敵ロボットにHPをつける
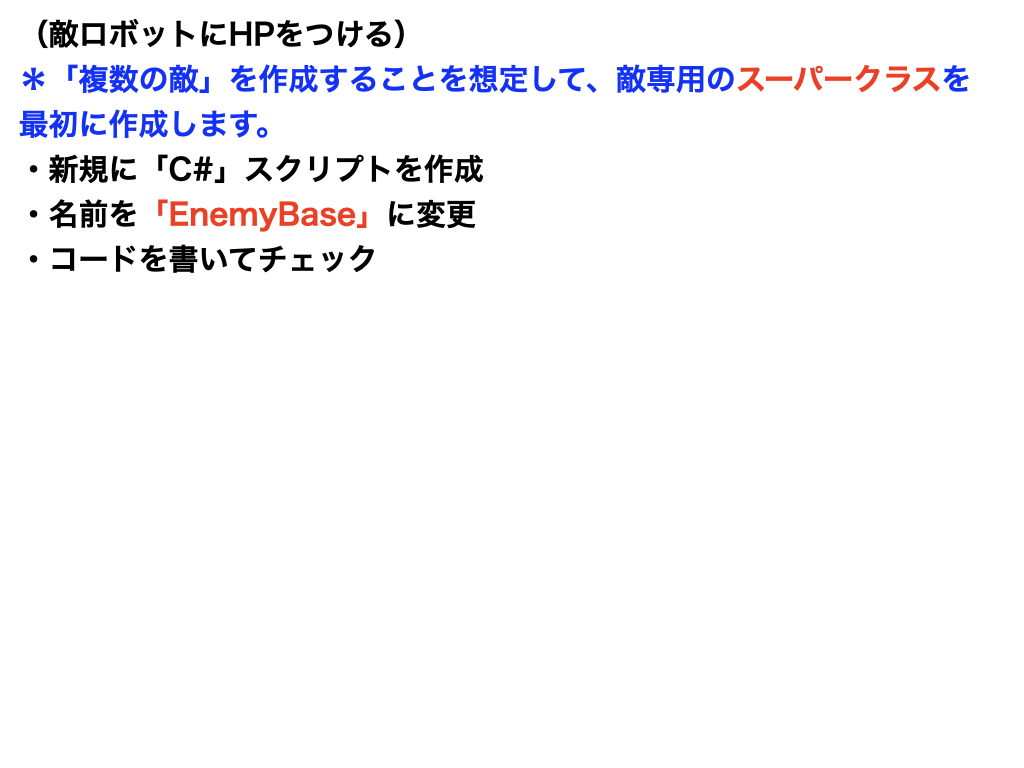
敵のスーパークラスの作成
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
// ★「abstract」キーワードを追加すると「抽象クラス」になる(ポイント)
public abstract class EnemyBase : MonoBehaviour
{
public int HP;
// ★「virtual」キーワードを追加すると、メソッドを「オーバーライド」できるようになる(ポイント)
public virtual void TakeDamage(int attackPower)
{
HP -= attackPower;
if(HP < 1)
{
Destroy(gameObject);
}
}
}
