動く敵の作り方1(Vライン)
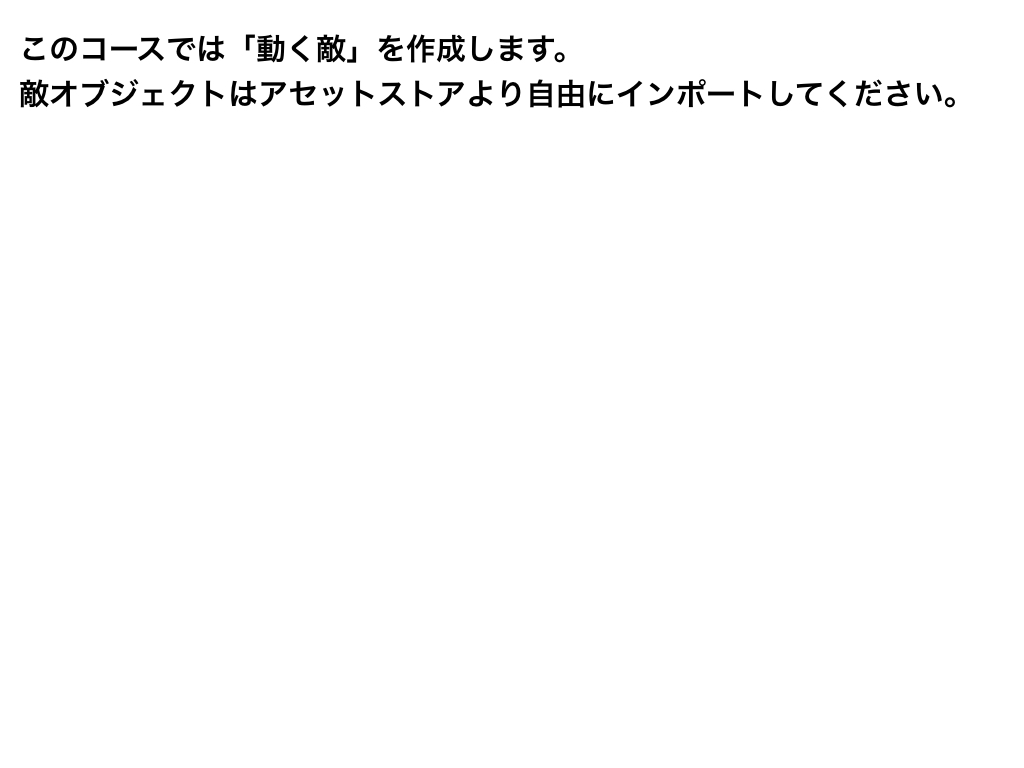
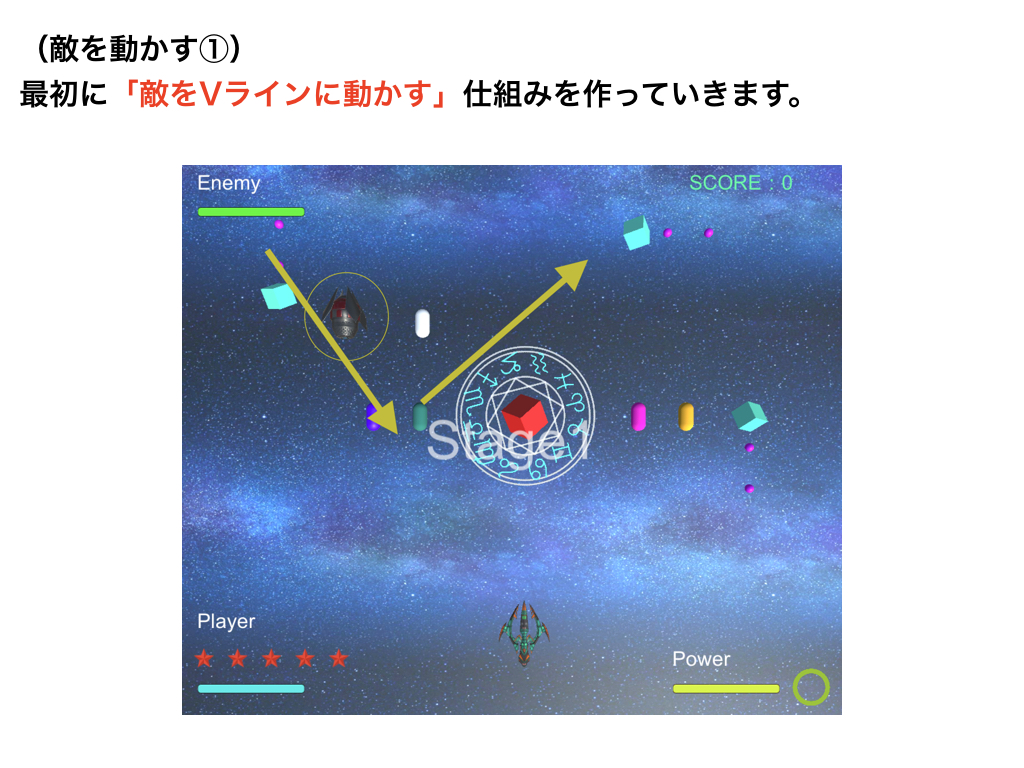
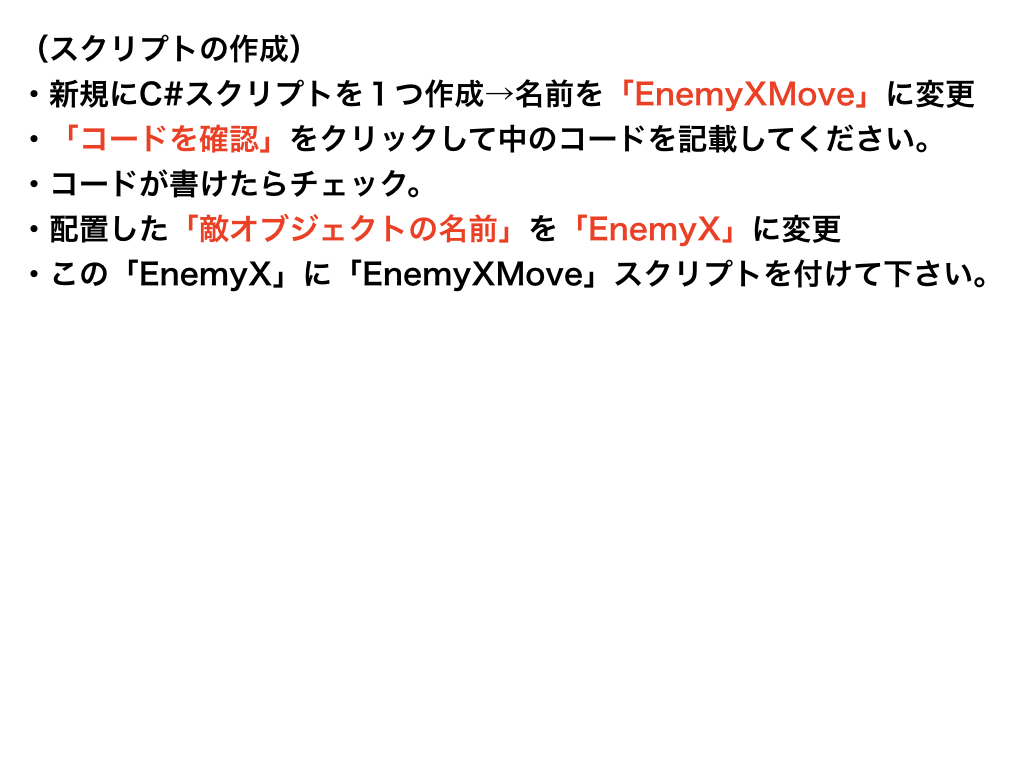
敵を動かす(Vライン)
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class EnemyXMove : MonoBehaviour
{
[Range(0, 50)]
public float moveDistance;
private Vector3 pos;
private bool isReturn = false;
private void Update()
{
pos = transform.position;
if (pos.z > 0 && isReturn == false)
{
// Translate(x軸, y軸, z軸)
// x軸がプラス →方向のベクトル
// z軸がマイナス ↓方向のベクトル
// この2つのベクトルを合成すると「↘️」方向のベクトルになる。(→ + ↓ = ↘️)
transform.Translate(moveDistance * Time.deltaTime, 0, -moveDistance * Time.deltaTime, Space.World);
