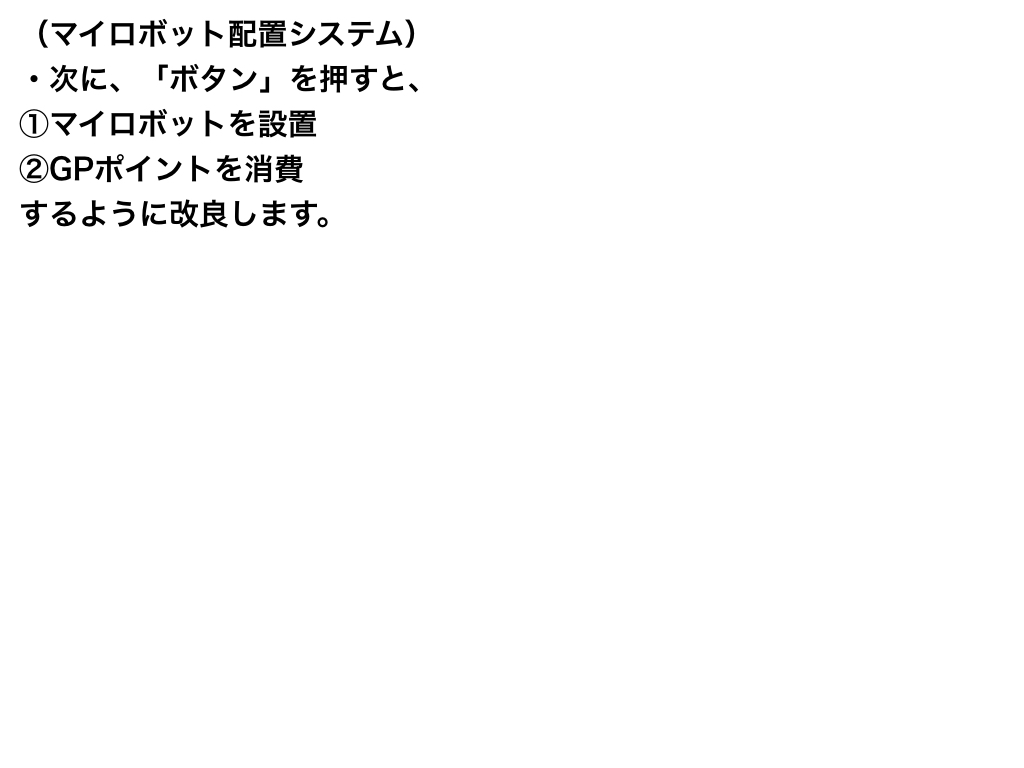
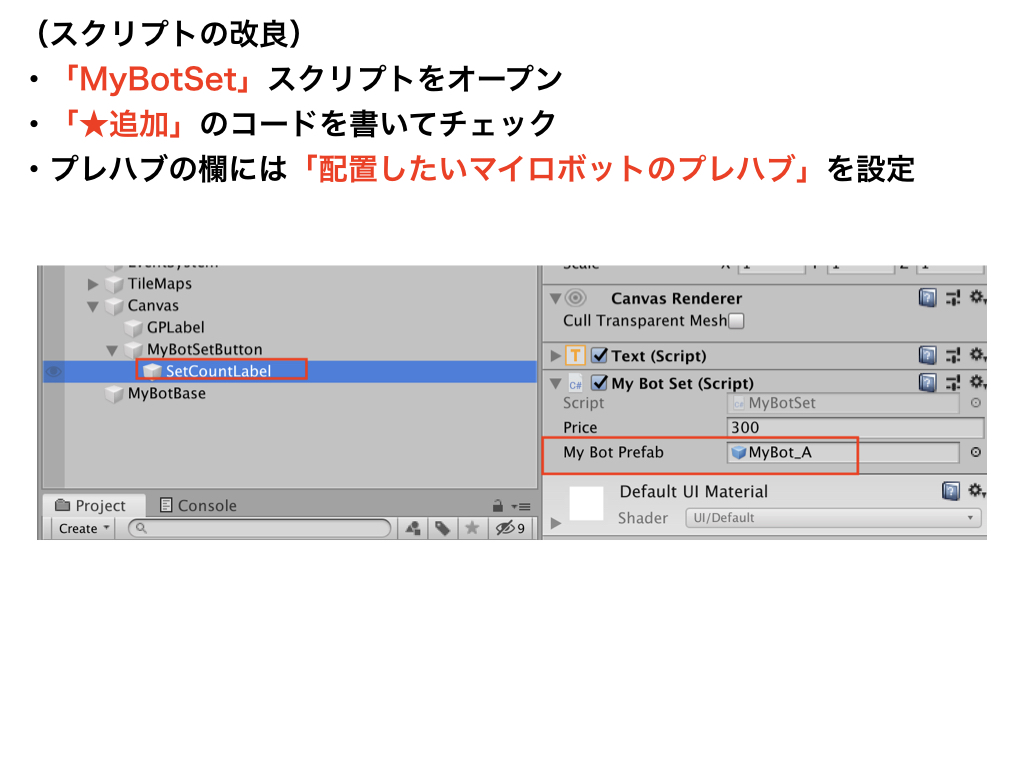
オブジェクトの配置
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class MyBotSet : MonoBehaviour
{
private int setCount;
private Text countLabel;
private int getGPpoint;
private GPManager gpm;
public int price;
// ★追加
public GameObject myBotPrefab;
private GameObject myBotBase;
void Start()
{
setCount = 0;
countLabel = GetComponent<Text>();
countLabel.text = "× " + setCount.ToString("D3");