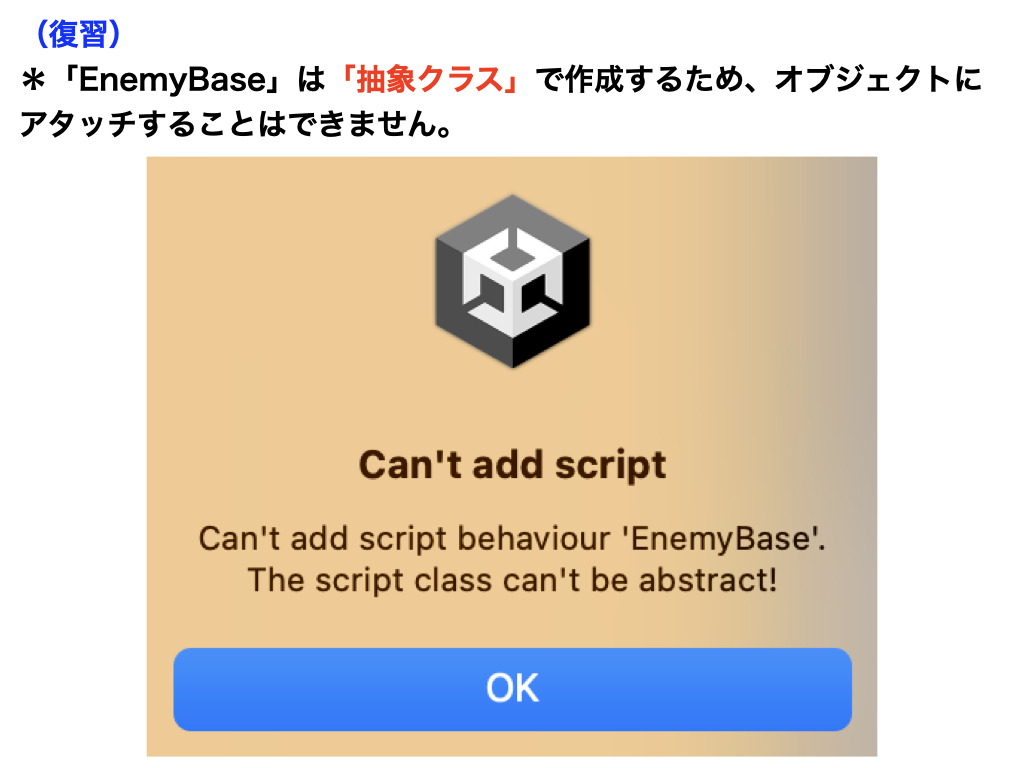
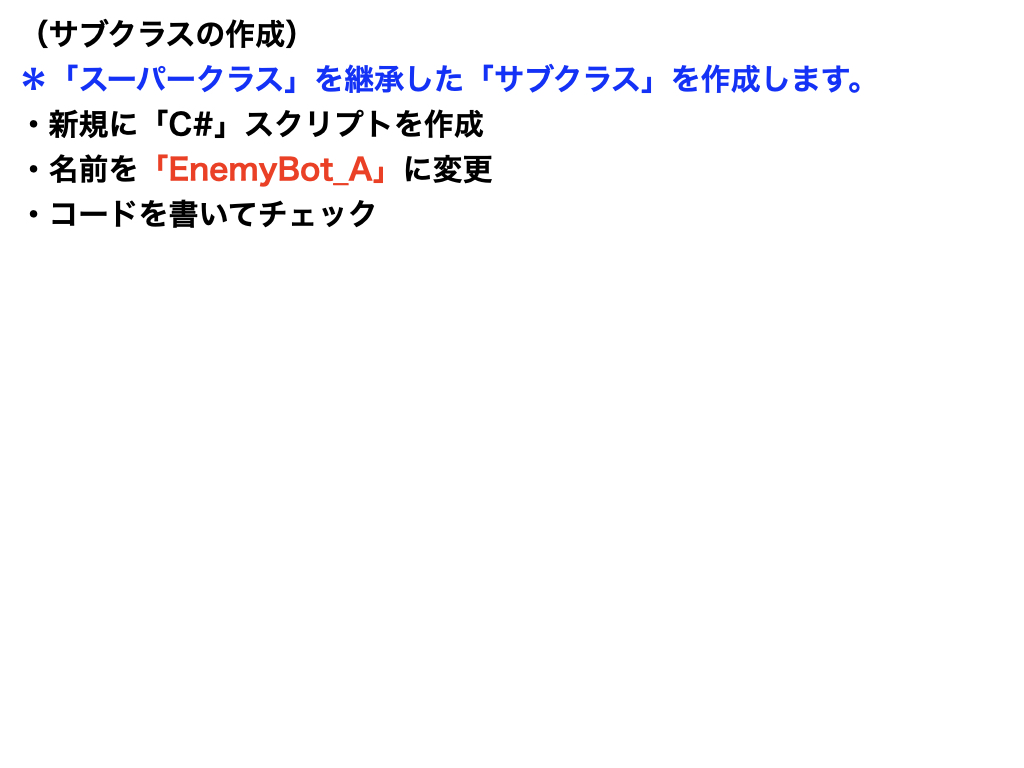
敵のサブクラスの作成
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class EnemyBot_A : EnemyBase // 変更(ポイント)
{
private void Start()
{
// EnemyBaseを「継承」しているので、そこで定義されている「HP」変数を使うことができる。
HP = 1;
}
}
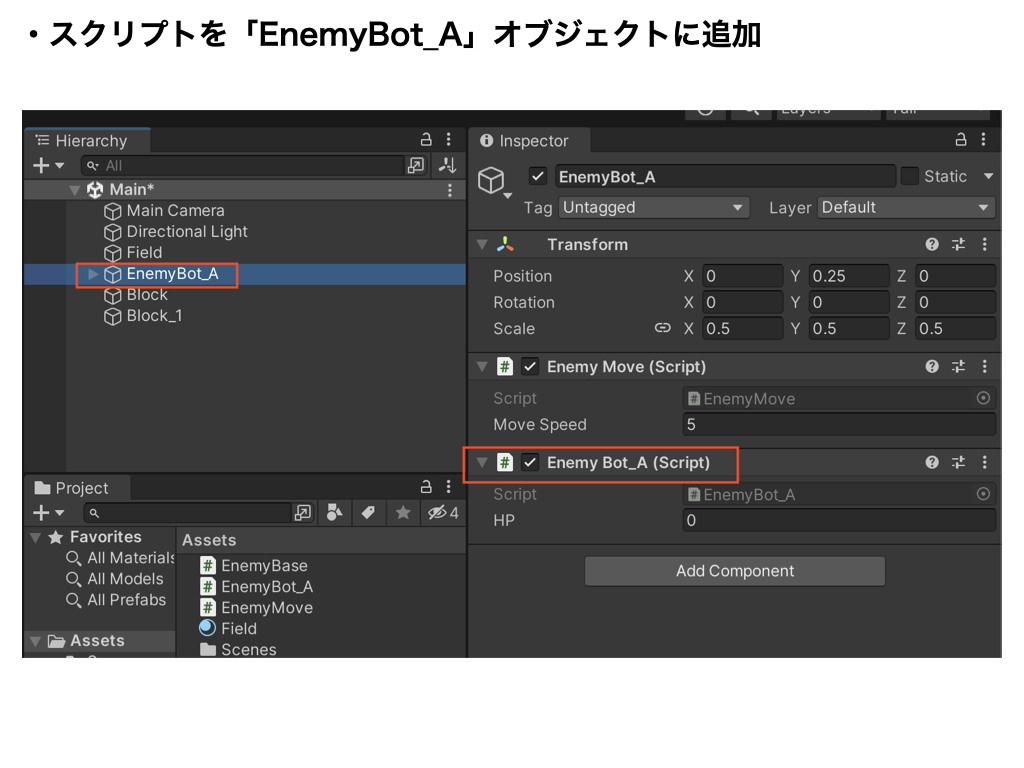
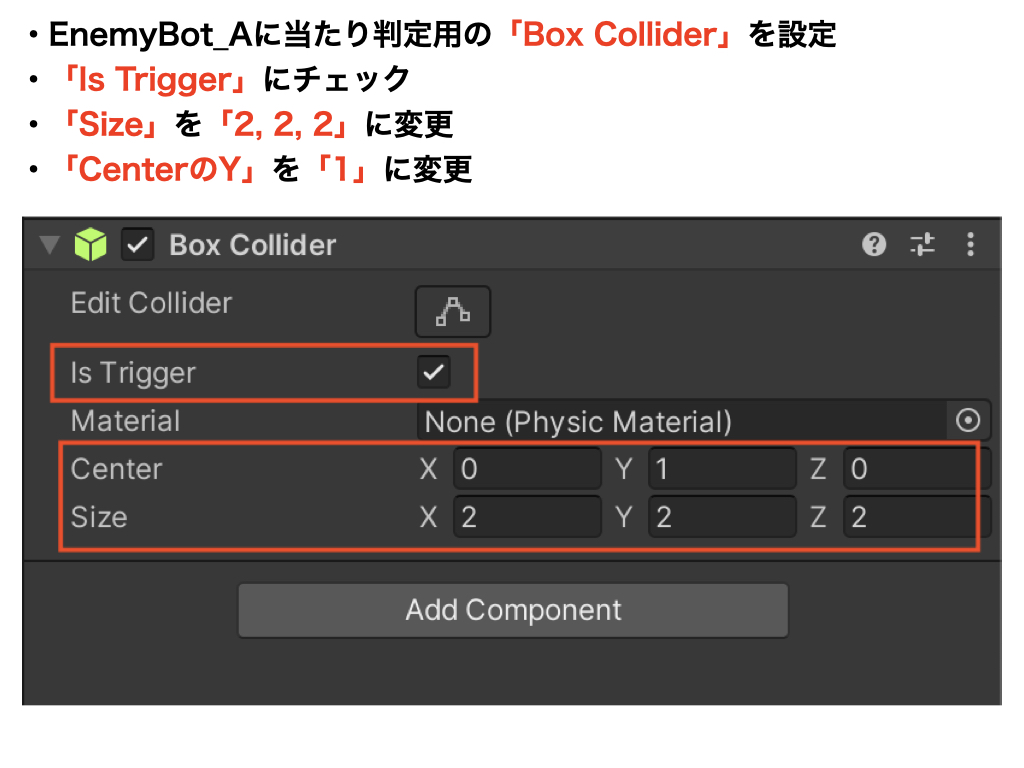
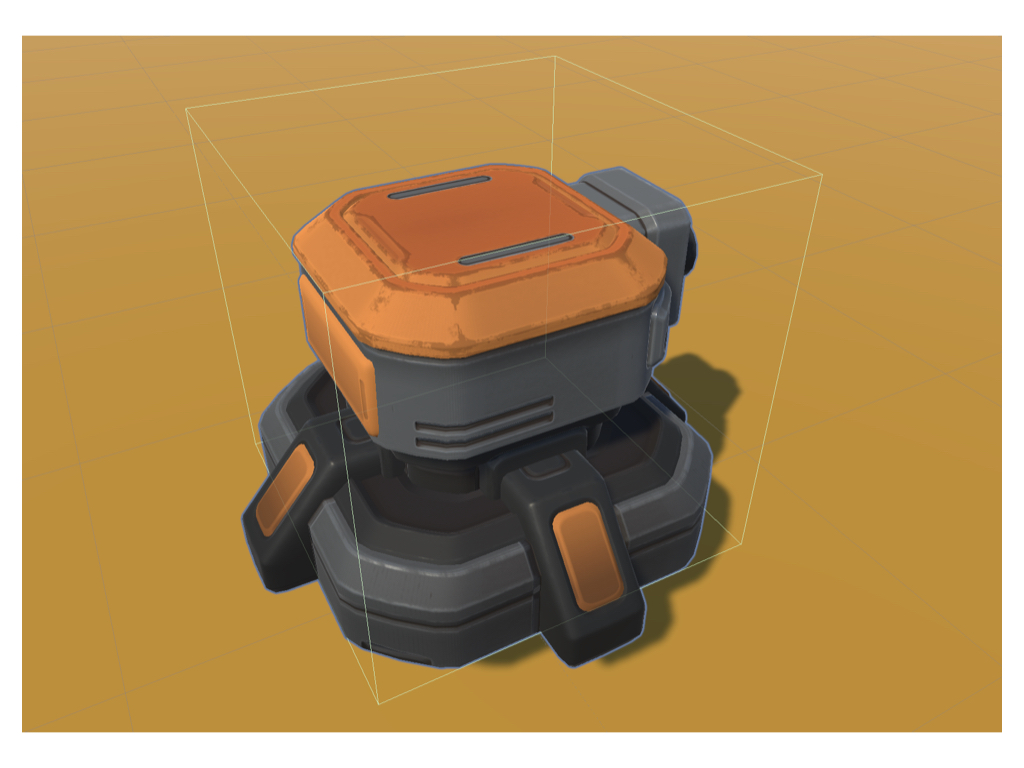
