攻撃力の作成
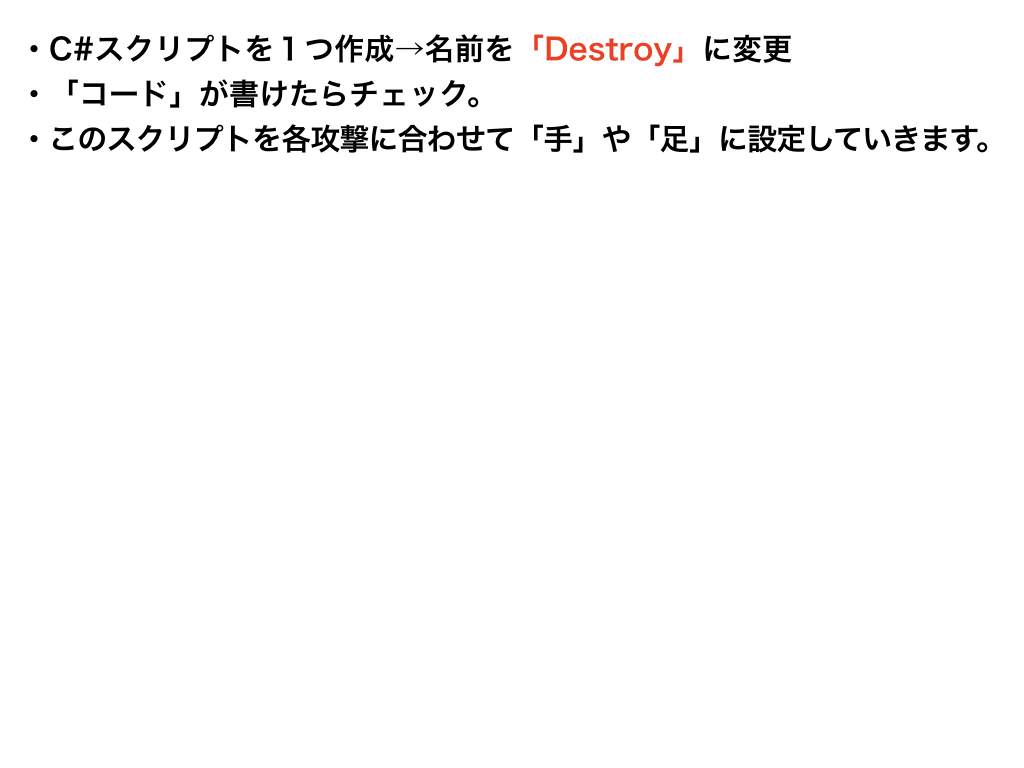
敵を破壊する
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Destroy : MonoBehaviour {
public AudioClip effectSound;
public GameObject effectPrefab;
void OnTriggerEnter(Collider other){
if (other.CompareTag ("Enemy")) {
Destroy (other.gameObject);
AudioSource.PlayClipAtPoint (effectSound, Camera.main.transform.position);
GameObject effect = (GameObject)Instantiate (effectPrefab, other.transform.position, Quaternion.identity);