gpm = GameObject.Find("GPLabel").GetComponent<GPManager>();
// ★追加
myBotBase = GameObject.Find("MyBotBase");
}
void Update()
{
getGPpoint = gpm.gpPoint;
// 配置できるマイロボットの数・・・>「獲得したGPポイント ÷ マイロボットの価格」
setCount = getGPpoint / price;
countLabel.text = "×" + setCount.ToString("D3");
}
// ★追加
public void OnMyBotButtonClicked()
{
// (復習)この条件の意味は?
if (setCount > 0)
{
Instantiate(myBotPrefab, myBotBase.transform.position, myBotBase.transform.rotation);
}
}
}
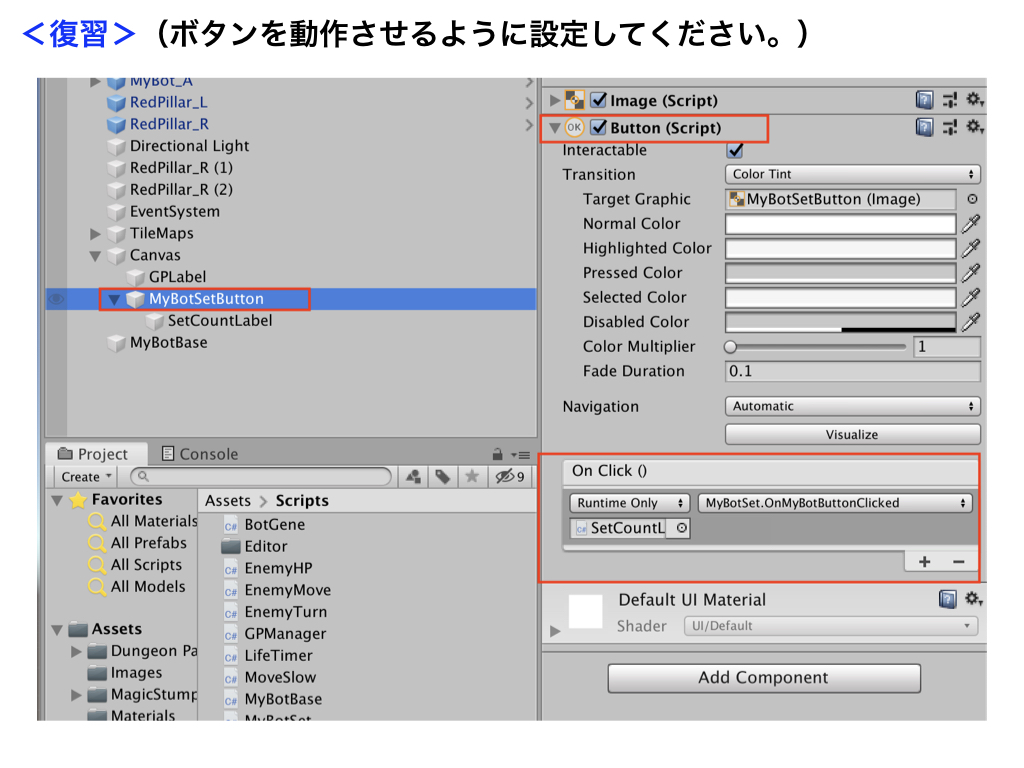