}
else // pos.zが1以下になった時、進行方向を変化させる。
{
isReturn = true;
// x軸がプラス →方向のベクトル
// z軸がマイナス ↑方向のベクトル
// この2つのベクトルを合成すると「↗️」方向のベクトルになる。(→ + ↑ = ↗️)
transform.Translate(moveDistance * Time.deltaTime, 0, moveDistance * Time.deltaTime, Space.World);
}
}
}
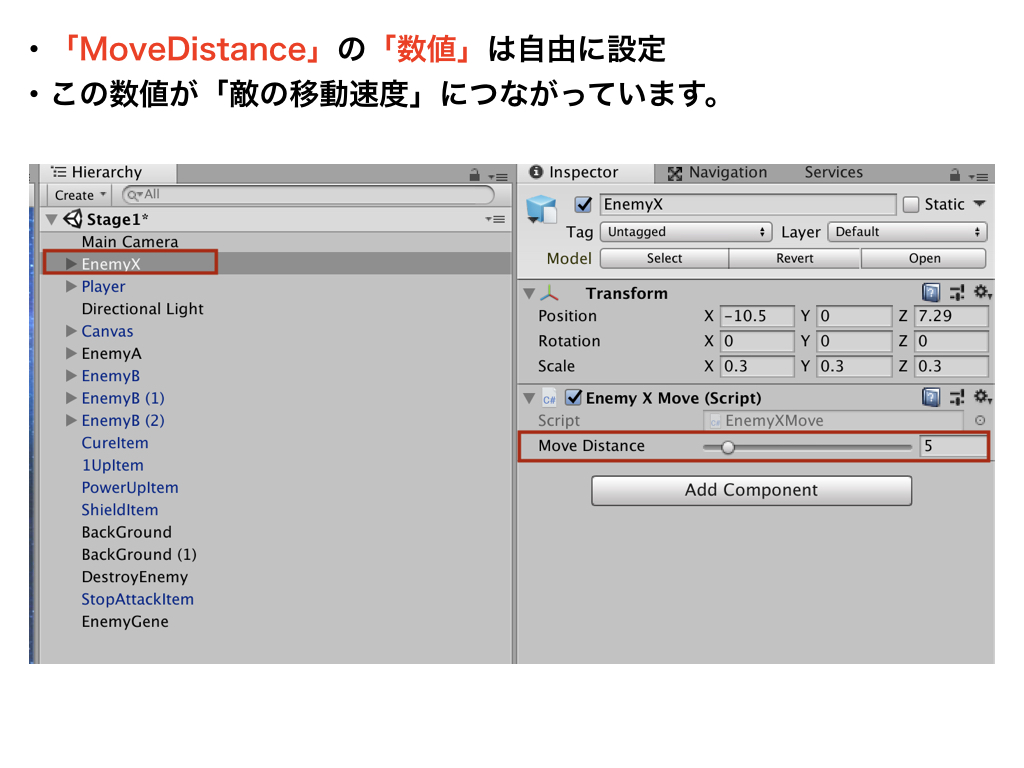
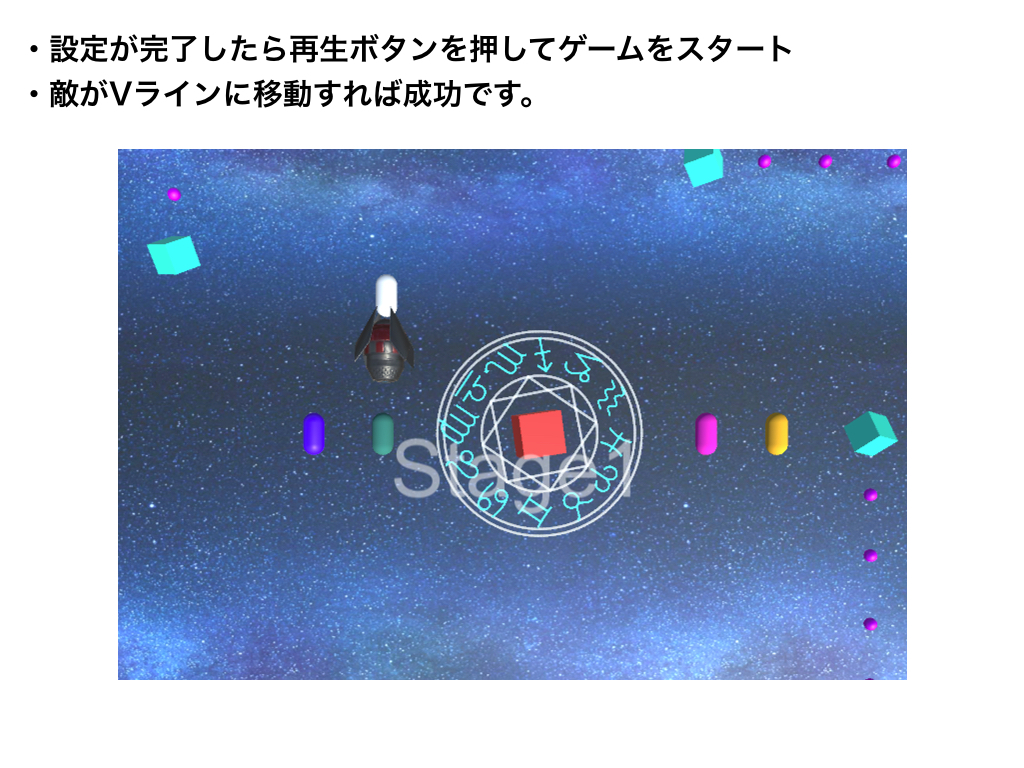
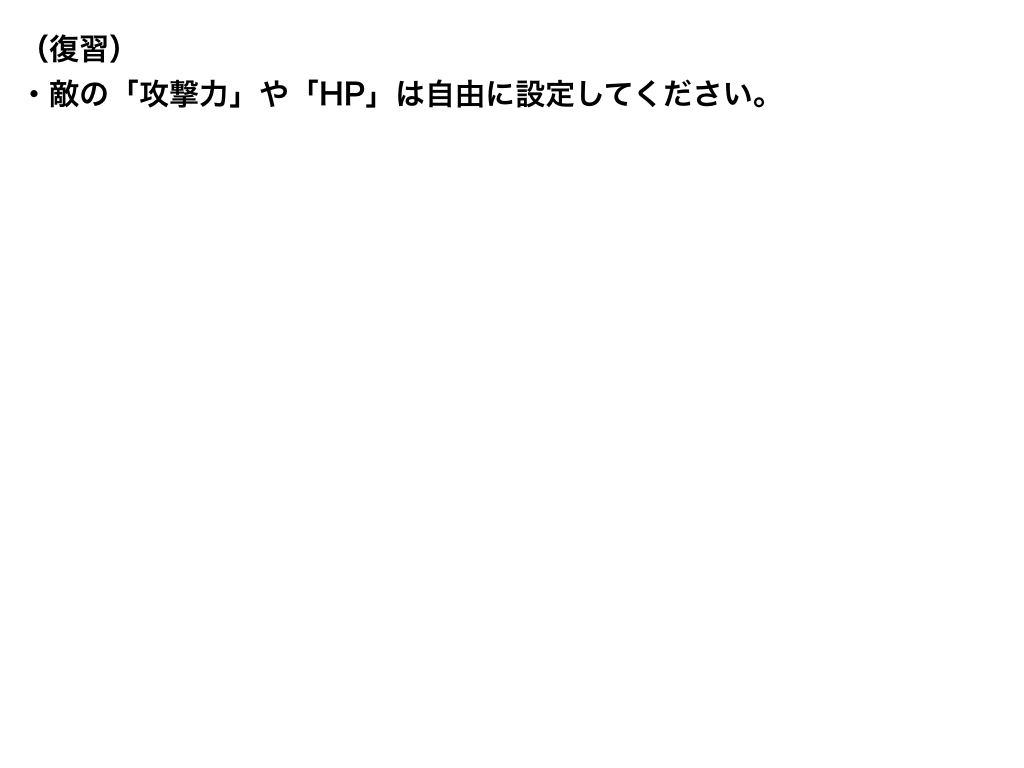
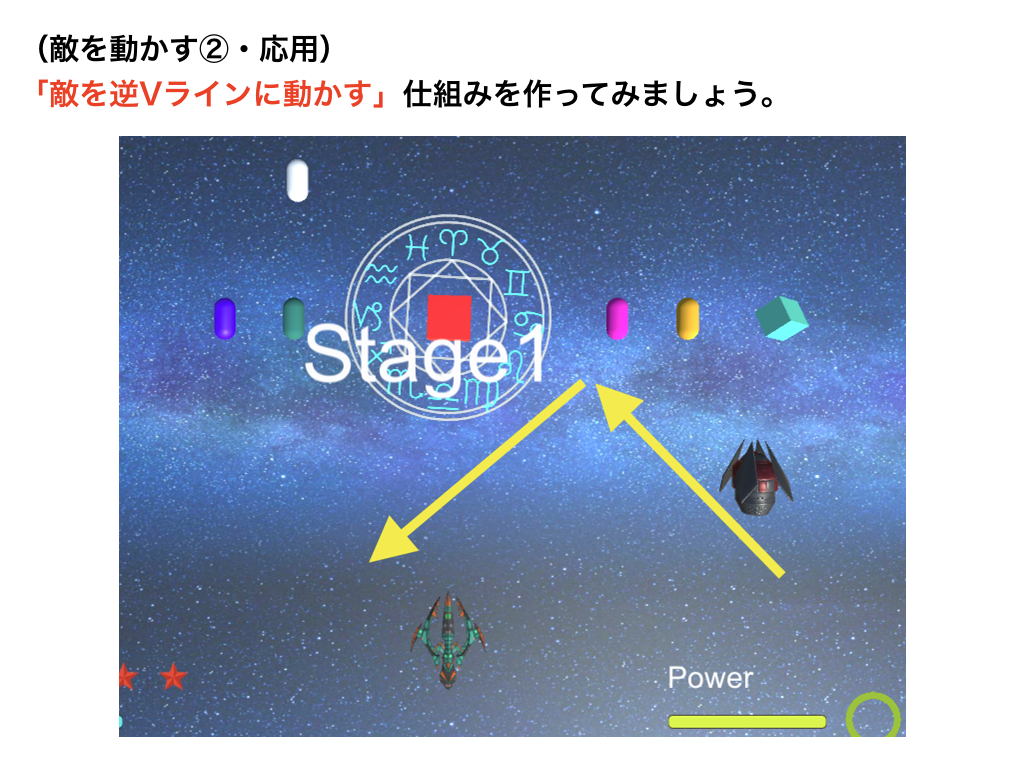
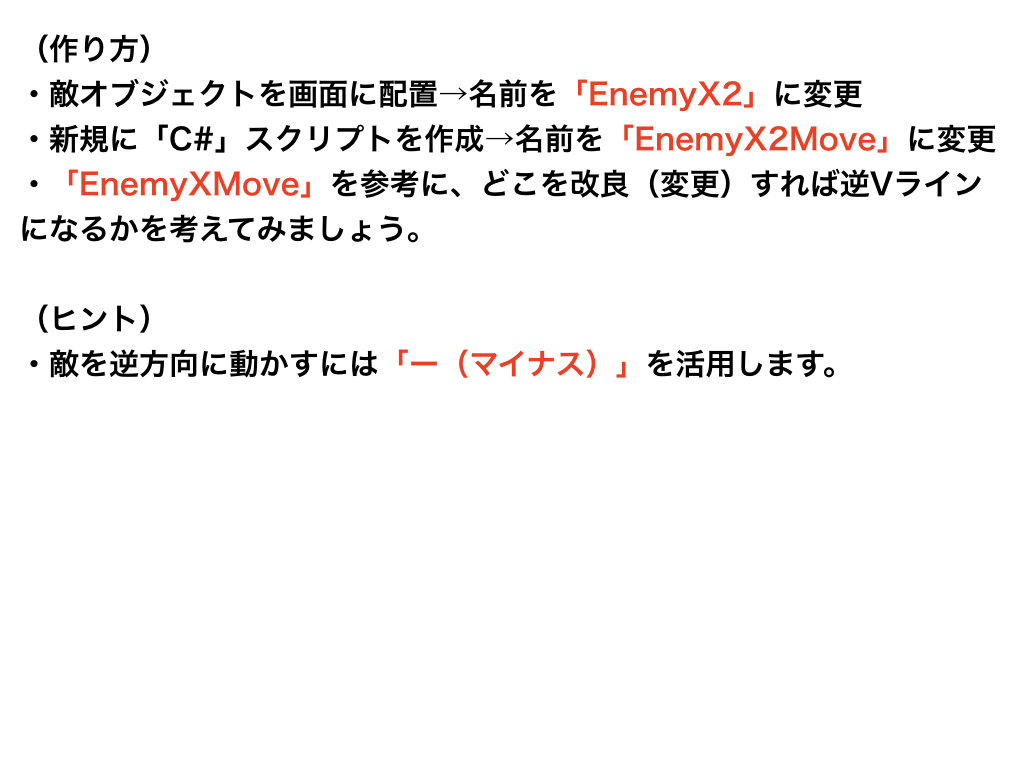
敵を動かす(逆Vライン)
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class EnemyX2Move : MonoBehaviour
{
[Range(0, 50)]