Destroy (effect, 0.5f);
}
}
}
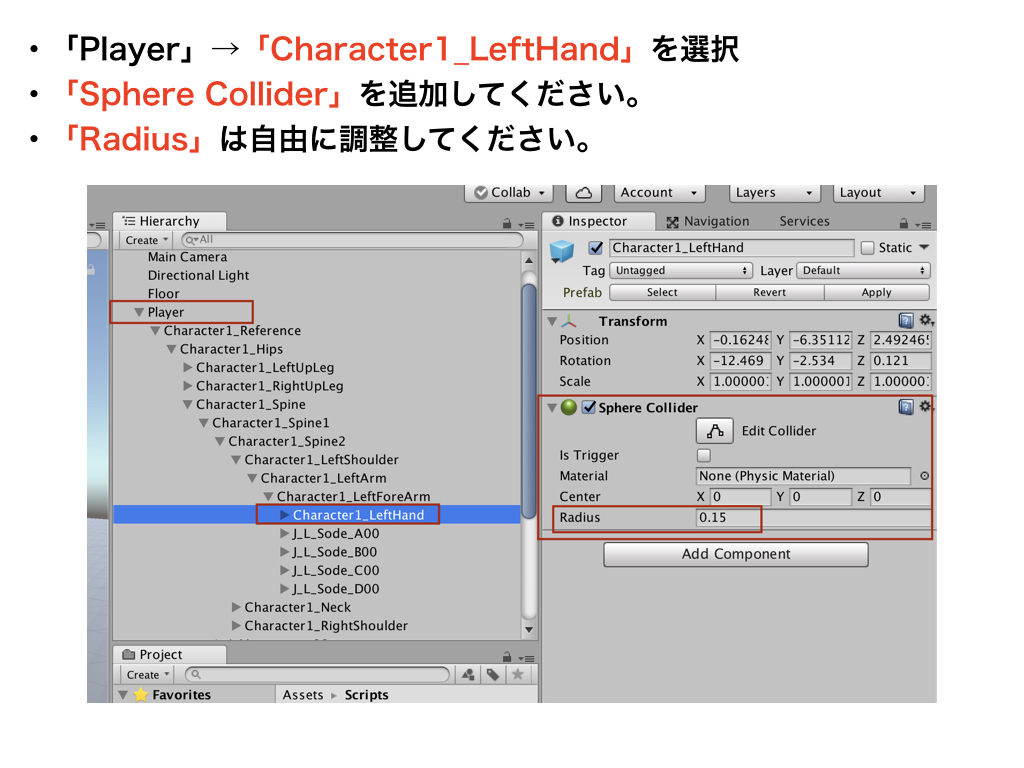
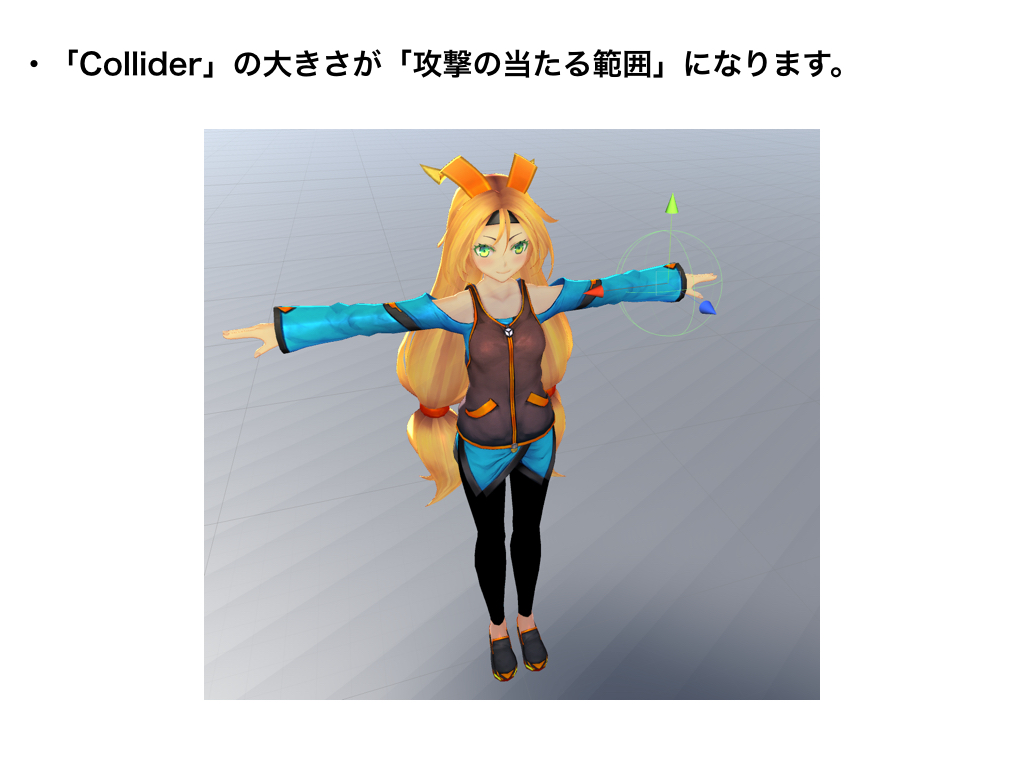
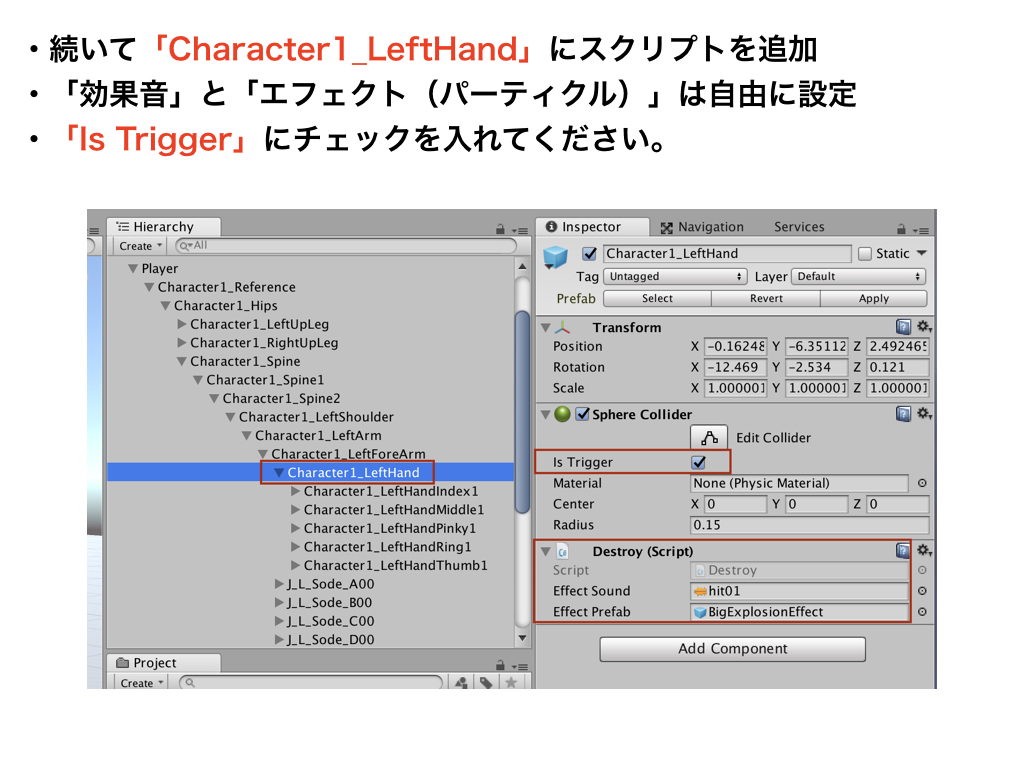
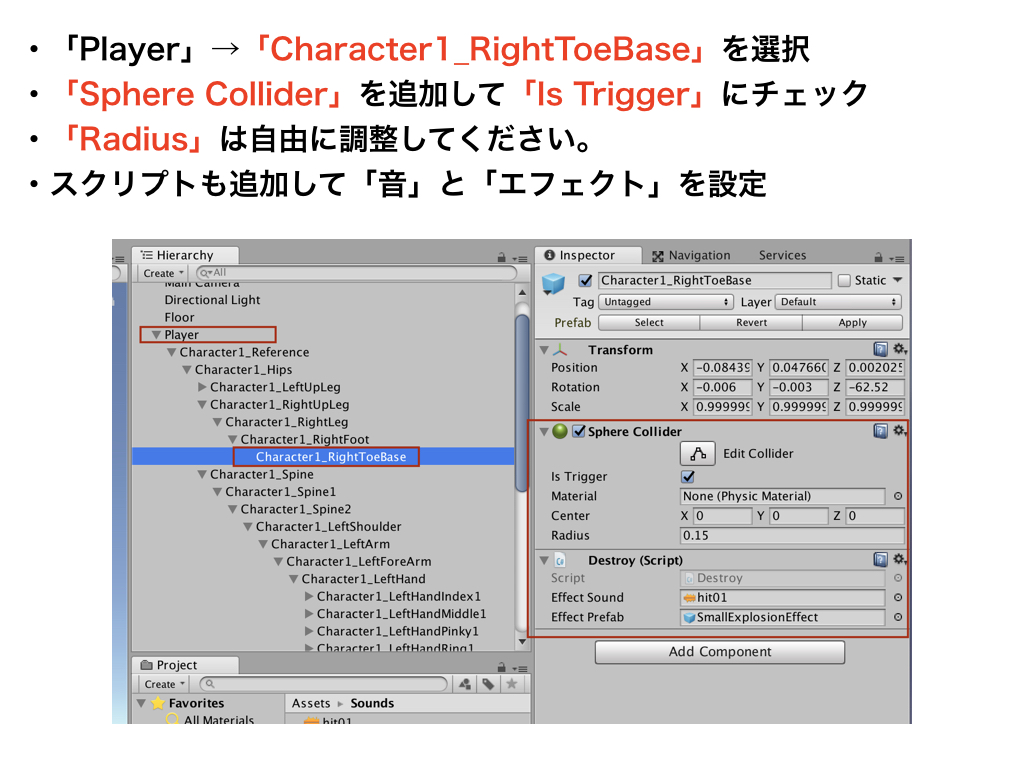
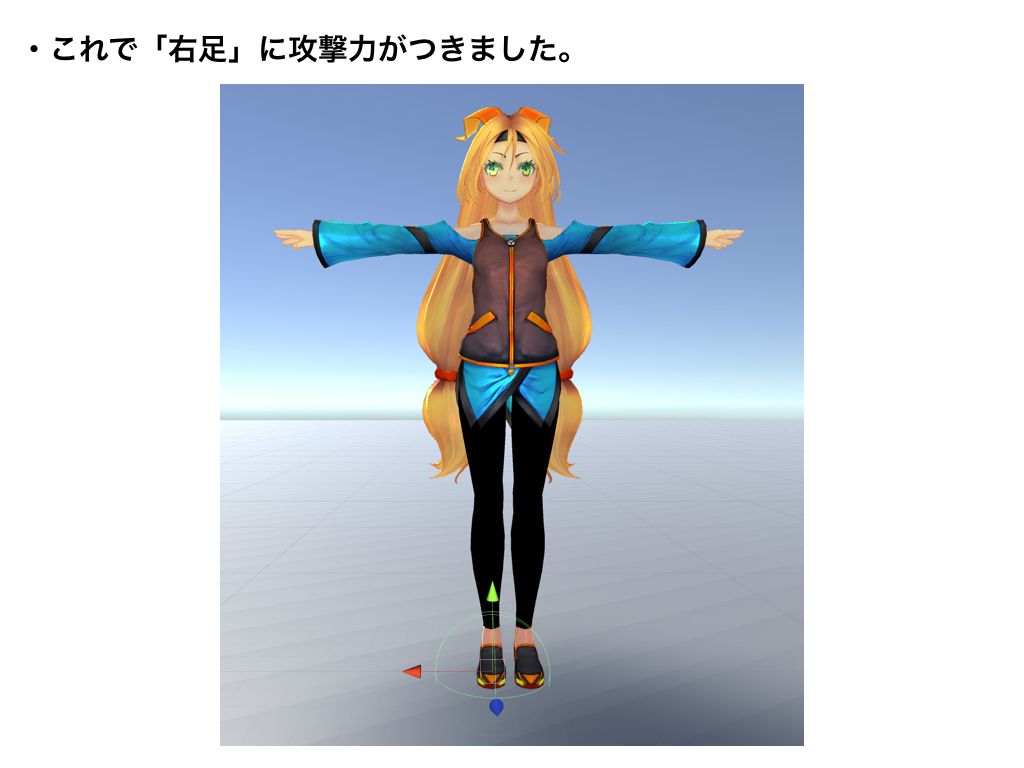
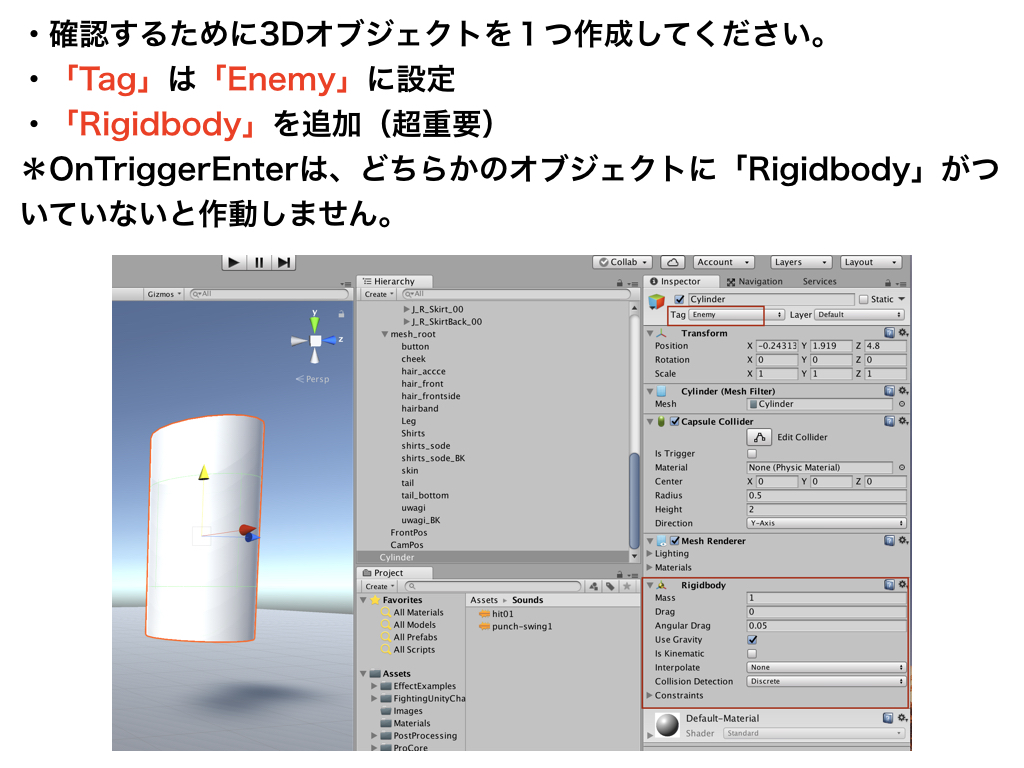
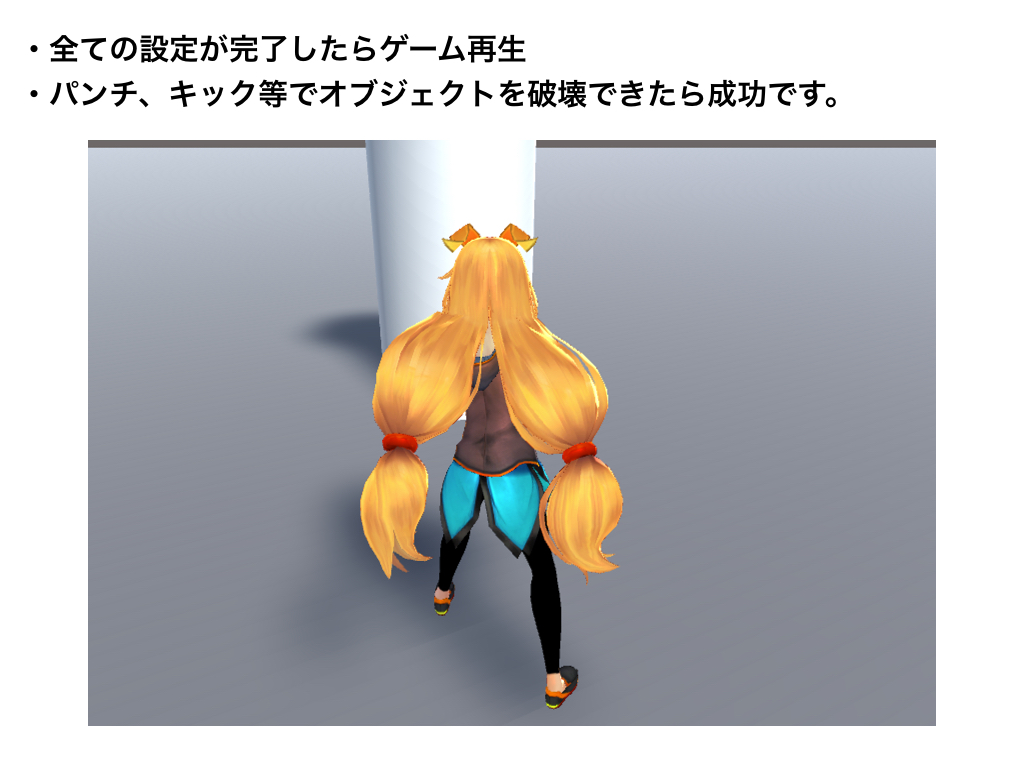
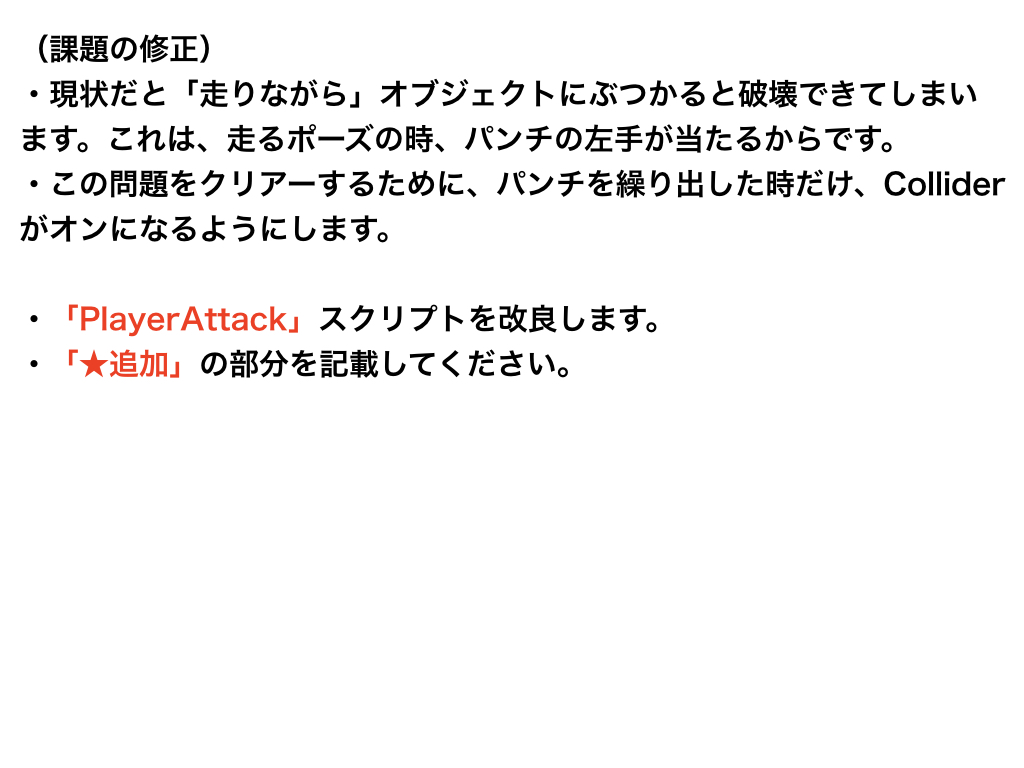
パンチを繰り出した時だけコライダーをオンにする
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerAttack : MonoBehaviour {