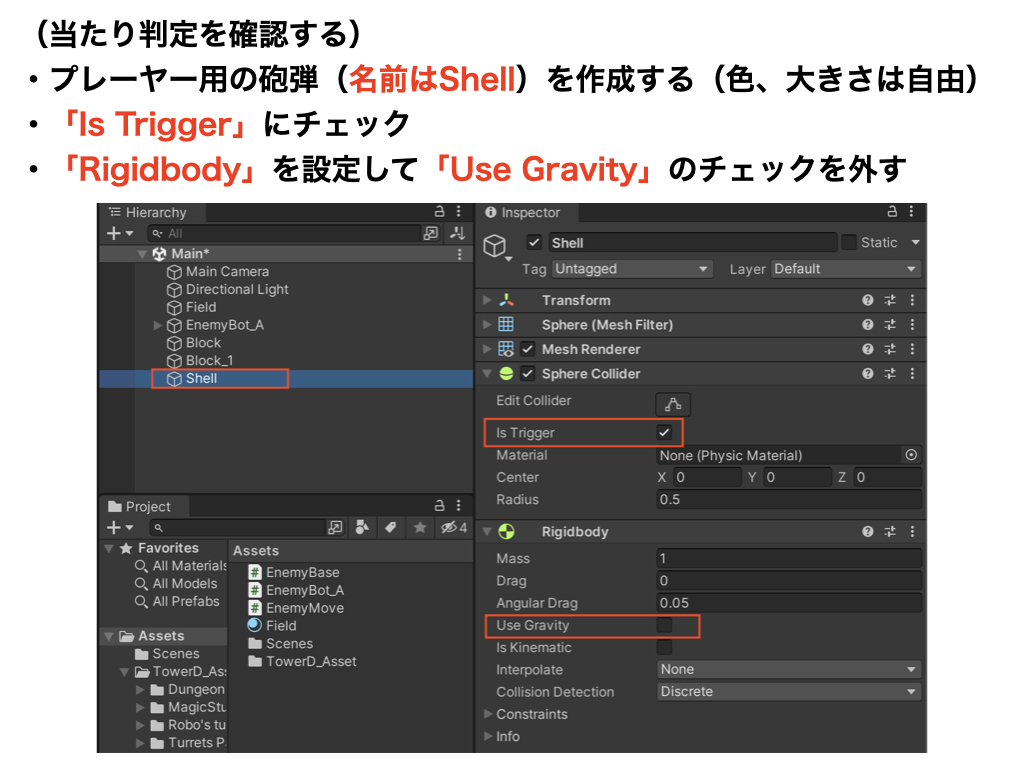
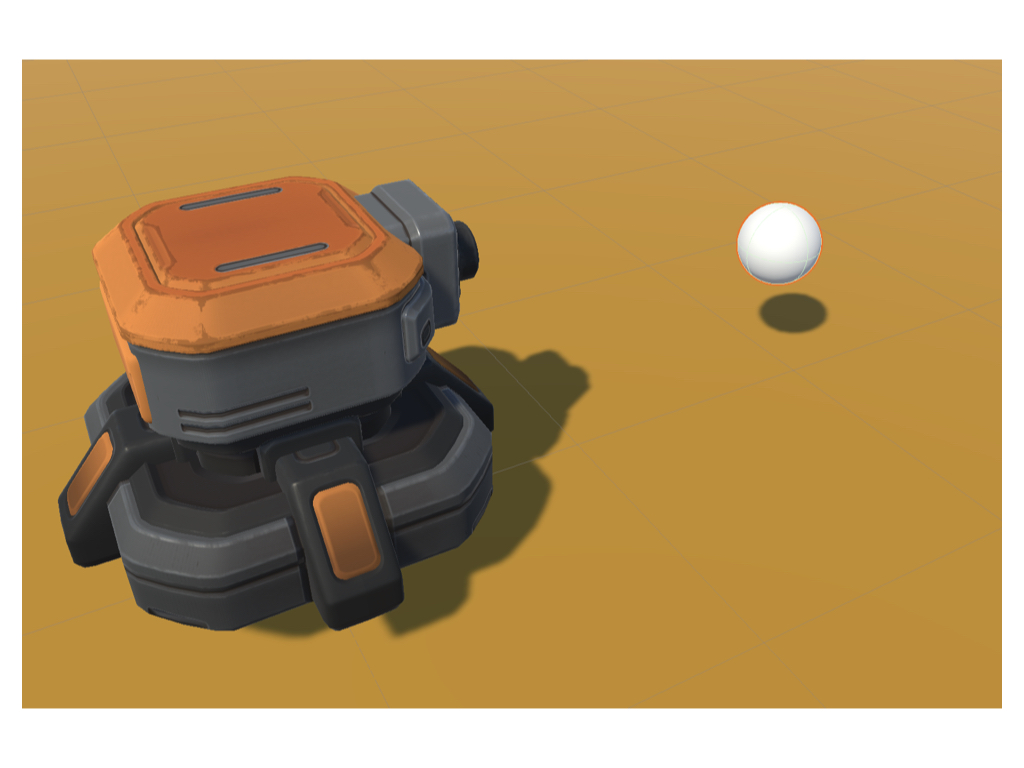
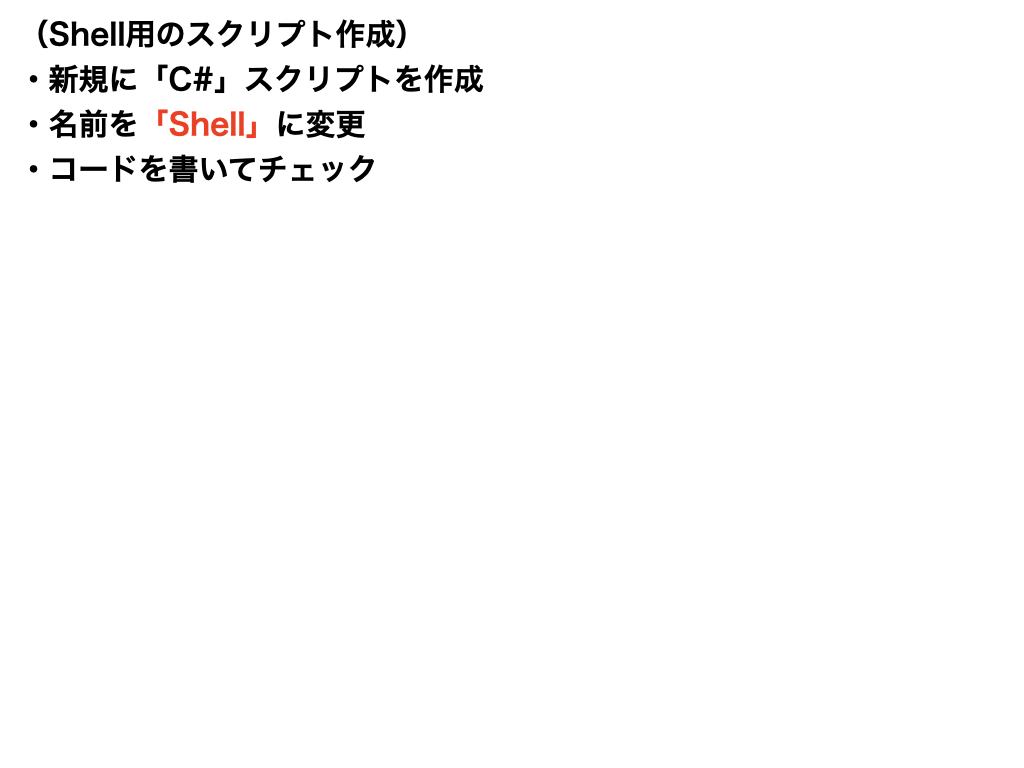
敵にダメージを与える
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Shell : MonoBehaviour
{
public GameObject effectPrefab;
public AudioClip sound;
private void OnTriggerEnter(Collider other)
{
// ★テクニック
// TryGetComponentはoutの後ろで指定したコンポーネント(スクリプト)を持っているか否かを判定できる。
// 「クラス継承」の場合、「スーパークラス」まで辿ることができる。