public float moveDistance;
private Vector3 pos;
private bool isReturn = false;
void Update()
{
pos = transform.position;
if (pos.z < 0 && isReturn == false)
{
transform.Translate(-moveDistance * Time.deltaTime, 0, moveDistance * Time.deltaTime, Space.World);
}
else
{
isReturn = true;
transform.Translate(-moveDistance * Time.deltaTime, 0, -moveDistance * Time.deltaTime, Space.World);
}
}
}
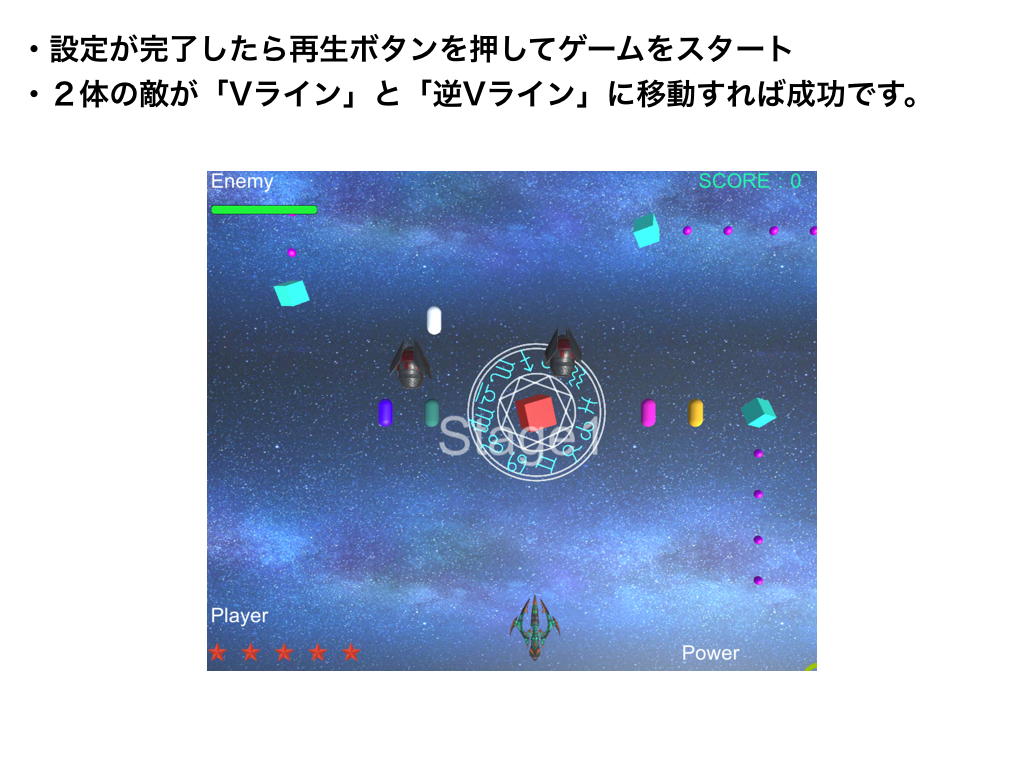
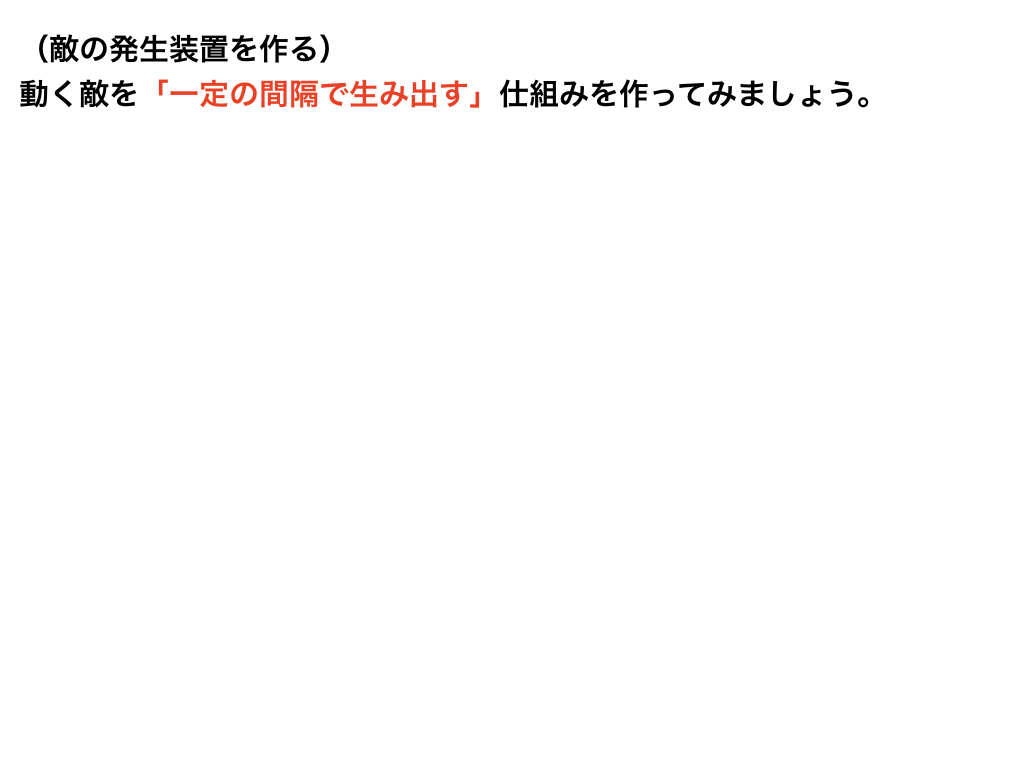
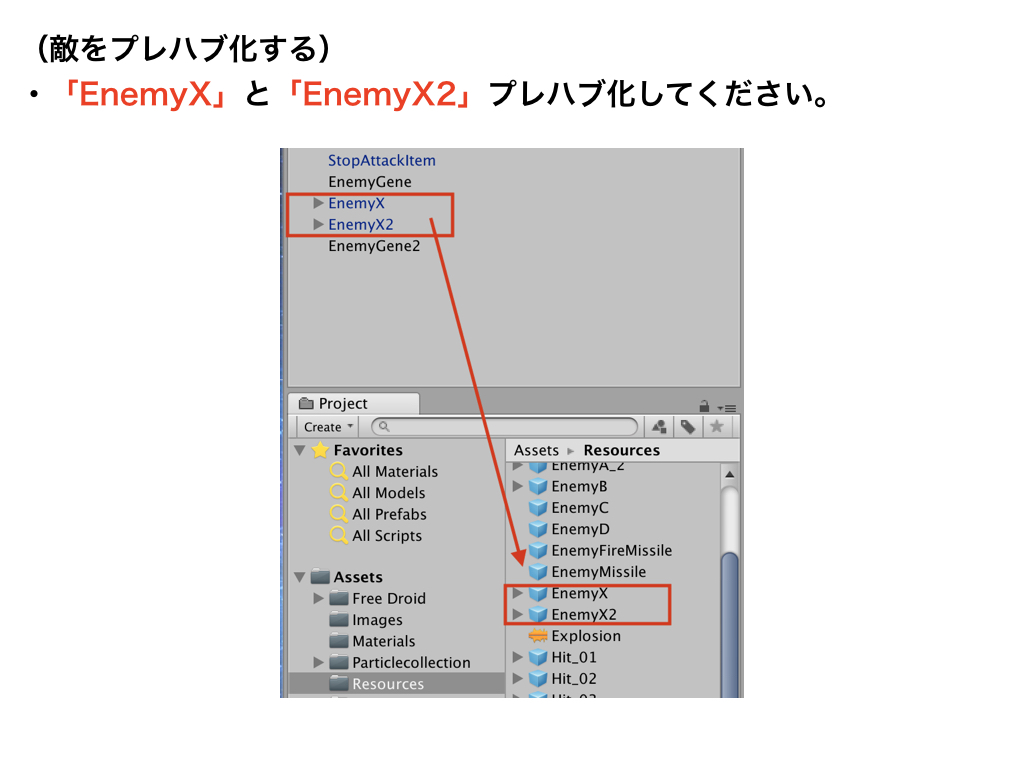
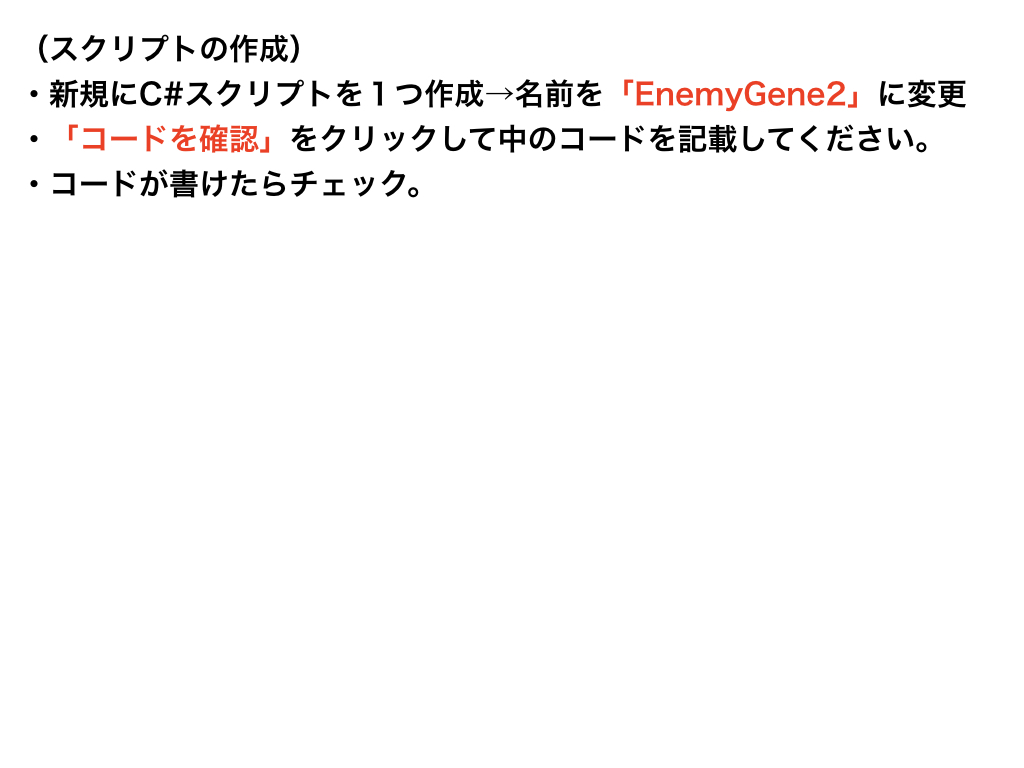
敵の発生装置