private Animator anim;
public AudioClip punchSound;
// ★追加
private Collider left_hand_col;
void Start () {
anim = GetComponent<Animator> ();
// ★追加
left_hand_col = GameObject.Find("Character1_LeftHand").GetComponent<SphereCollider>();
}
void Update () {
// Qボタンを押すとパンチ
if (Input.GetKeyDown (KeyCode.Q)) {
anim.SetBool ("Jab", true);
AudioSource.PlayClipAtPoint (punchSound, Camera.main.transform.position);
// ★追加
// 当たり判定をとるためにコライダーをオンの状態にする。
left_hand_col.enabled = true;
// ★追加
// 0.15秒後にコライダーをオフの状態に戻す。
Invoke("Reset", 0.15f);
}
// Eボタンを押すとハイキック
if (Input.GetKeyDown (KeyCode.E)) {
anim.SetBool ("Hikick", true);
}
// Sボタンを押すと回転キック
if (Input.GetKeyDown (KeyCode.S)) {
anim.SetBool ("Spinkick", true);
}
// Zボタンを押すとムーンソルトキック
if (Input.GetKeyDown (KeyCode.Z)) {
anim.SetBool ("SAMK", true);
}
}
// ★追加
void Reset(){
left_hand_col.enabled = false;
}
}
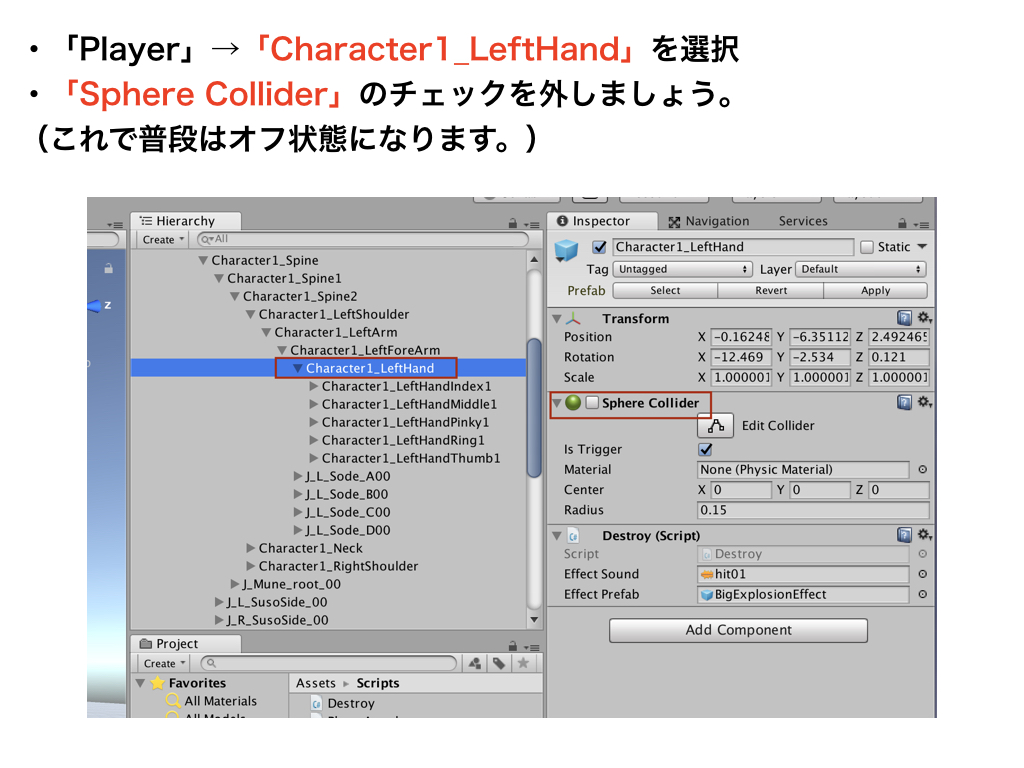
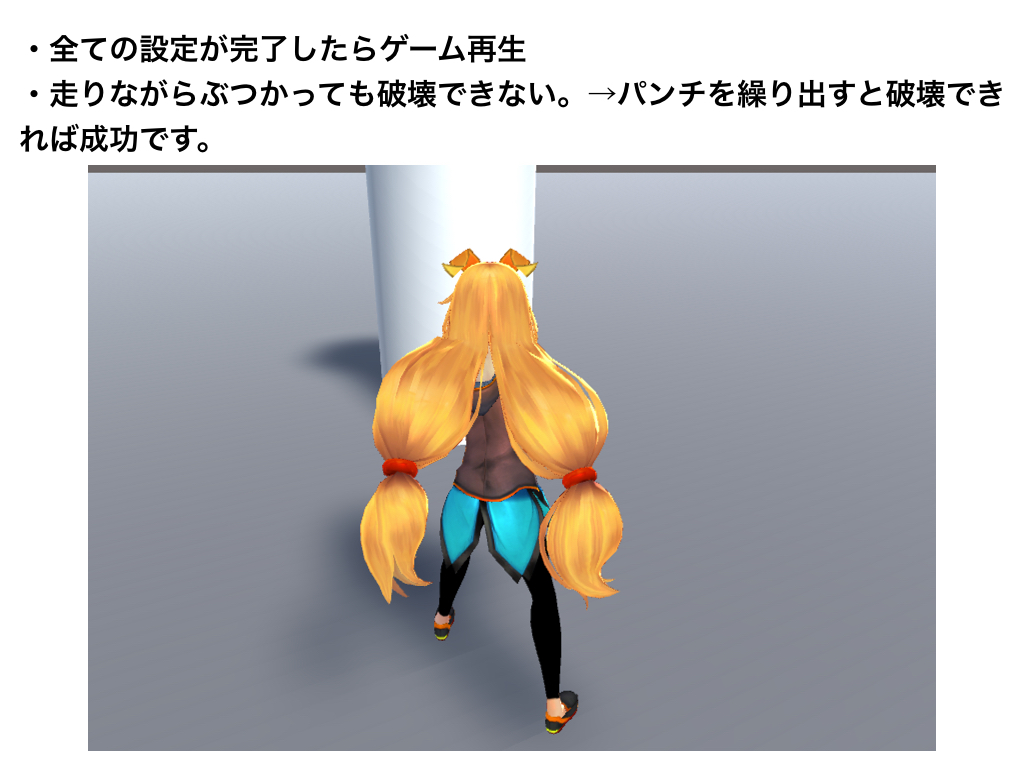