// (条件)もしも、砲弾にぶつかった相手方がEnemyBaseを持っているならば・・・
if (other.TryGetComponent(out EnemyBase enemy))
{
// TakeDamage()メソッドを呼び出す。
// 「1」はこの砲弾の攻撃力
enemy.TakeDamage(1);
// 砲弾を破壊する。
Destroy(gameObject);
GameObject effect = Instantiate(effectPrefab, transform.position, Quaternion.identity);
Destroy(effect, 1.0f);
AudioSource.PlayClipAtPoint(sound, transform.position);
}
}
}
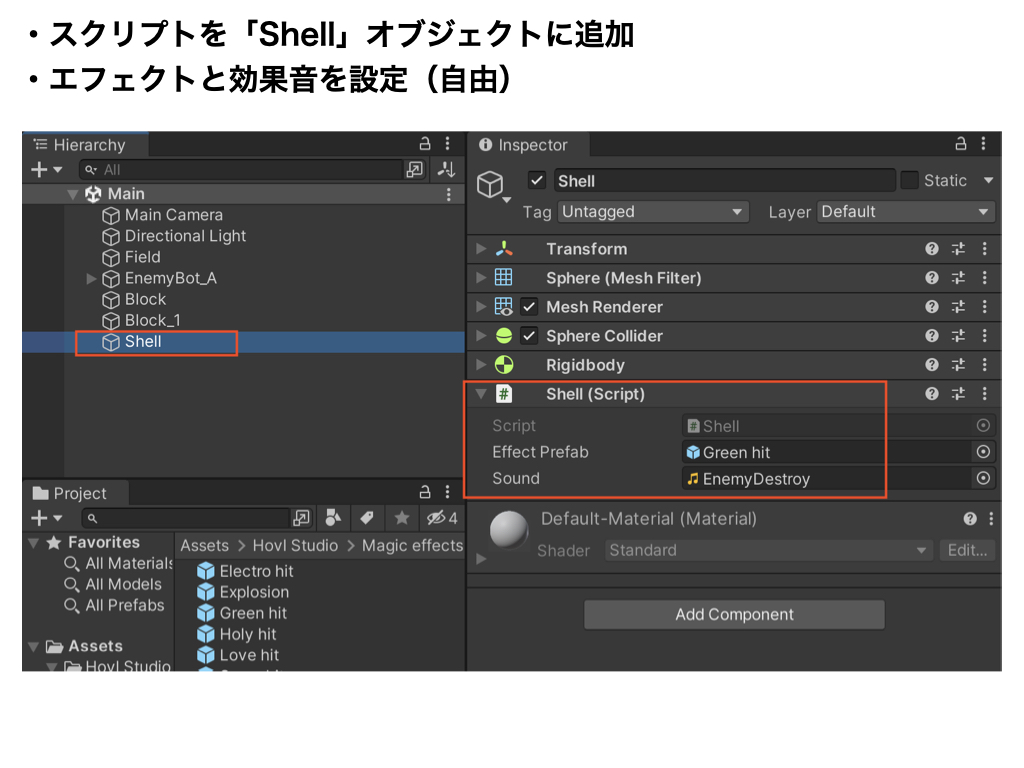