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class EnemyGene2 : MonoBehaviour
{
public GameObject enemyPrefab;
public int geneAmount;
[Range(0, 1.5f)]
public float waitTime;
private void Start()
{
StartCoroutine(GeneEnemy());
}
IEnumerator GeneEnemy()
{
for (int i = 0; i < geneAmount; i++)
{
GameObject enemy = Instantiate(enemyPrefab, transform.position, Quaternion.identity);
Destroy(enemy, 15.0f);
yield return new WaitForSeconds(waitTime);
}
}
}
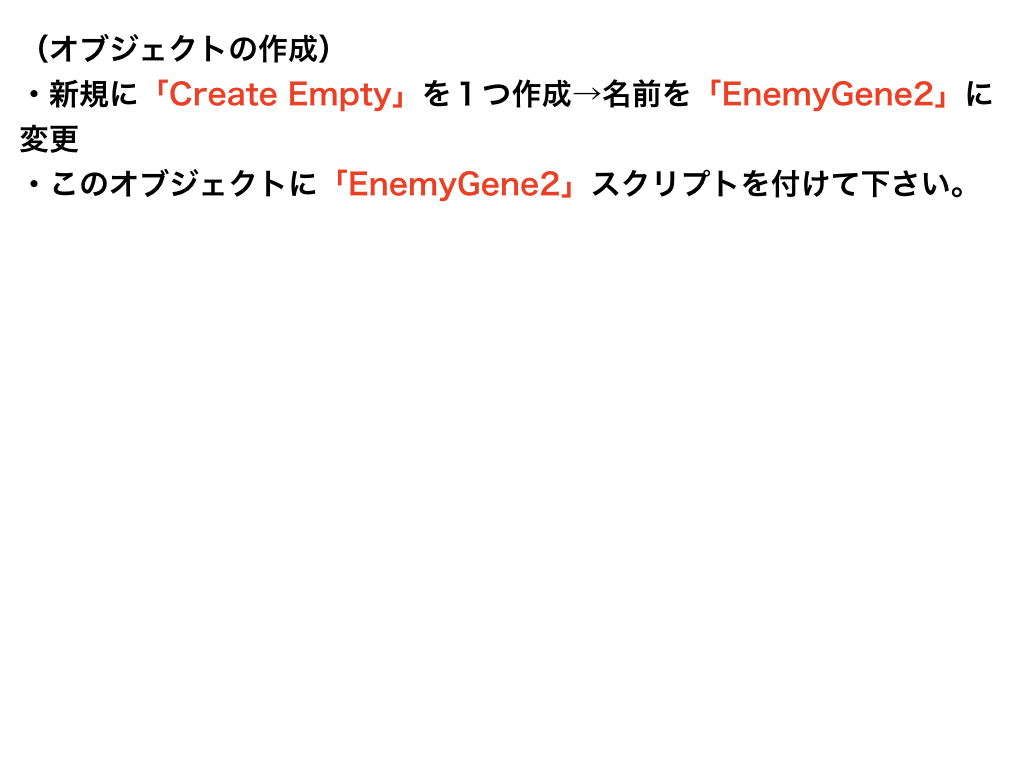
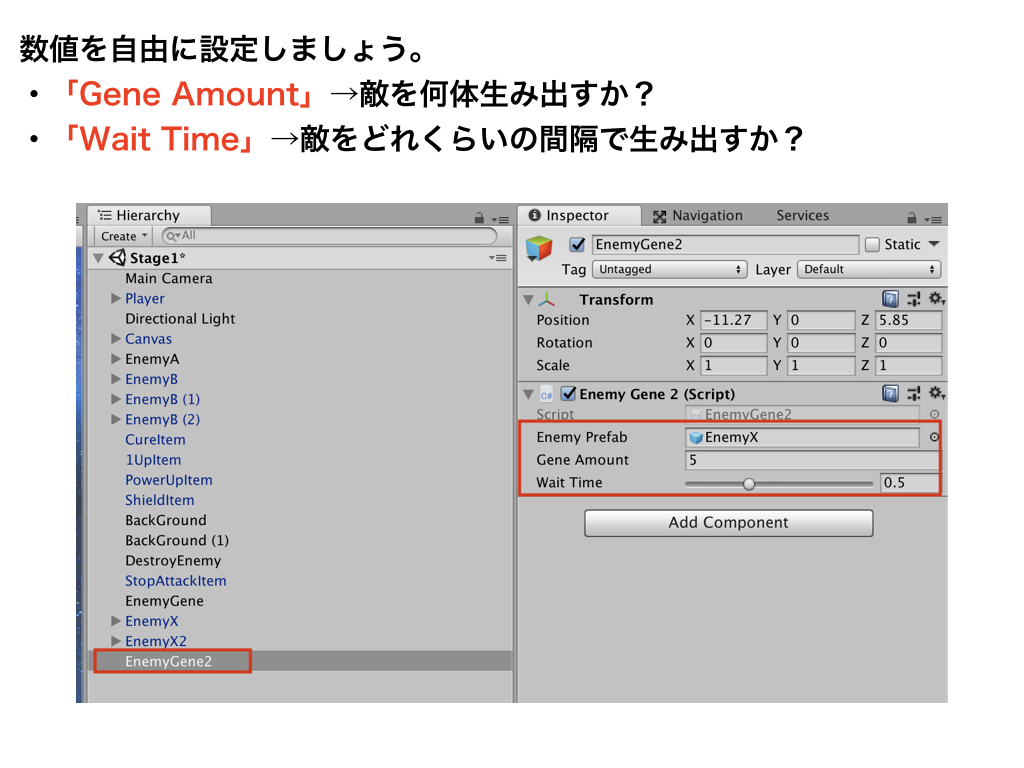
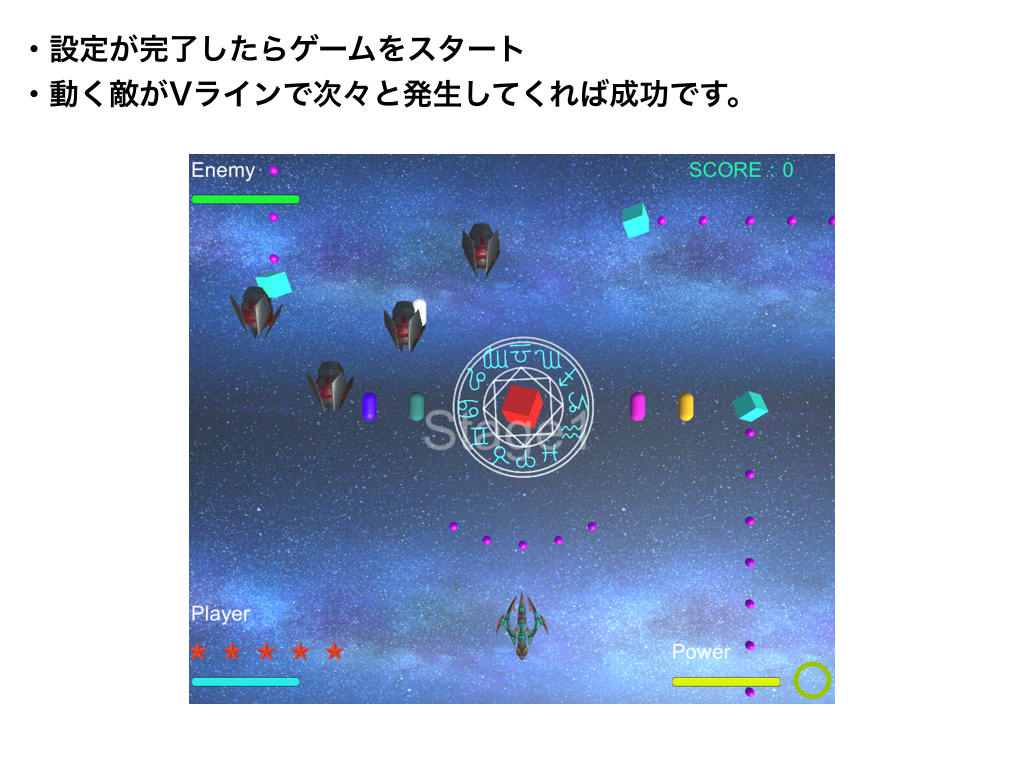
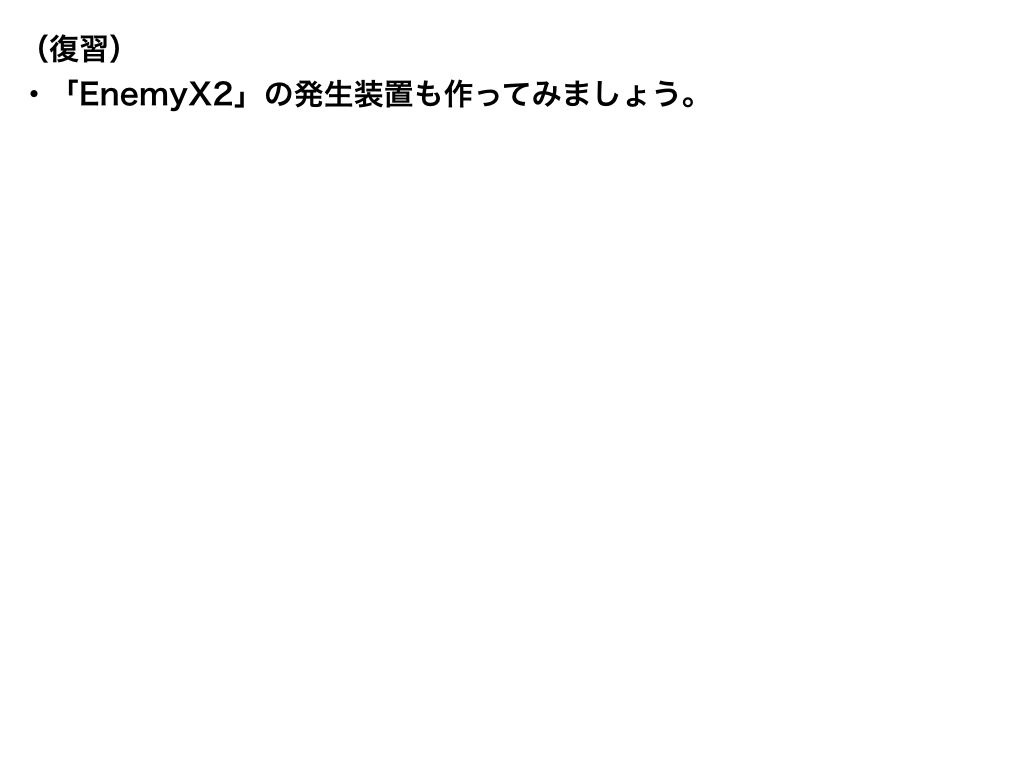