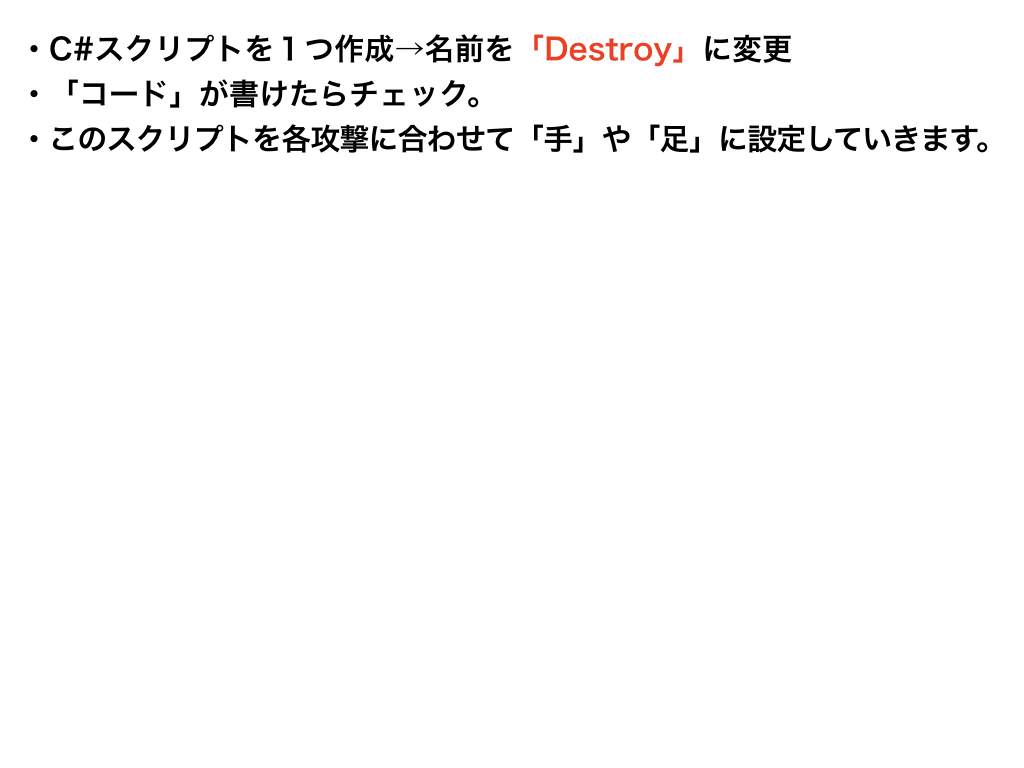
敵を破壊する
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Destroy : MonoBehaviour {
public AudioClip effectSound;
public GameObject effectPrefab;
void OnTriggerEnter(Collider other){
if (other.CompareTag ("Enemy")) {
Destroy (other.gameObject);
AudioSource.PlayClipAtPoint (effectSound, Camera.main.transform.position);
GameObject effect = (GameObject)Instantiate (effectPrefab, other.transform.position, Quaternion.identity);