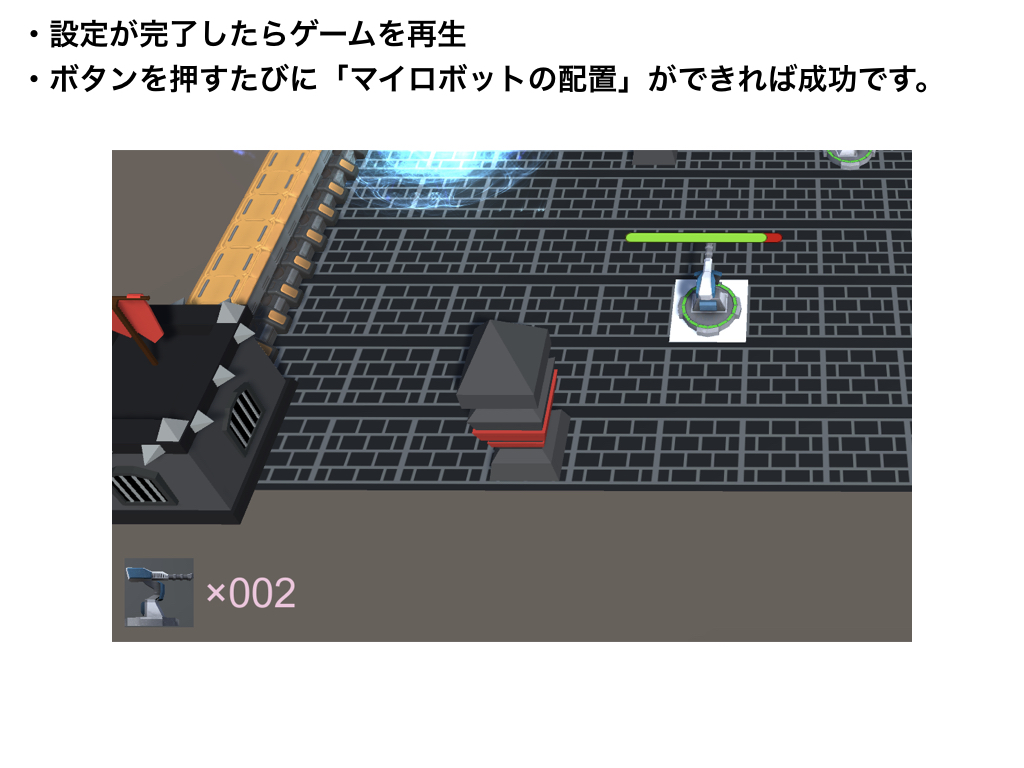
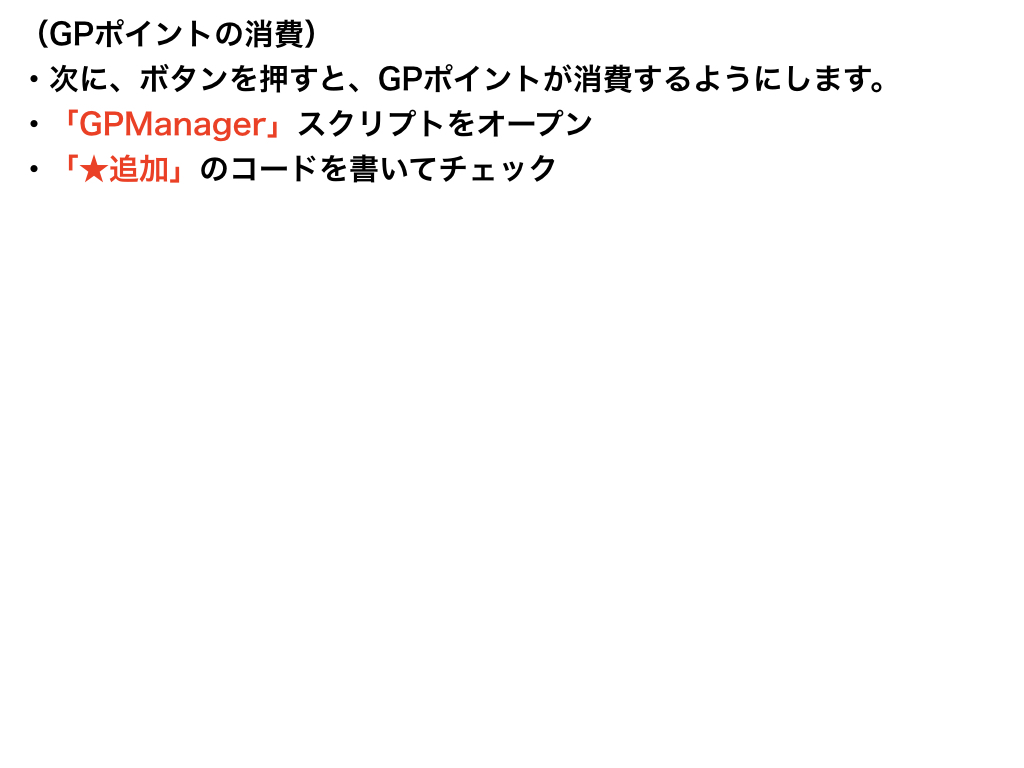
GPポイントの消費
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class GPManager : MonoBehaviour
{
private Text gpLabel;
public int gpPoint;
void Start()
{
gpPoint = 0;
gpLabel = GetComponent<Text>();
gpLabel.text = "GP:" + gpPoint.ToString("D10");
}
public void AddGP(int amount)
{
gpPoint += amount;
gpLabel.text = "GP:" + gpPoint.ToString("D10");
}
// ★追加
public void ReduceGP(int amount)