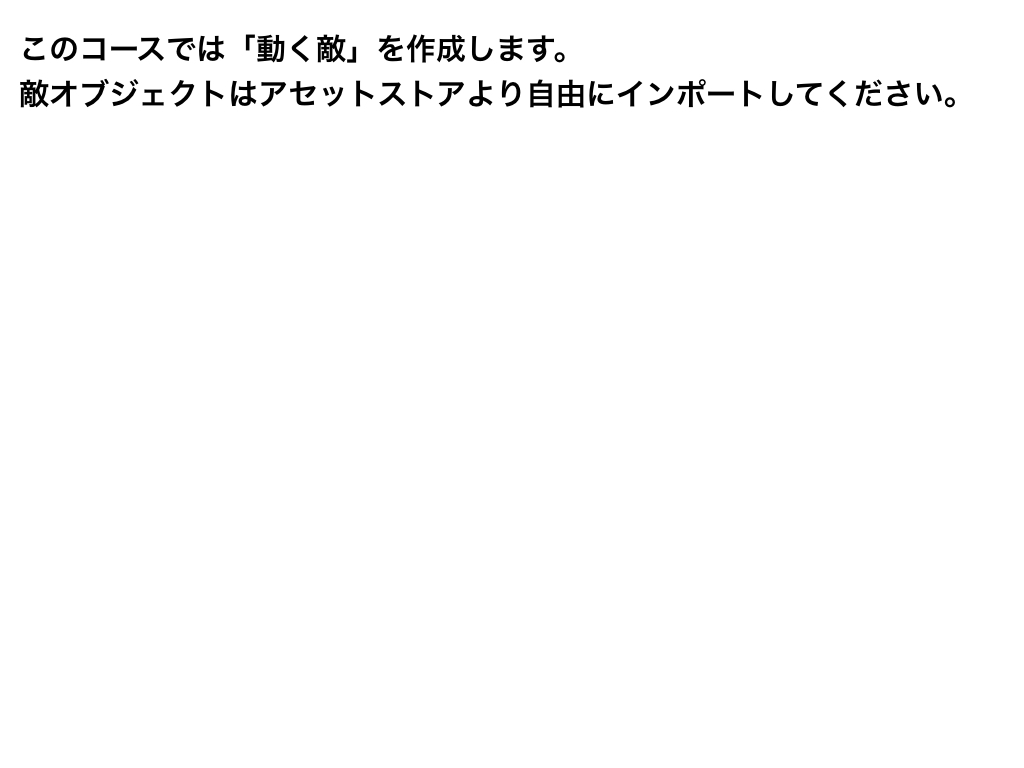
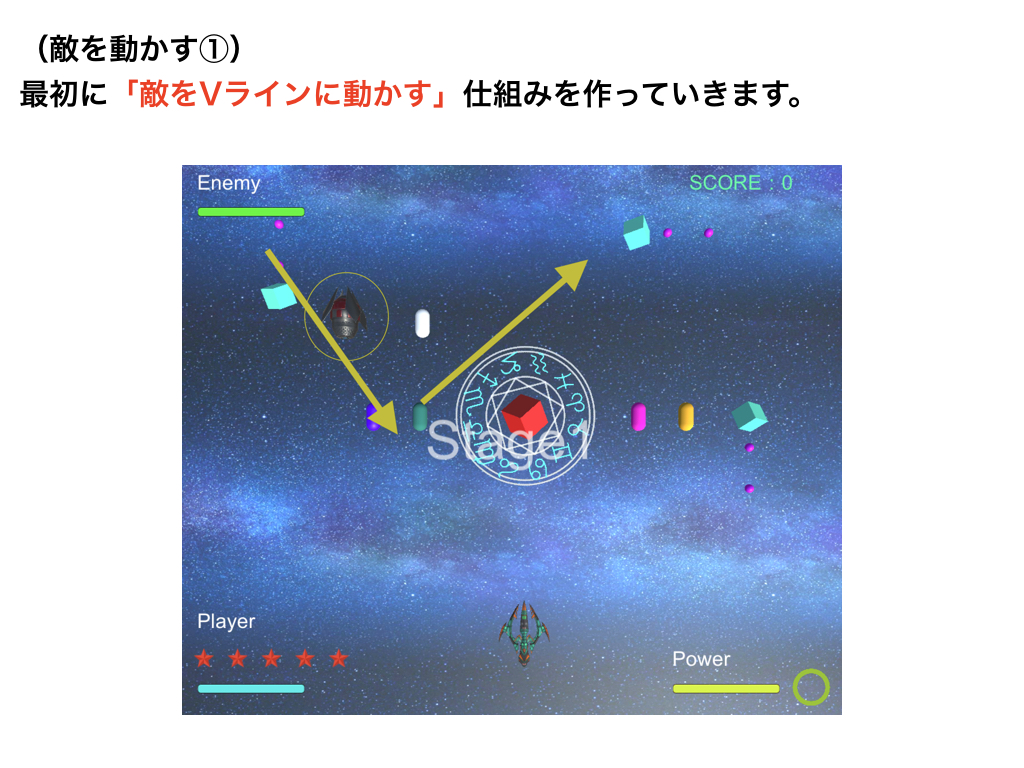
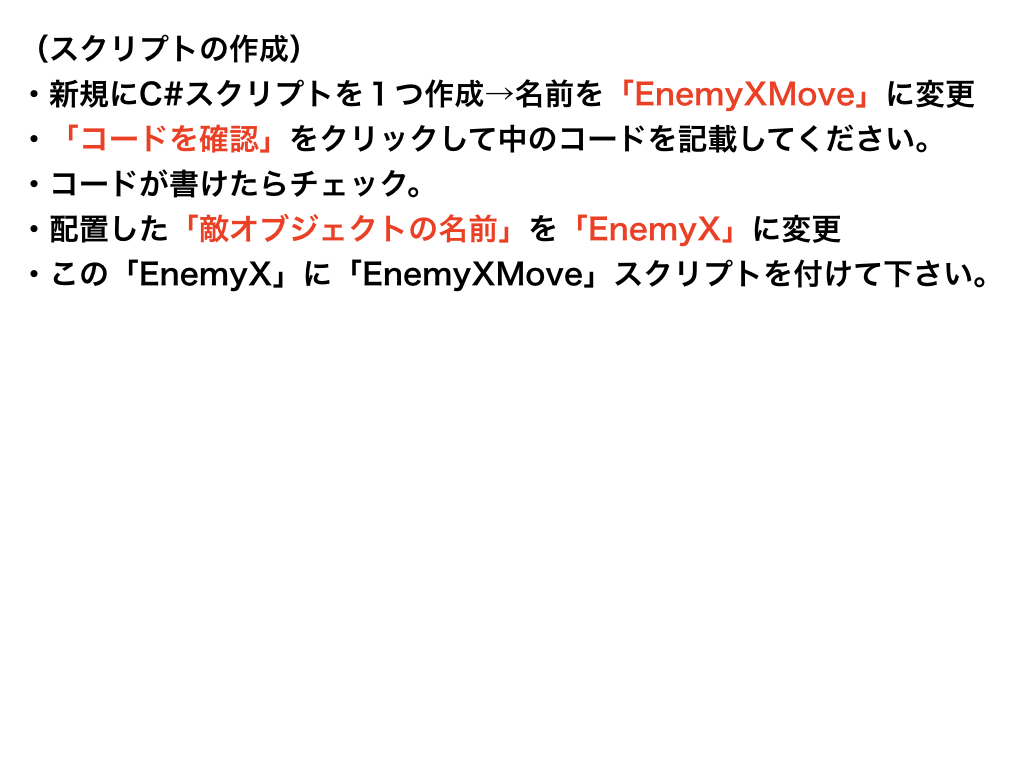
敵を動かす(Vライン)
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class EnemyXMove : MonoBehaviour
{
[Range(0, 50)]
public float moveDistance;
private Vector3 pos;
private bool isReturn = false;
private void Update()
{
pos = transform.position;
if (pos.z > 0 && isReturn == false)
{
// Translate(x軸, y軸, z軸)
// x軸がプラス →方向のベクトル
// z軸がマイナス ↓方向のベクトル
// この2つのベクトルを合成すると「↘️」方向のベクトルになる。(→ + ↓ = ↘️)
transform.Translate(moveDistance * Time.deltaTime, 0, -moveDistance * Time.deltaTime, Space.World);