Destroy (effect, 0.5f);
}
}
}
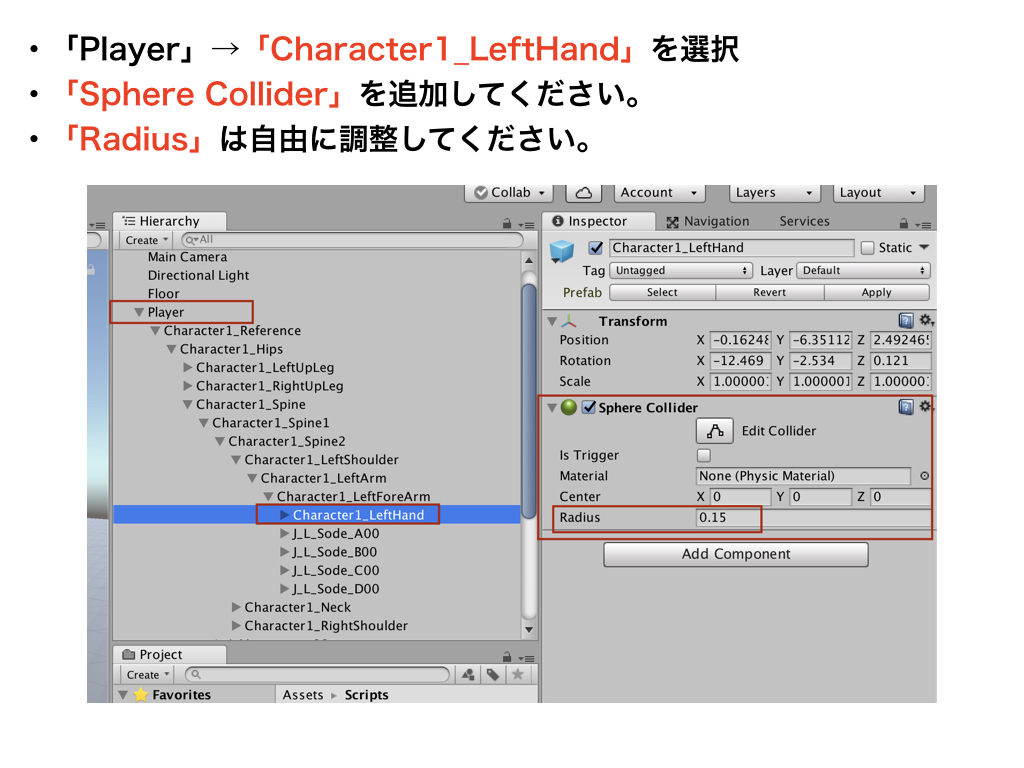
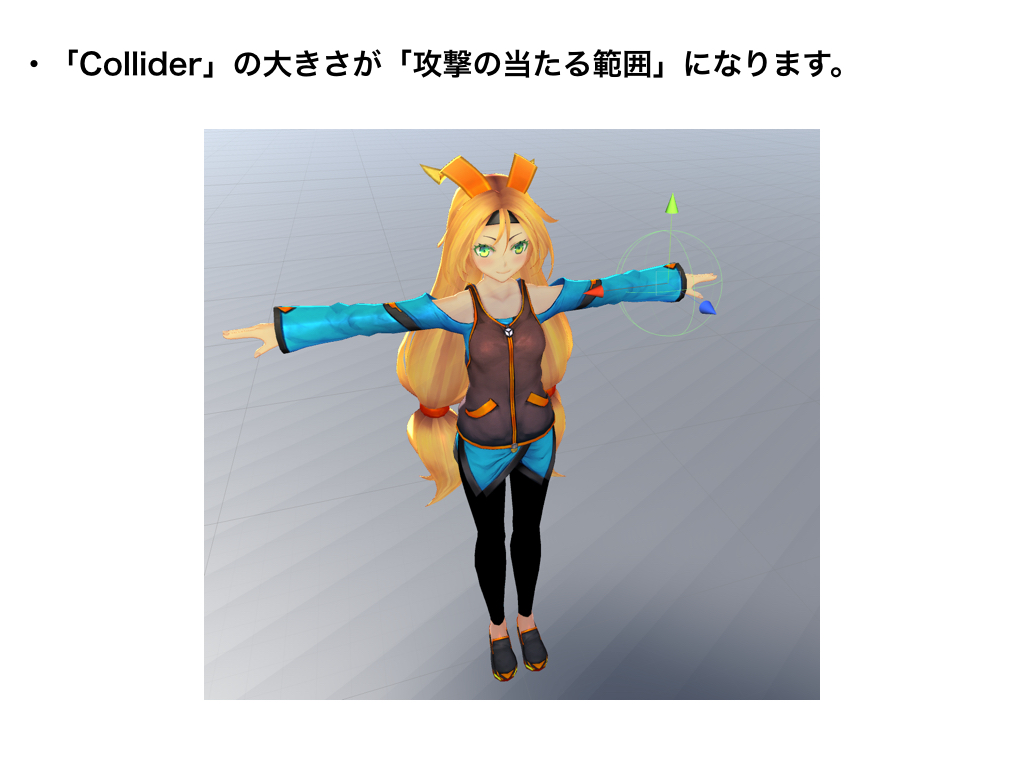
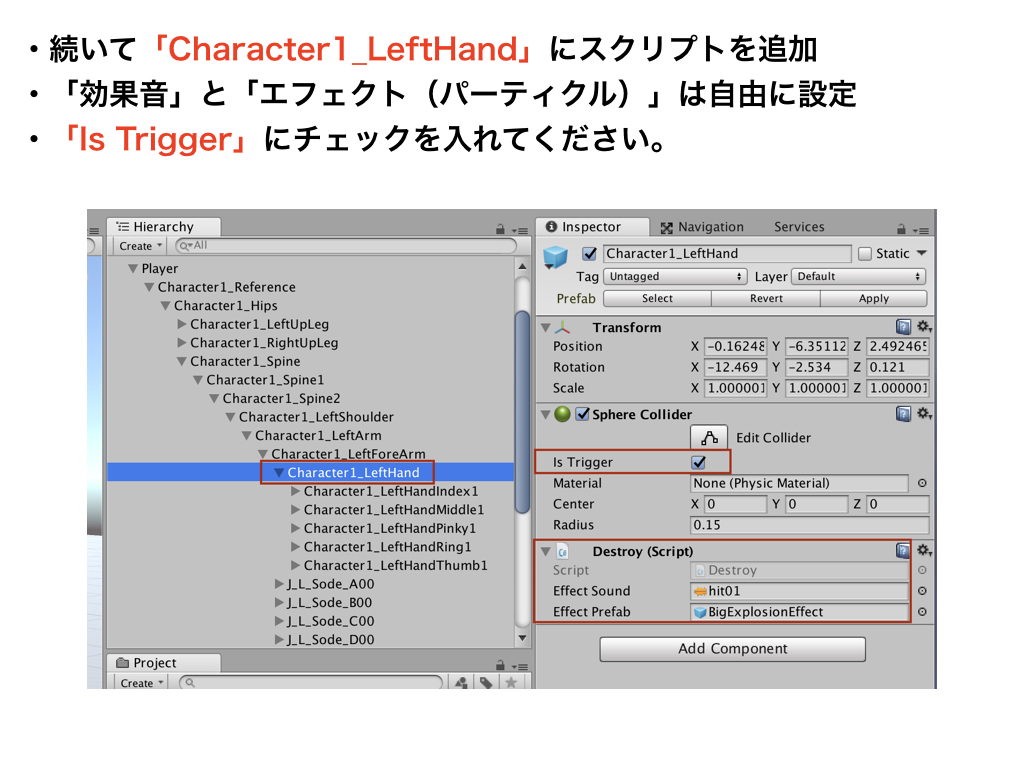
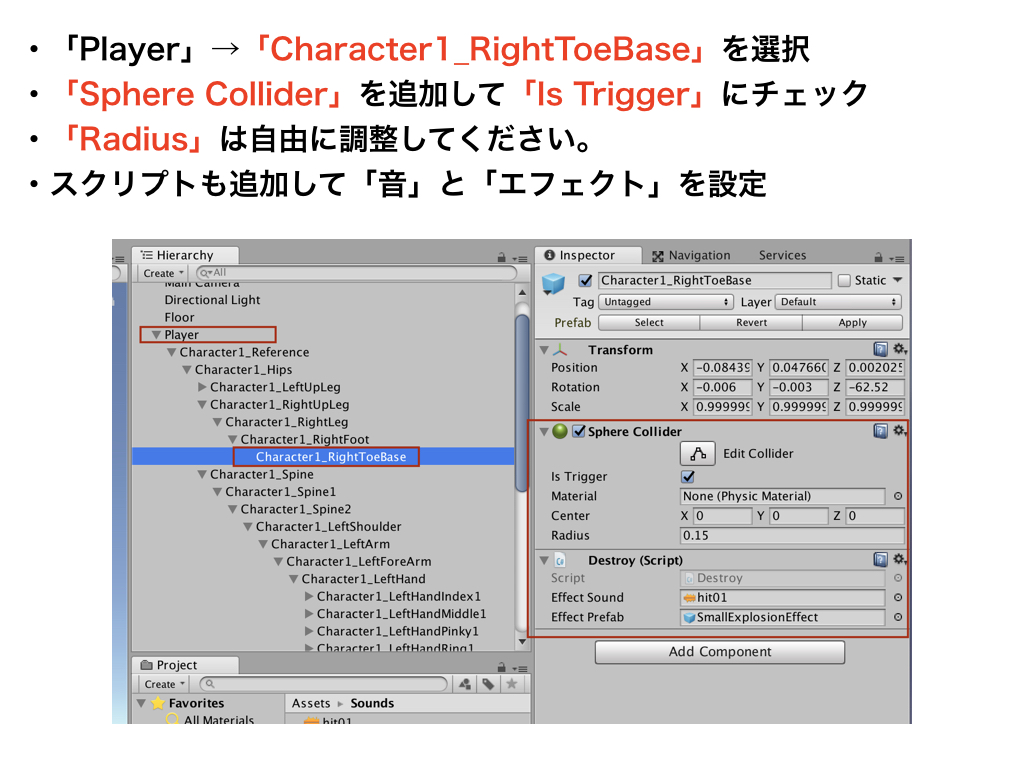
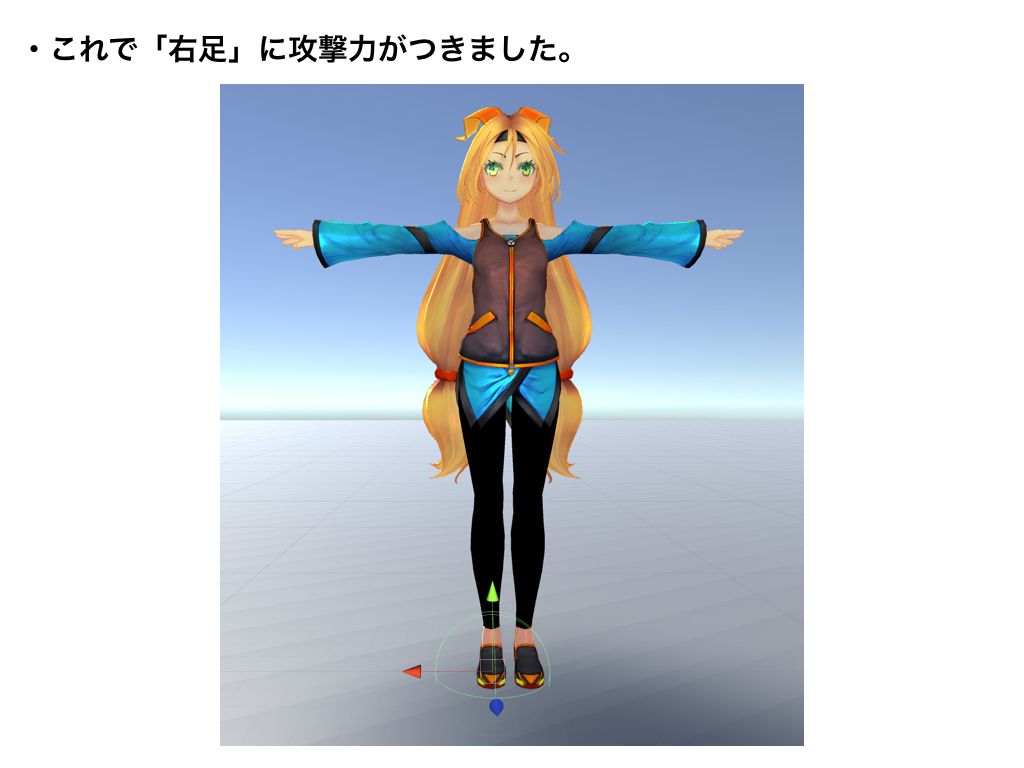
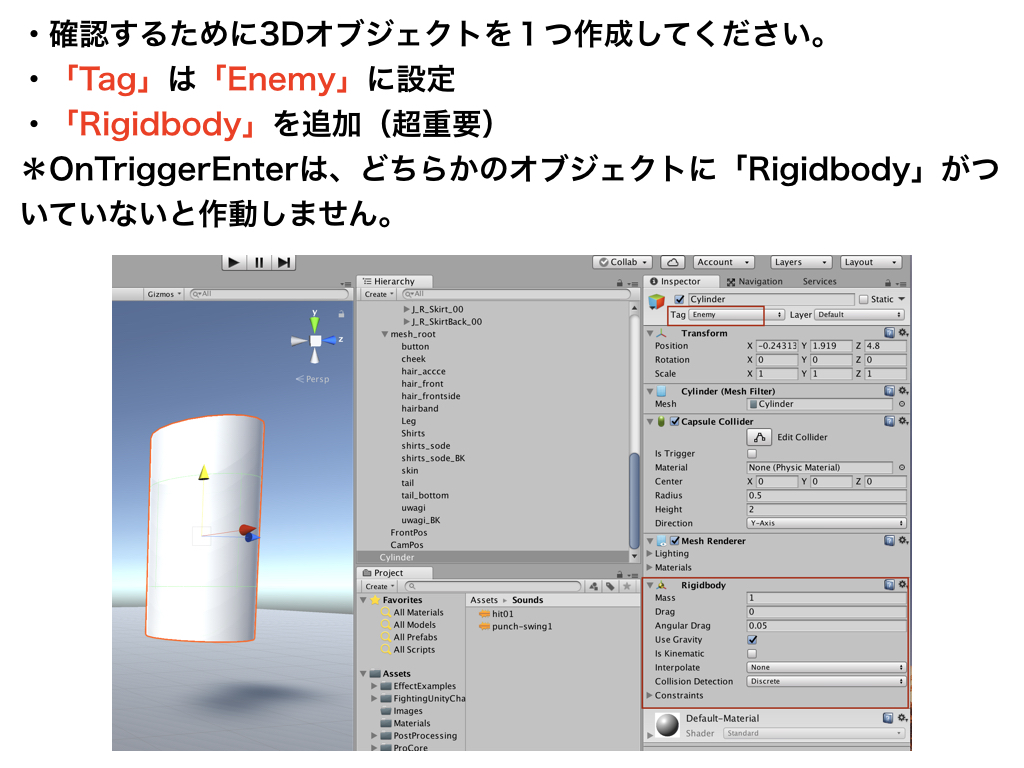
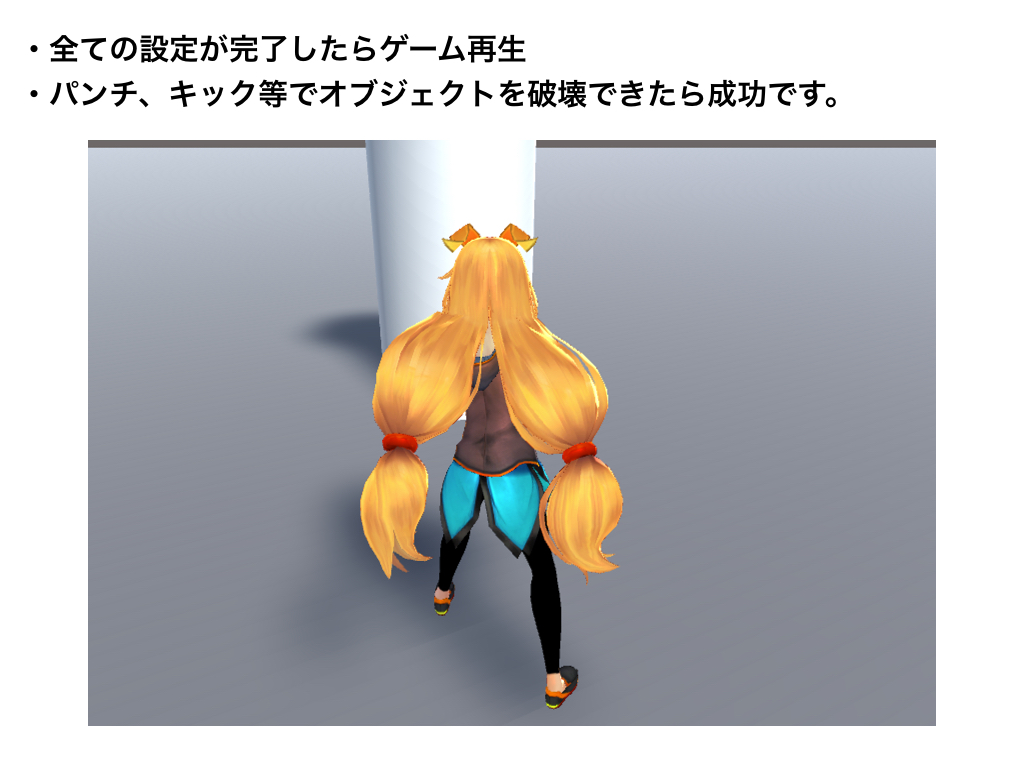
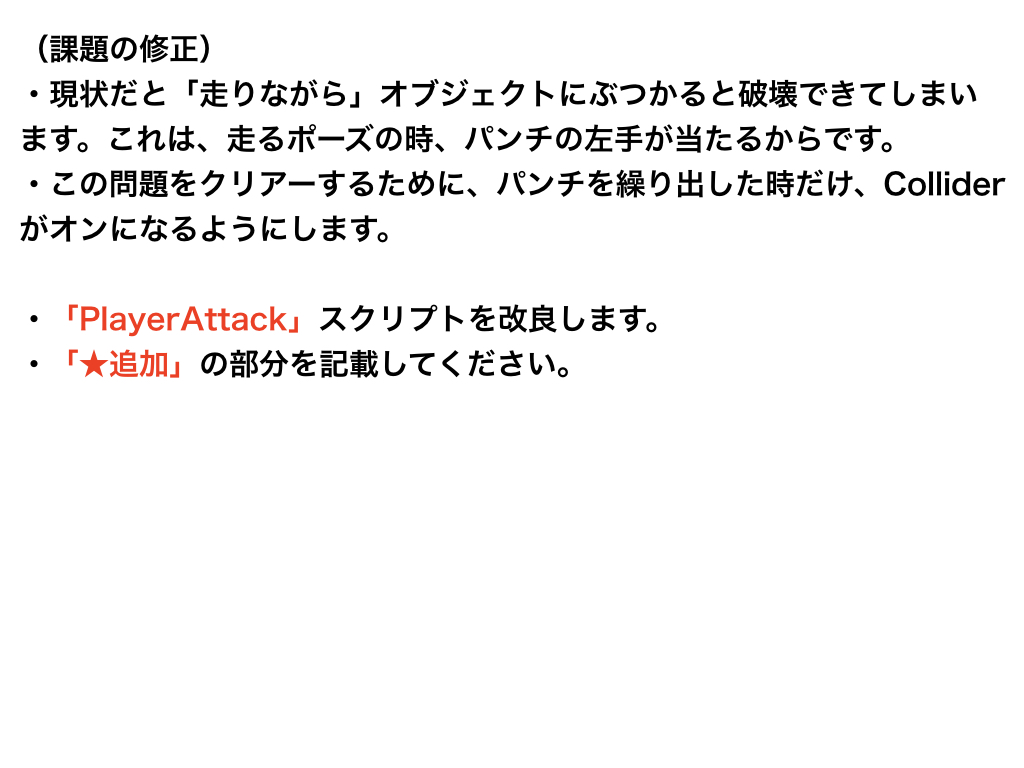
パンチを繰り出した時だけコライダーをオンにする
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerAttack : MonoBehaviour {