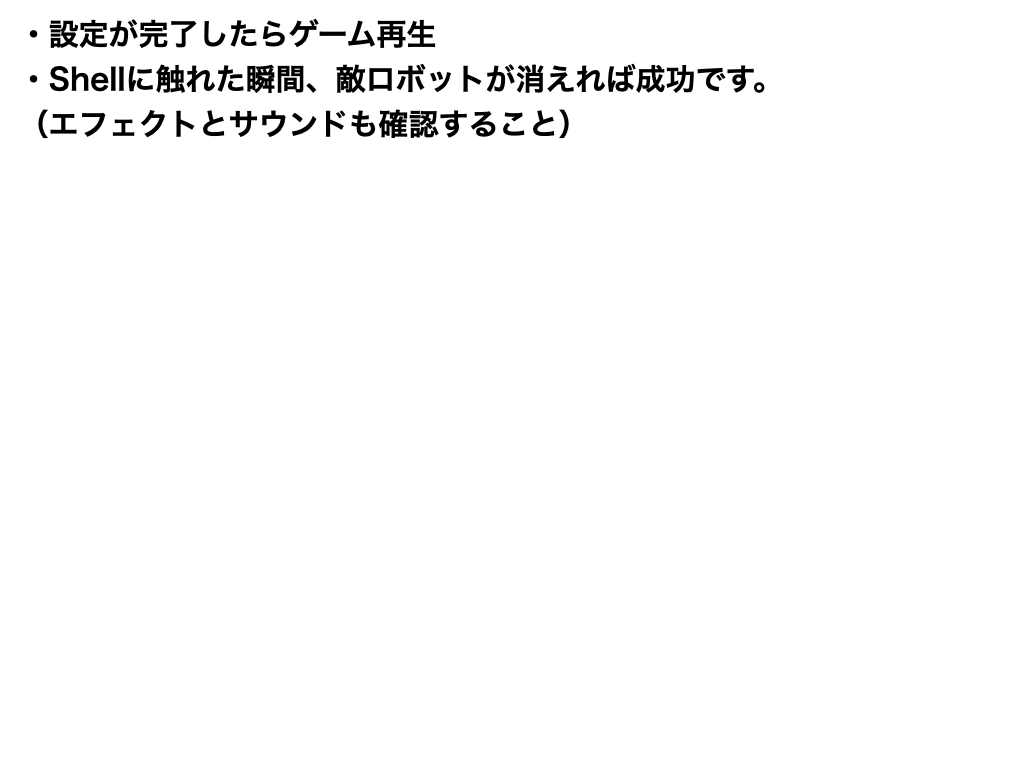
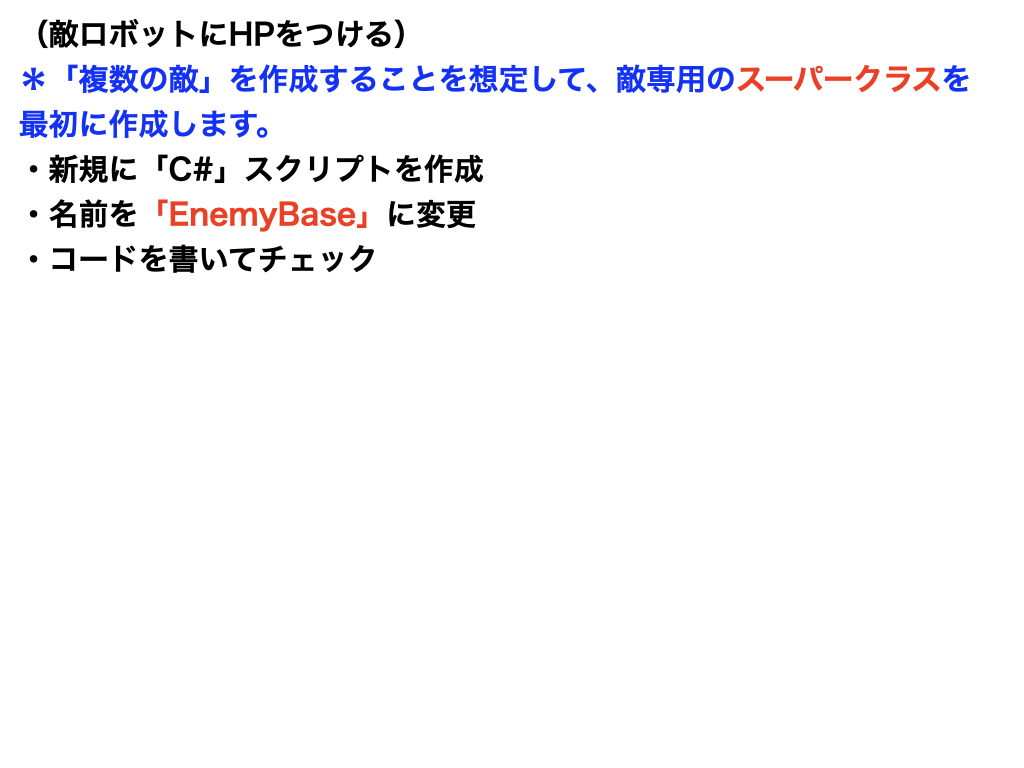
敵のスーパークラスの作成
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
// ★「abstract」キーワードを追加すると「抽象クラス」になる(ポイント)
public abstract class EnemyBase : MonoBehaviour
{
public int HP;
// ★「virtual」キーワードを追加すると、メソッドを「オーバーライド」できるようになる(ポイント)
public virtual void TakeDamage(int attackPower)
{
HP -= attackPower;
if(HP < 1)
{