}
else // pos.zが1以下になった時、進行方向を変化させる。
{
isReturn = true;
// x軸がプラス →方向のベクトル
// z軸がマイナス ↑方向のベクトル
// この2つのベクトルを合成すると「↗️」方向のベクトルになる。(→ + ↑ = ↗️)
transform.Translate(moveDistance * Time.deltaTime, 0, moveDistance * Time.deltaTime, Space.World);
}
}
}
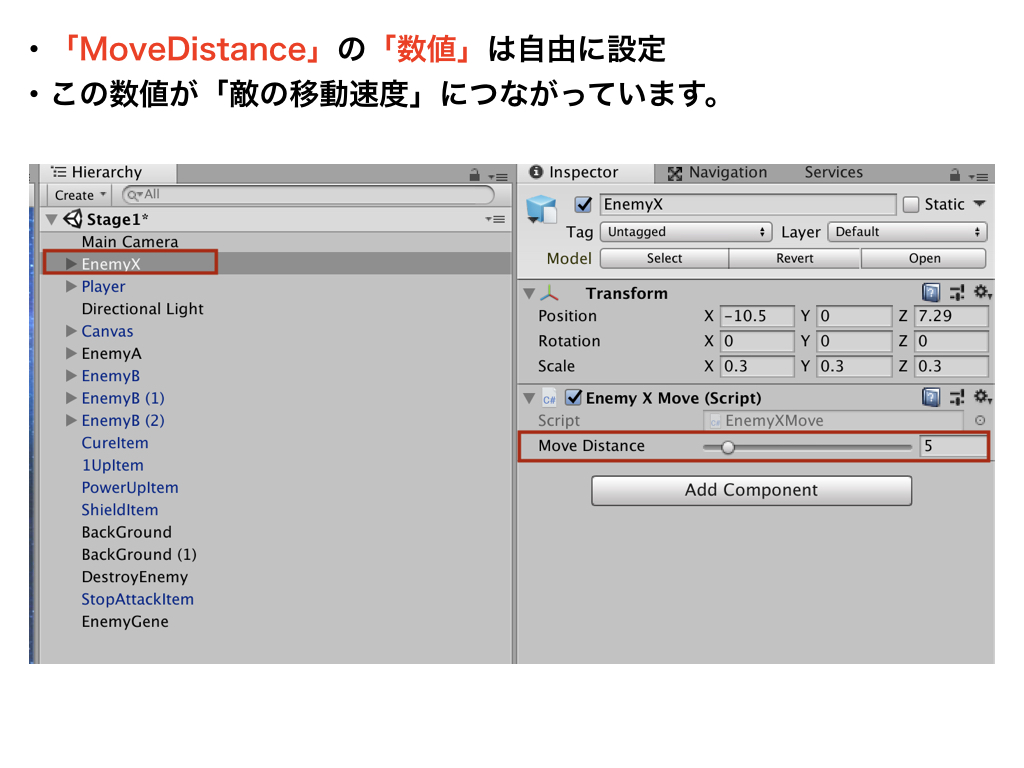
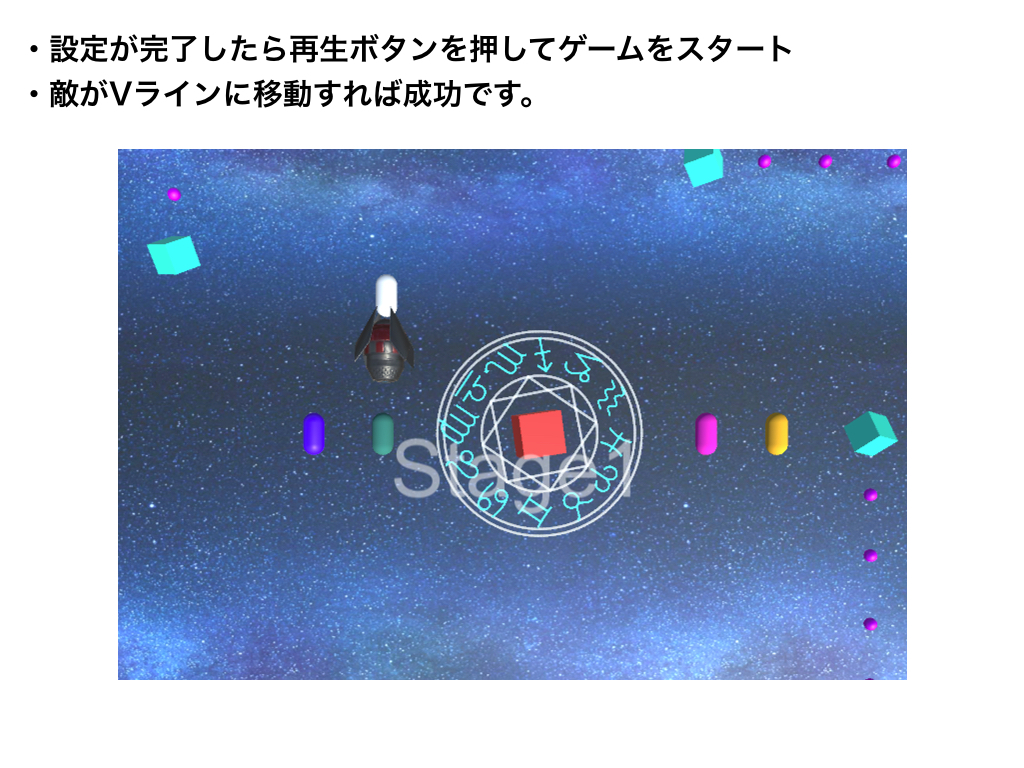
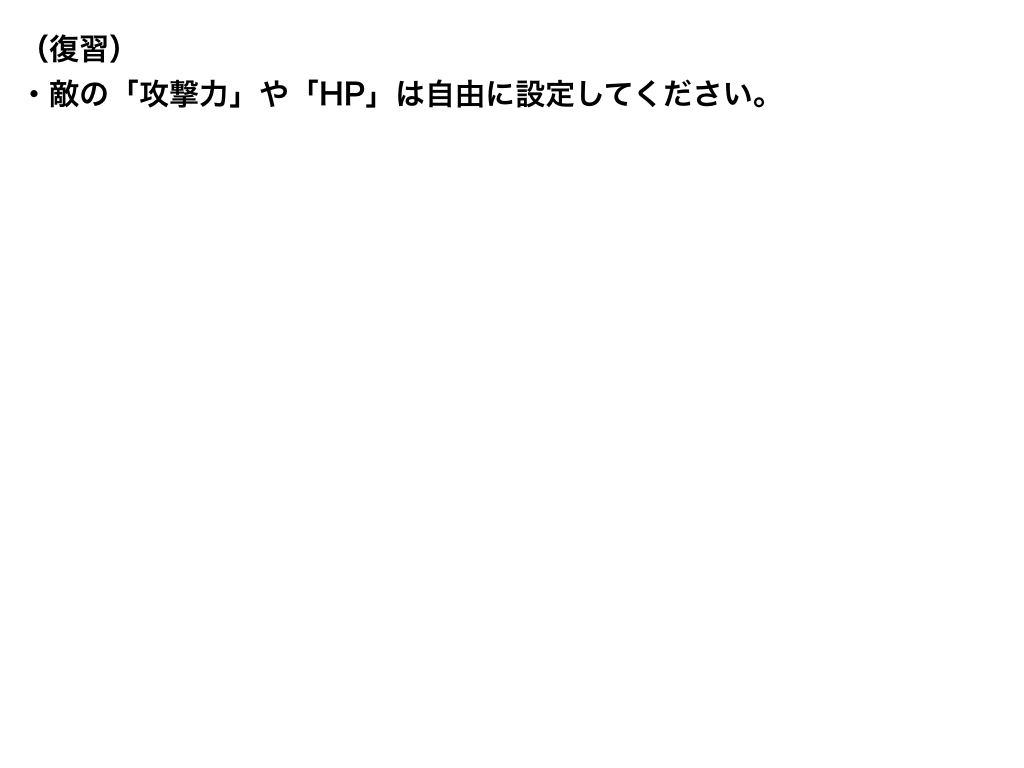
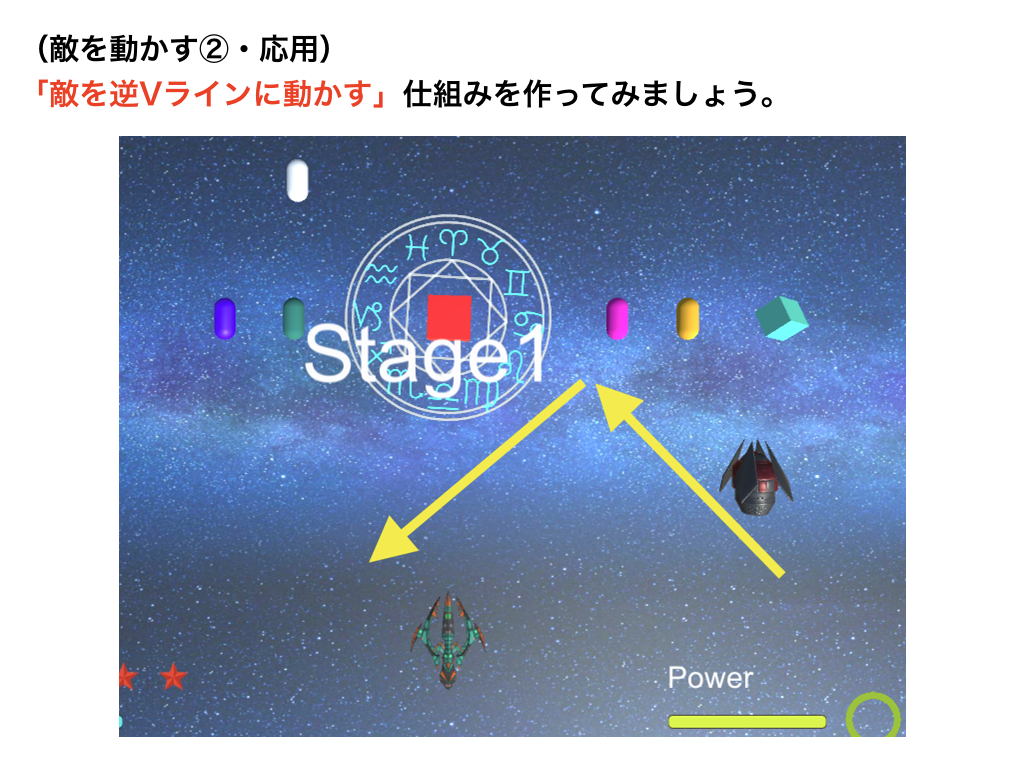
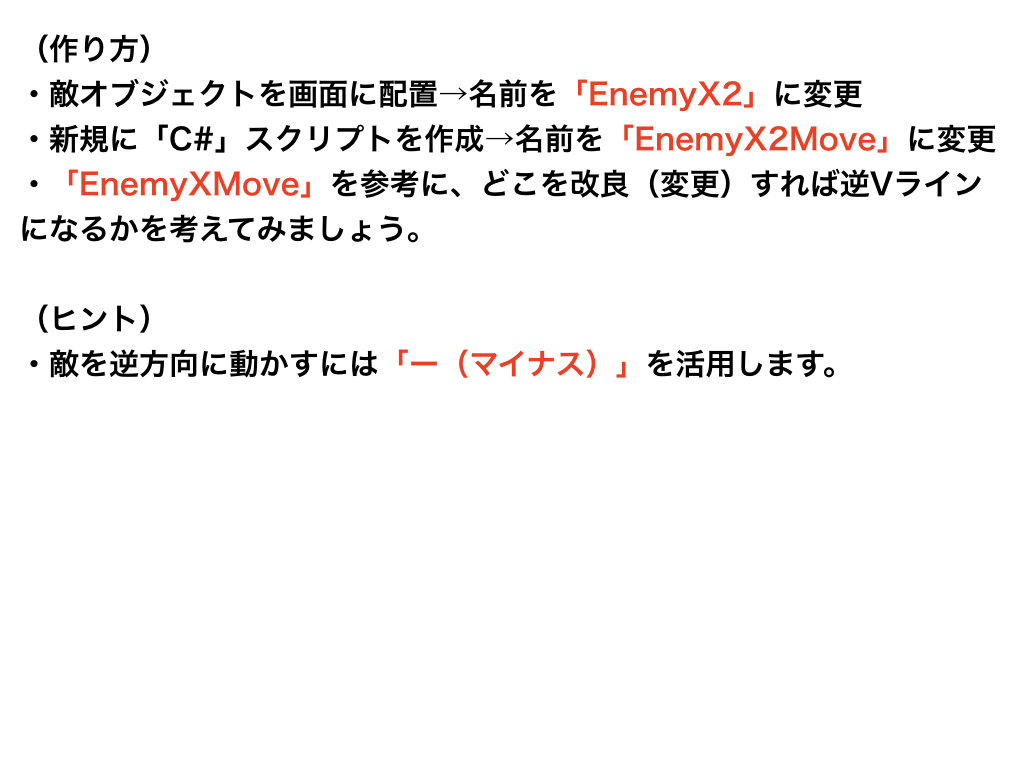
敵を動かす(逆Vライン)
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class EnemyX2Move : MonoBehaviour
{
[Range(0, 50)]