private Animator anim;
public AudioClip punchSound;
// ★追加
private Collider left_hand_col;
void Start () {
anim = GetComponent<Animator> ();
// ★追加
left_hand_col = GameObject.Find("Character1_LeftHand").GetComponent<SphereCollider>();
}
void Update () {
// Qボタンを押すとパンチ
if (Input.GetKeyDown (KeyCode.Q)) {
anim.SetBool ("Jab", true);
AudioSource.PlayClipAtPoint (punchSound, Camera.main.transform.position);
// ★追加
// 当たり判定をとるためにコライダーをオンの状態にする。
left_hand_col.enabled = true;
// ★追加
// 0.15秒後にコライダーをオフの状態に戻す。
Invoke("Reset", 0.15f);
}
// Eボタンを押すとハイキック
if (Input.GetKeyDown (KeyCode.E)) {
anim.SetBool ("Hikick", true);
}
// Sボタンを押すと回転キック
if (Input.GetKeyDown (KeyCode.S)) {
anim.SetBool ("Spinkick", true);
}
// Zボタンを押すとムーンソルトキック
if (Input.GetKeyDown (KeyCode.Z)) {
anim.SetBool ("SAMK", true);
}
}
// ★追加
void Reset(){
left_hand_col.enabled = false;
}
}
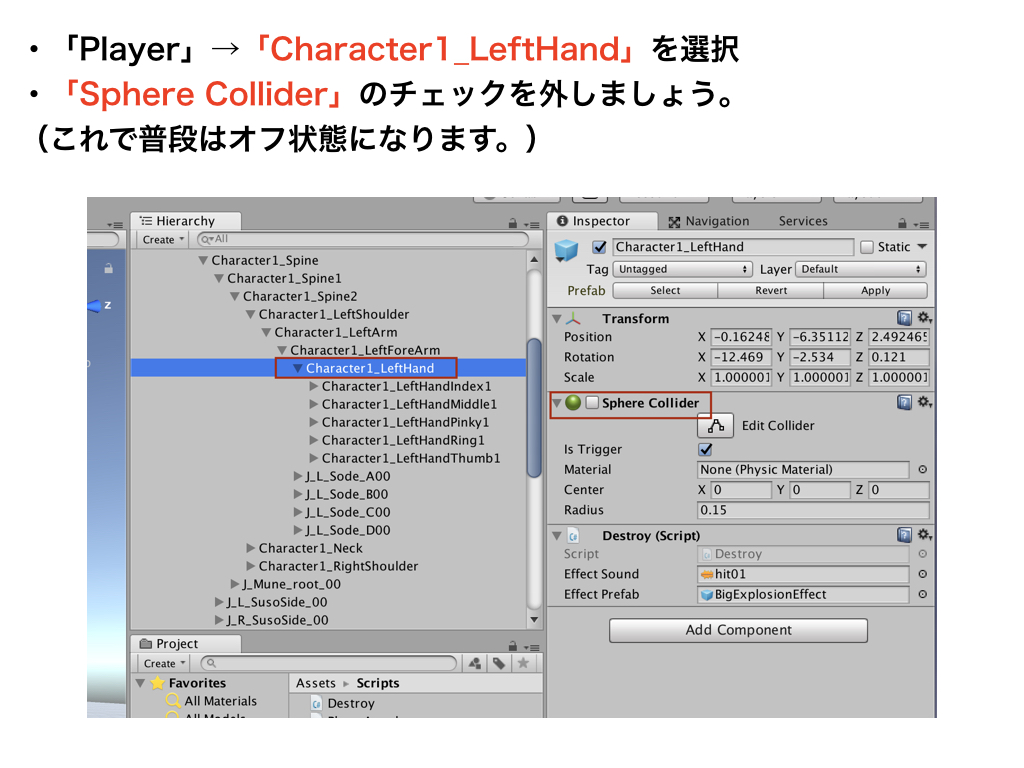
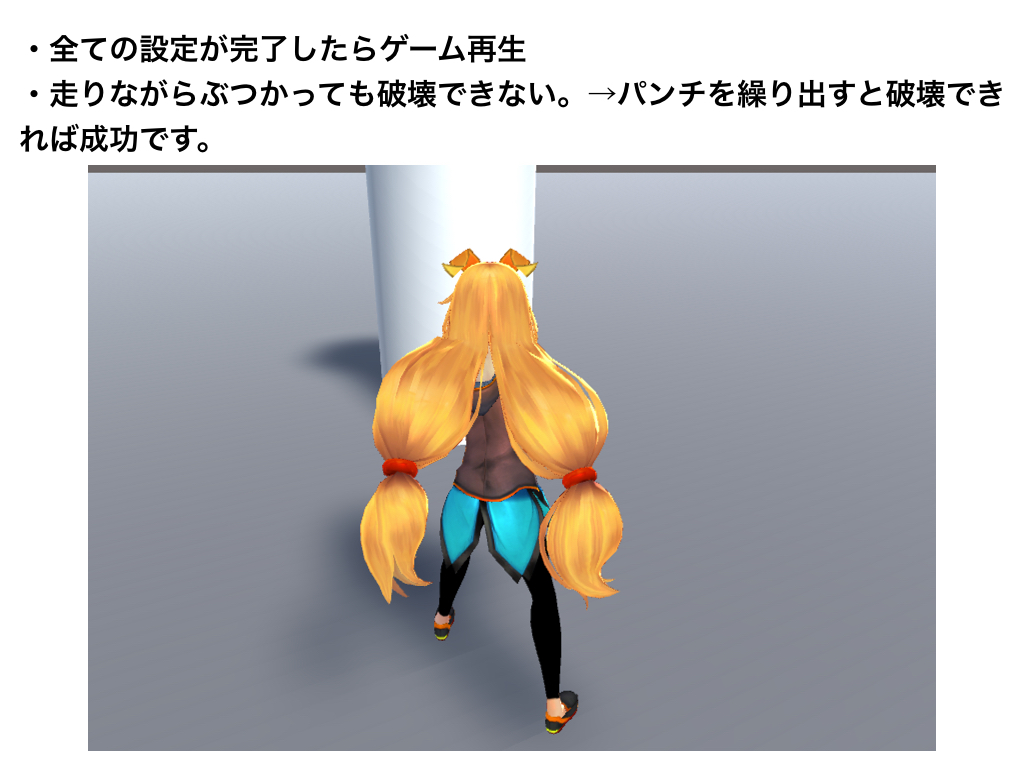
攻撃力の作成