Destroy(gameObject);
}
}
}
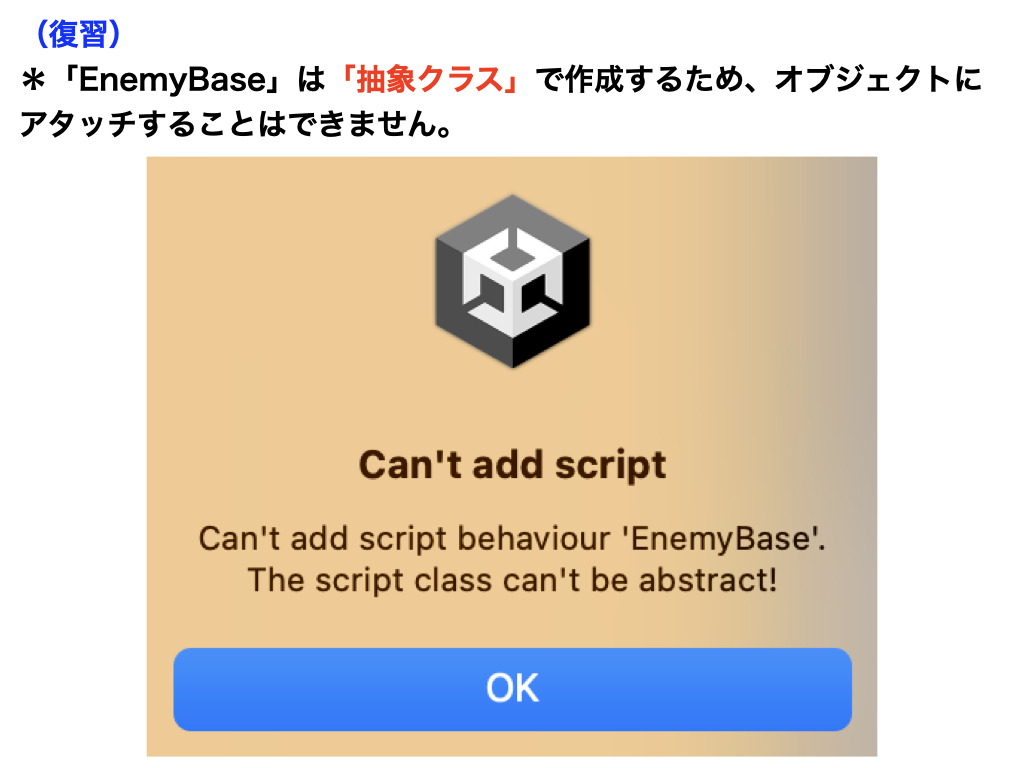
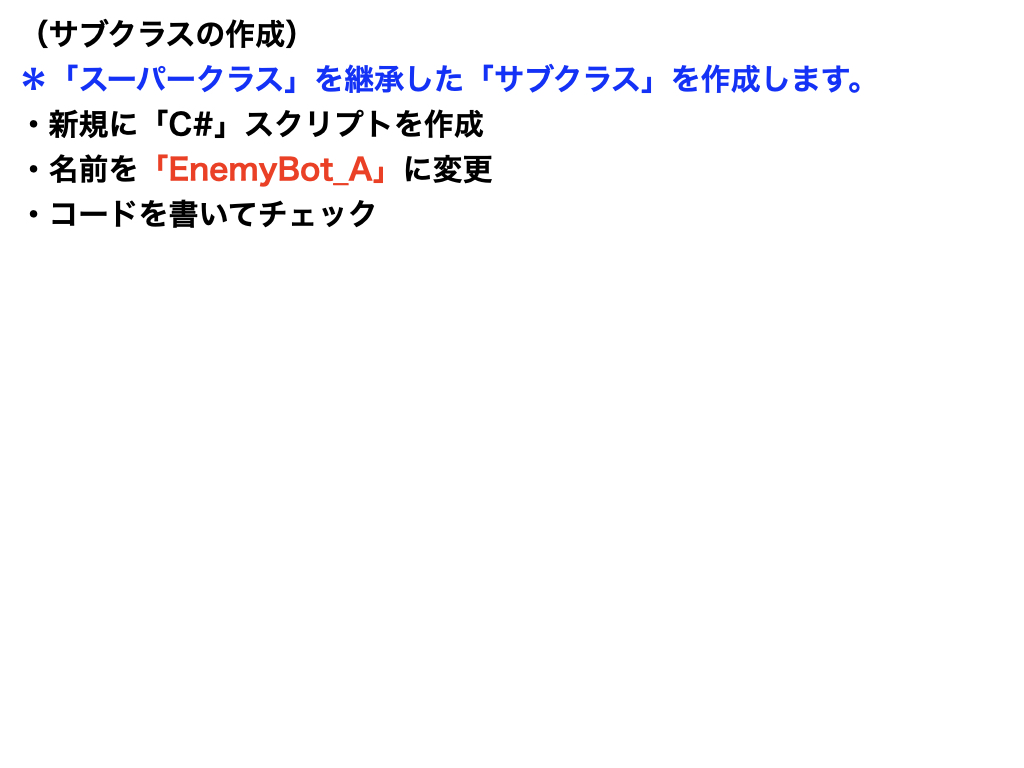
敵のサブクラスの作成
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class EnemyBot_A : EnemyBase // 変更(ポイント)
{
private void Start()
{
// EnemyBaseを「継承」しているので、そこで定義されている「HP」変数を使うことができる。
HP = 1;
}
}
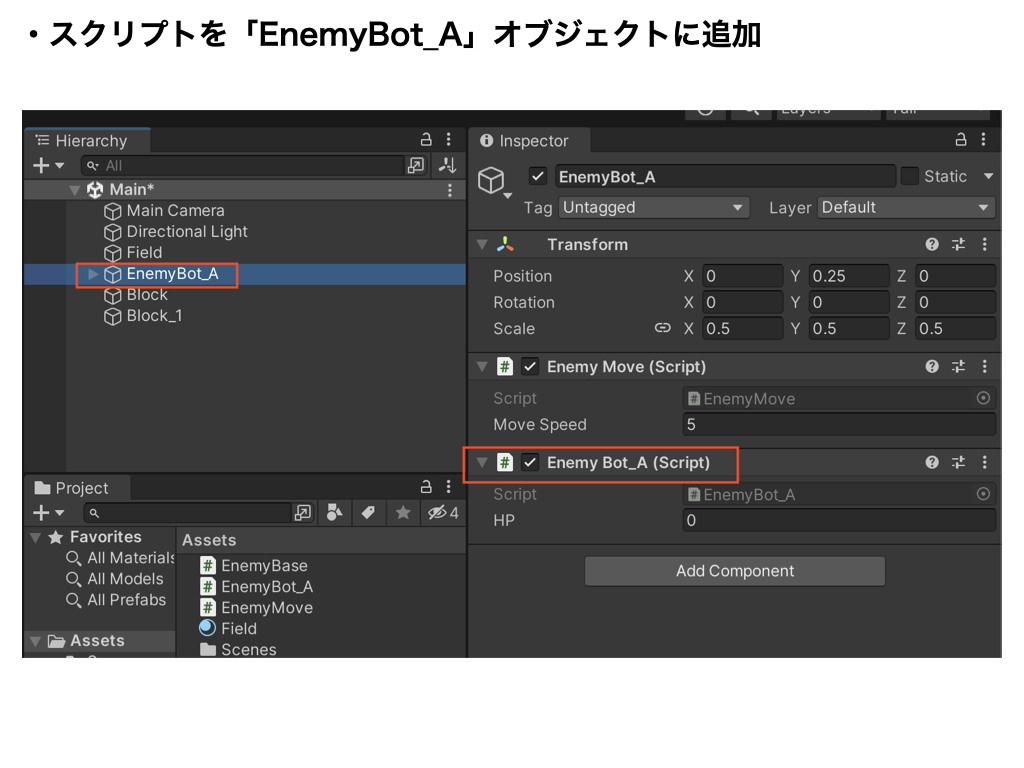
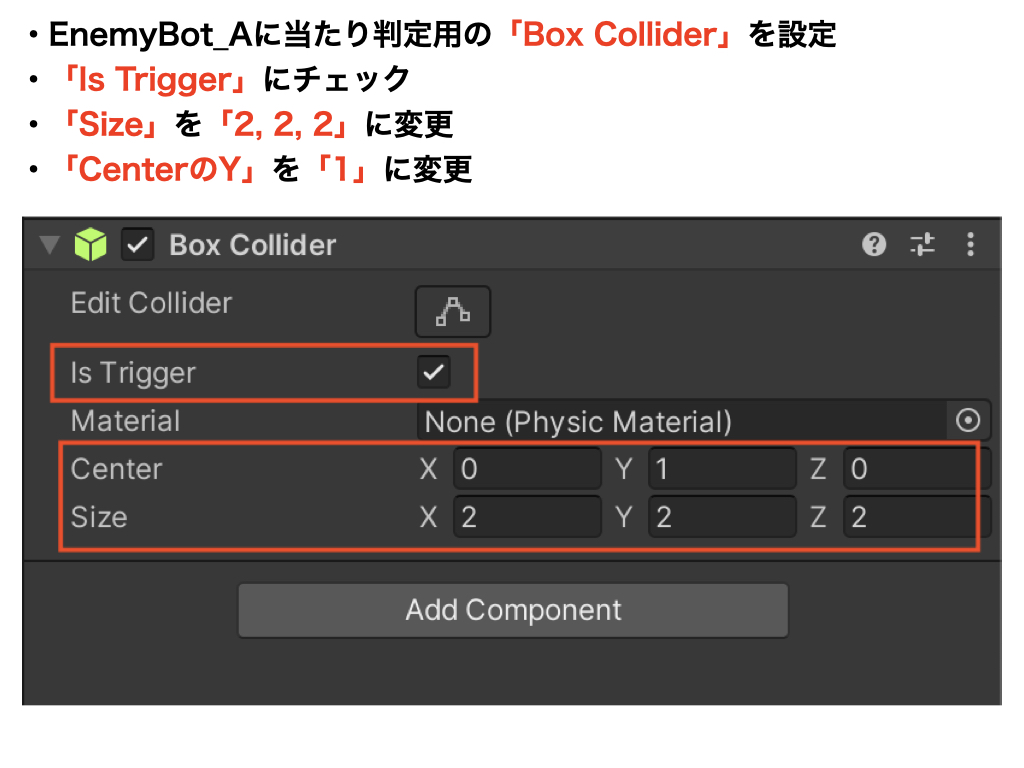
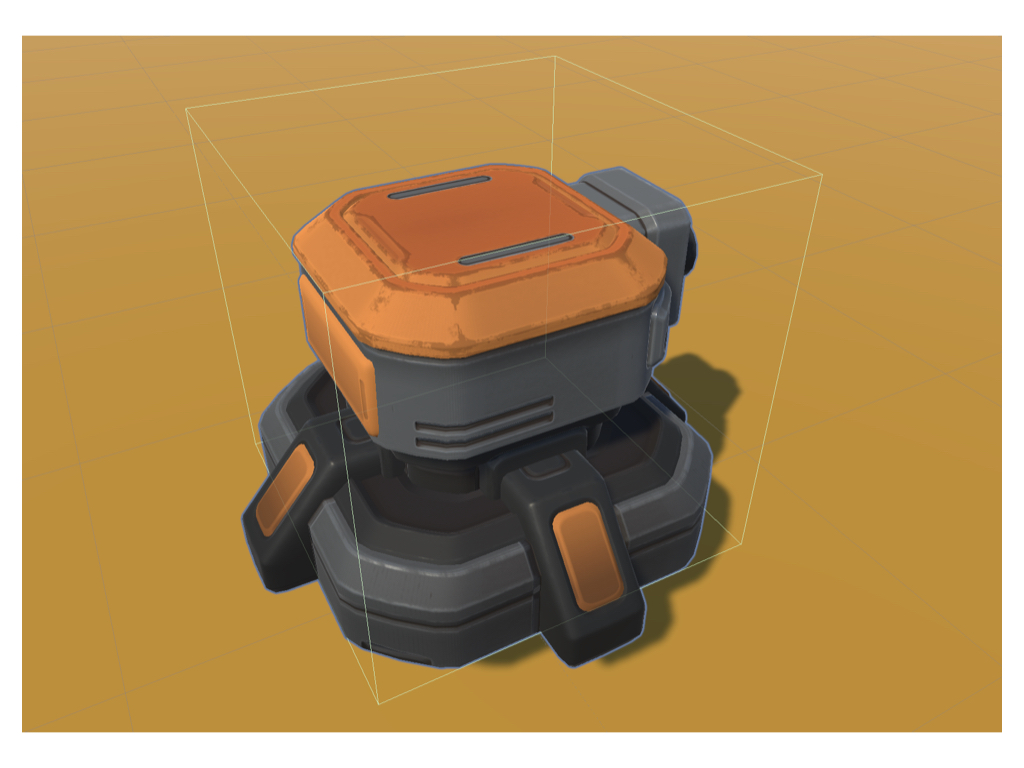