public float moveDistance;
private Vector3 pos;
private bool isReturn = false;
void Update()
{
pos = transform.position;
if (pos.z < 0 && isReturn == false)
{
transform.Translate(-moveDistance * Time.deltaTime, 0, moveDistance * Time.deltaTime, Space.World);
}
else
{
isReturn = true;
transform.Translate(-moveDistance * Time.deltaTime, 0, -moveDistance * Time.deltaTime, Space.World);
}
}
}
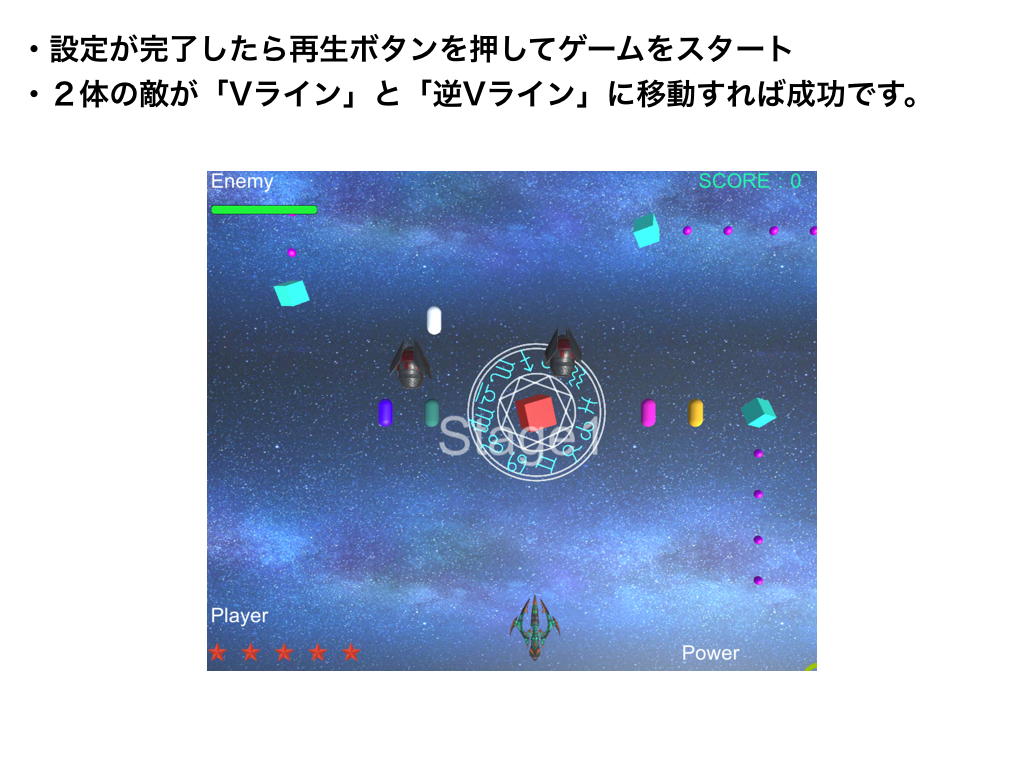
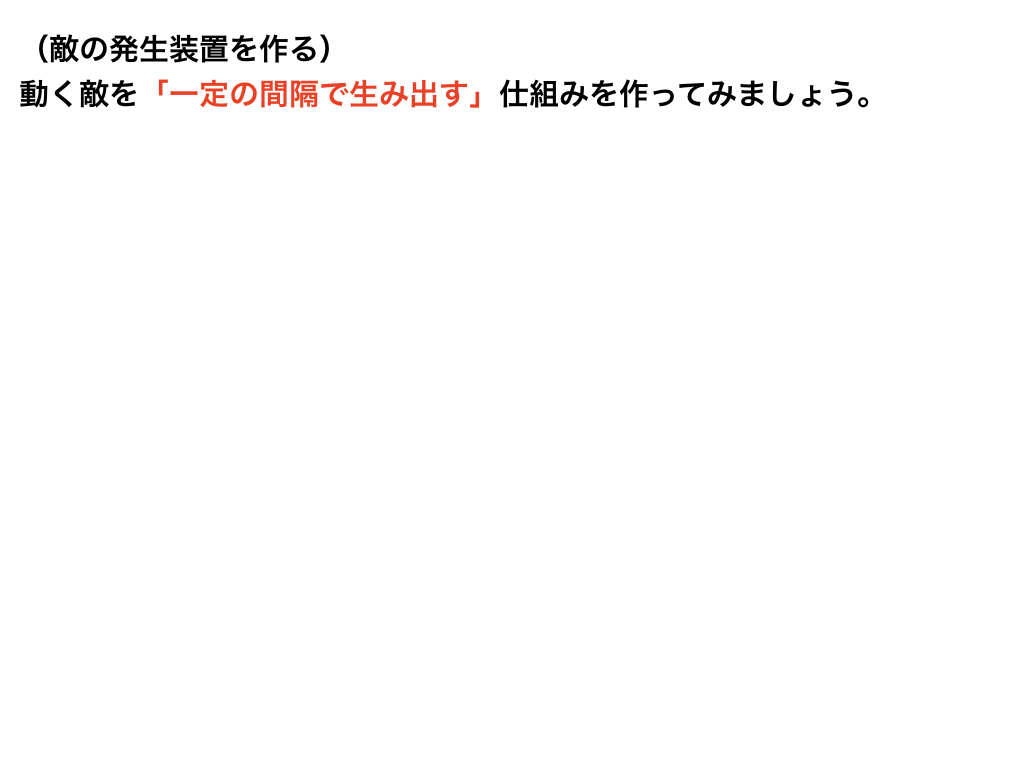
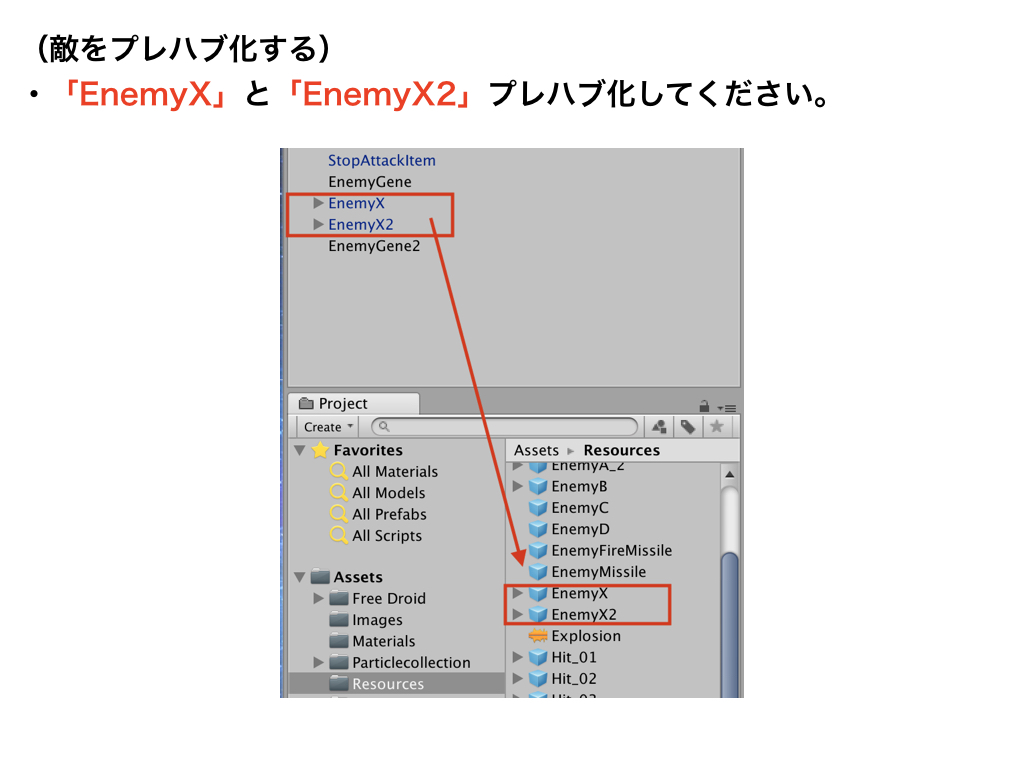
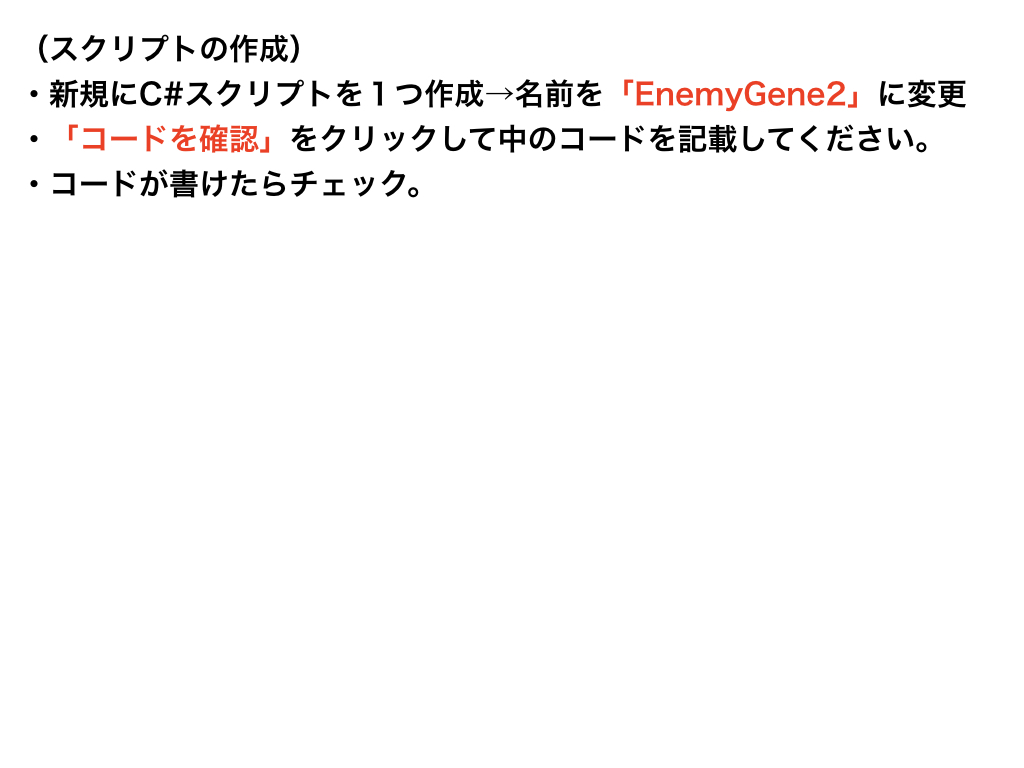
敵の発生装置