{
gpPoint -= amount;
gpLabel.text = "GP:" + gpPoint.ToString("D10");
}
}
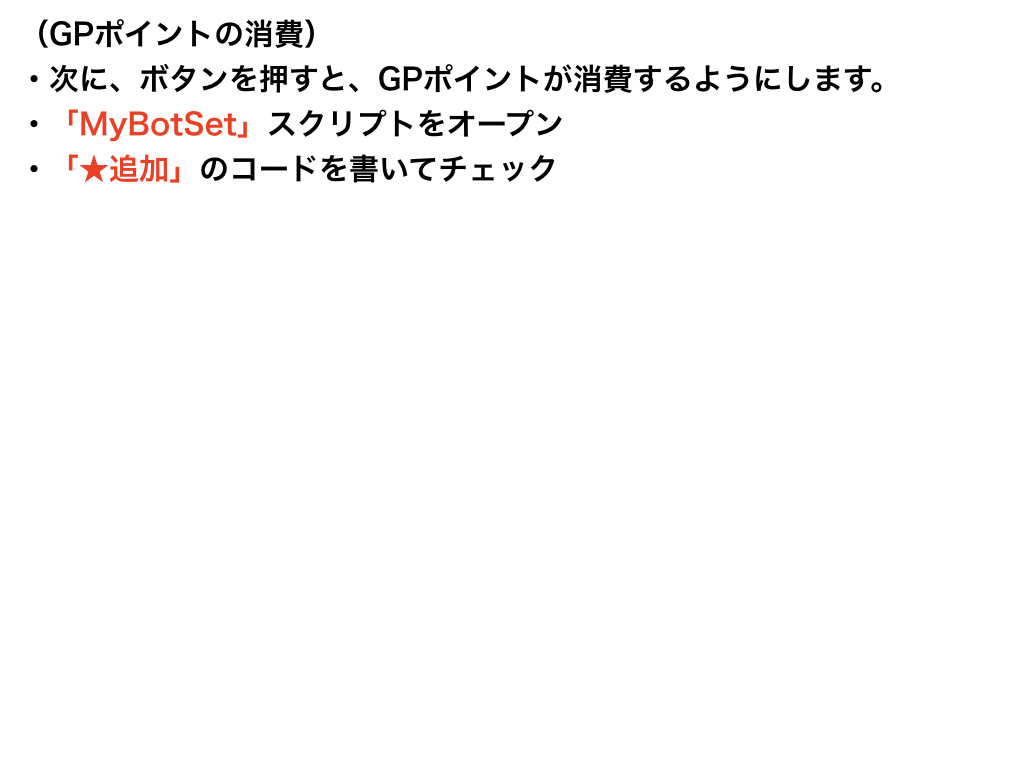
GPポイントの消費
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class MyBotSet : MonoBehaviour
{
private int setCount;
private Text countLabel;
private int getGPpoint;
private GPManager gpm;
public int price;
public GameObject myBotPrefab;
private GameObject myBotBase;
void Start()
{
setCount = 0;
countLabel = GetComponent<Text>();
countLabel.text = "× " + setCount.ToString("D3");