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class EnemyGene2 : MonoBehaviour
{
public GameObject enemyPrefab;
public int geneAmount;
[Range(0, 1.5f)]
public float waitTime;
private void Start()
{
StartCoroutine(GeneEnemy());
}
IEnumerator GeneEnemy()
{
for (int i = 0; i < geneAmount; i++)
{
GameObject enemy = Instantiate(enemyPrefab, transform.position, Quaternion.identity);
Destroy(enemy, 15.0f);
yield return new WaitForSeconds(waitTime);
}
}
}
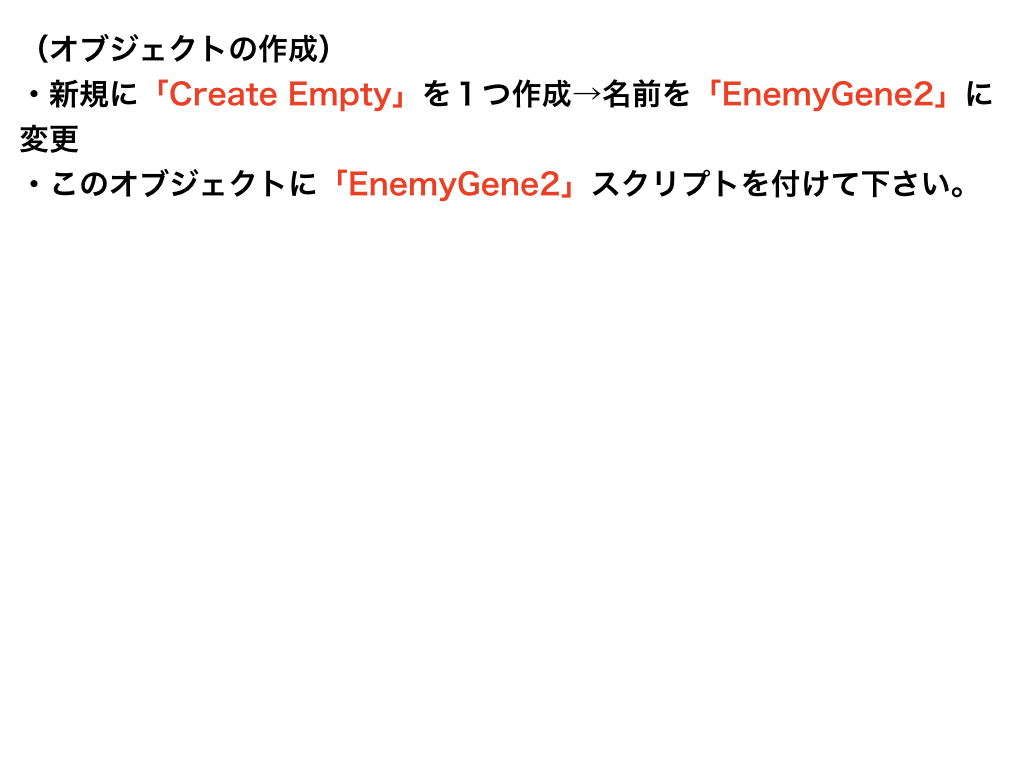
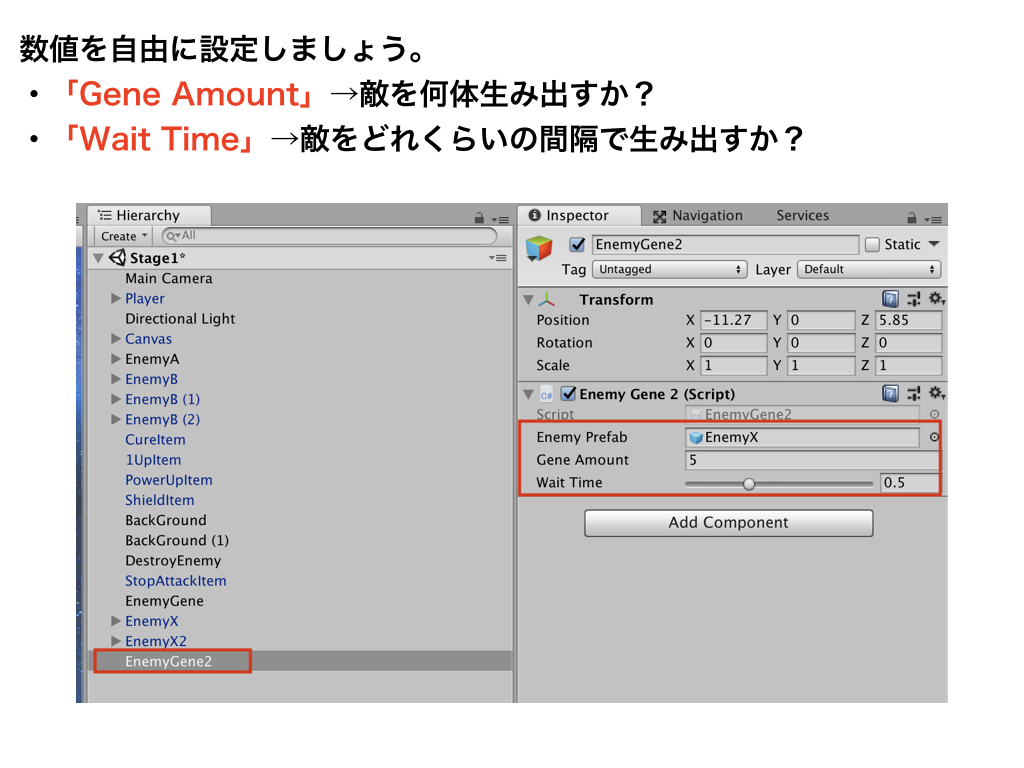
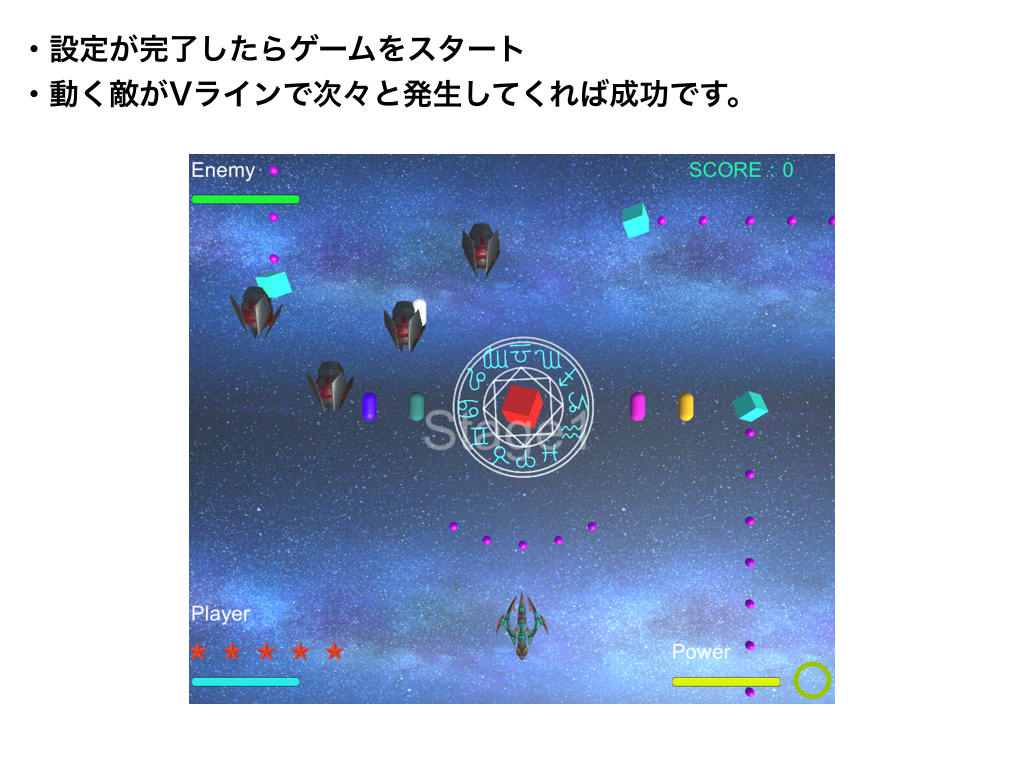
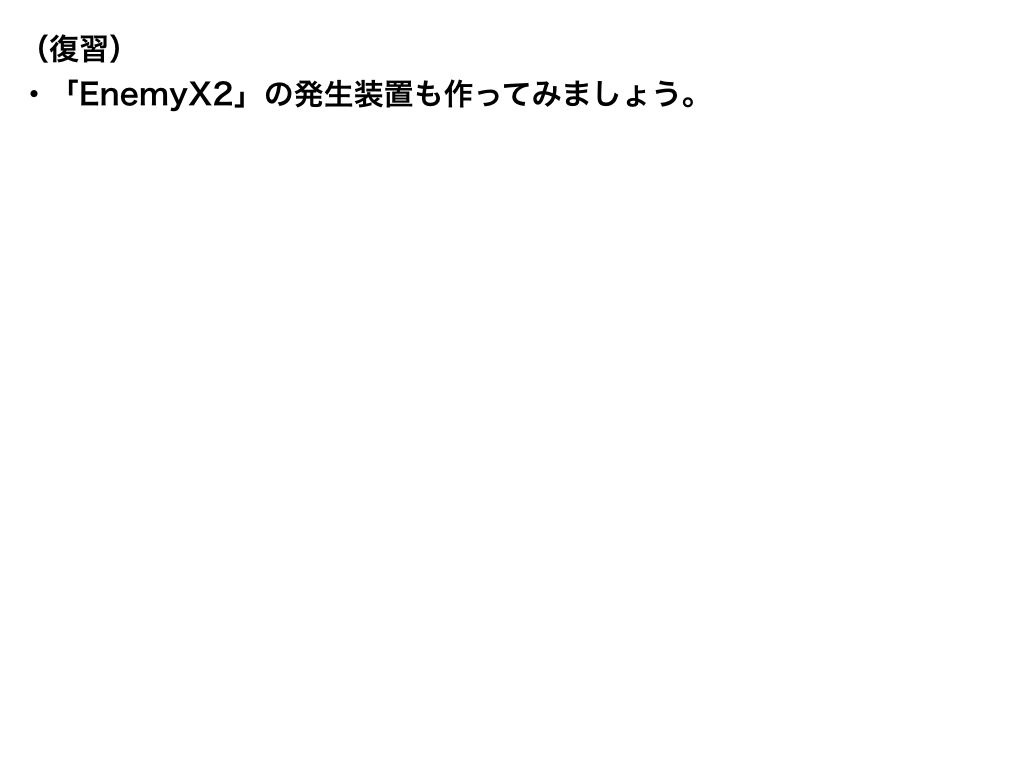
動く敵の作り方1(Vライン)