gpm = GameObject.Find("GPLabel").GetComponent<GPManager>();
myBotBase = GameObject.Find("MyBotBase");
}
void Update()
{
getGPpoint = gpm.gpPoint;
setCount = getGPpoint / price;
countLabel.text = "×" + setCount.ToString("D3");
}
public void OnMyBotButtonClicked()
{
if (setCount > 0)
{
Instantiate(myBotPrefab, myBotBase.transform.position, myBotBase.transform.rotation);
// ★追加
gpm.ReduceGP(price);
}
}
}
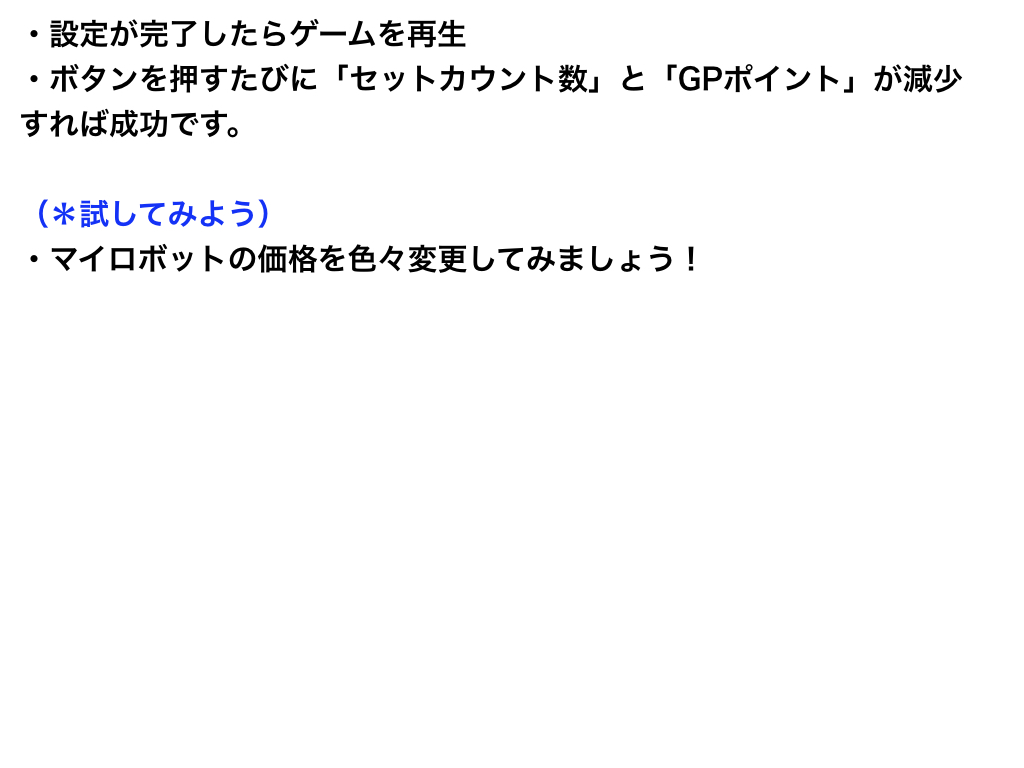
マイロボット配置システム3