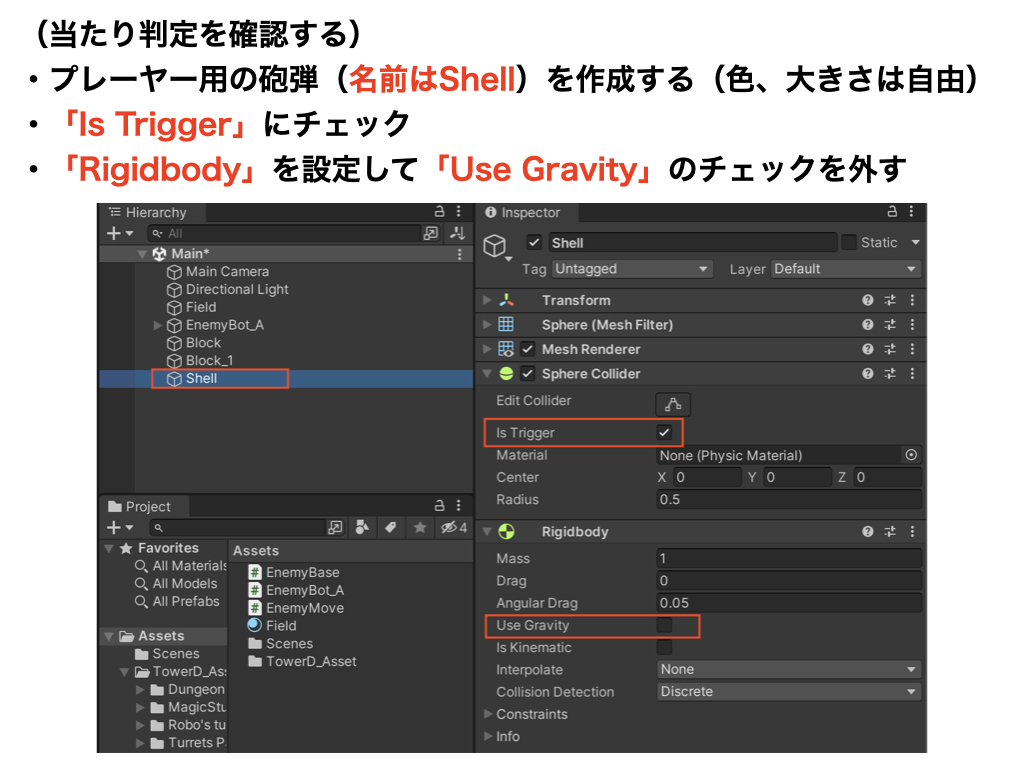
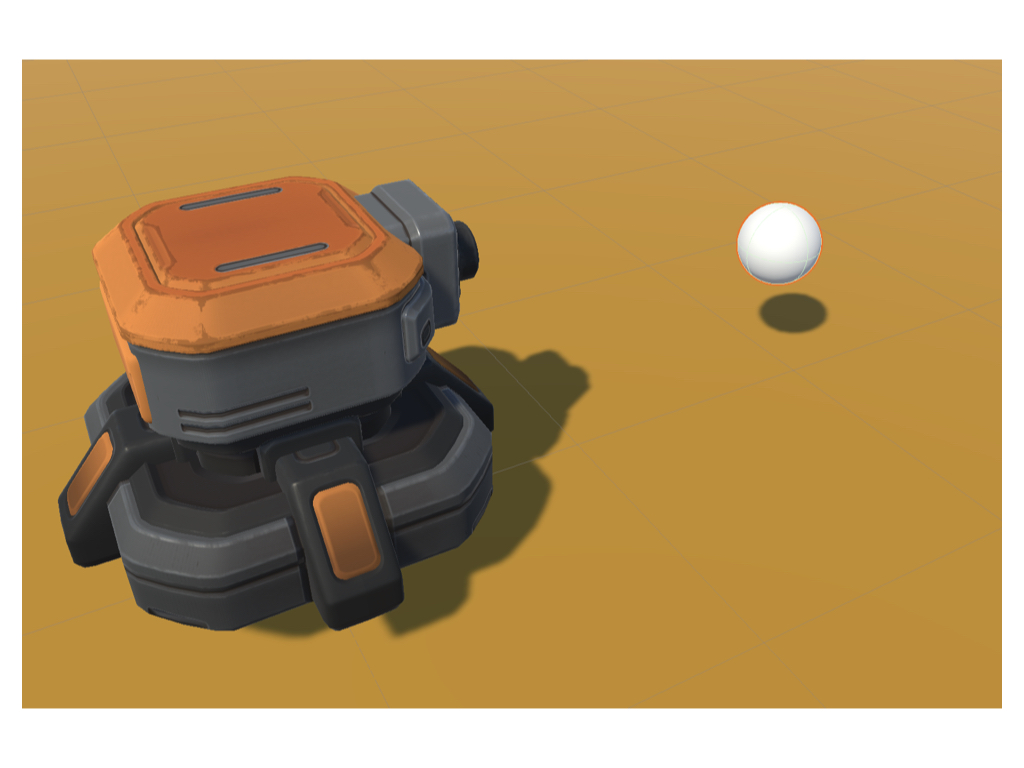
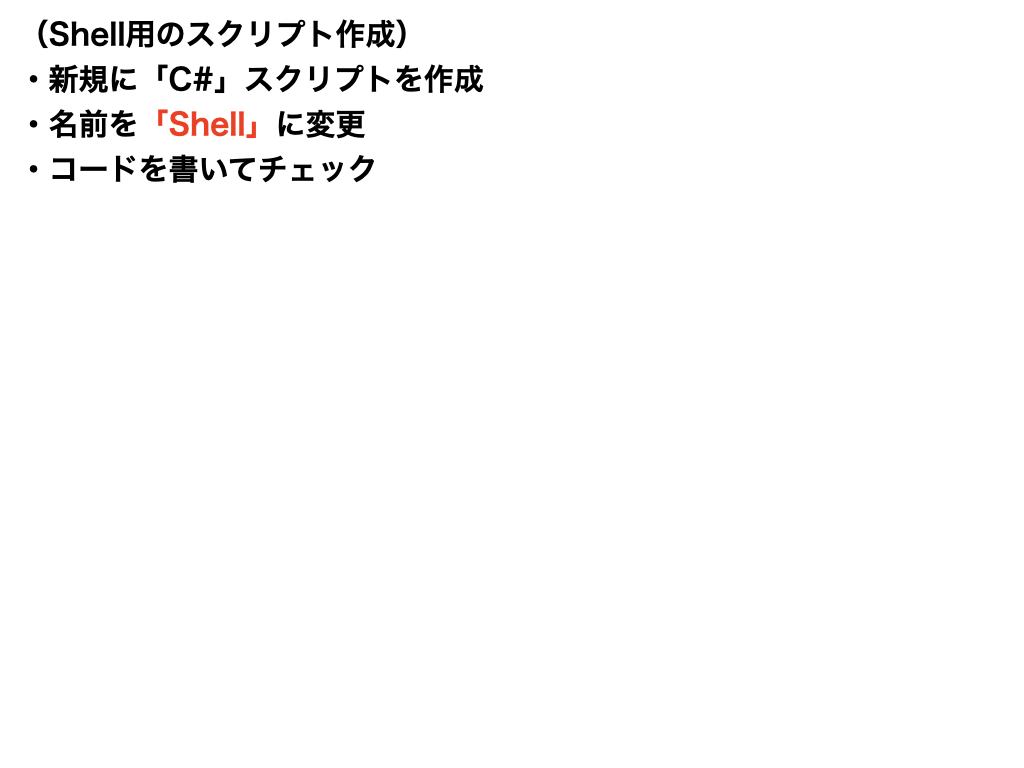
敵にダメージを与える
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Shell : MonoBehaviour
{
public GameObject effectPrefab;
public AudioClip sound;
private void OnTriggerEnter(Collider other)
{
// ★テクニック
// TryGetComponentはoutの後ろで指定したコンポーネント(スクリプト)を持っているか否かを判定できる。
// 「クラス継承」の場合、「スーパークラス」まで辿ることができる。
// (条件)もしも、砲弾にぶつかった相手方がEnemyBaseを持っているならば・・・
if (other.TryGetComponent(out EnemyBase enemy))
{
// TakeDamage()メソッドを呼び出す。
// 「1」はこの砲弾の攻撃力
enemy.TakeDamage(1);
// 砲弾を破壊する。
Destroy(gameObject);
GameObject effect = Instantiate(effectPrefab, transform.position, Quaternion.identity);
Destroy(effect, 1.0f);
AudioSource.PlayClipAtPoint(sound, transform.position);
}
}
}
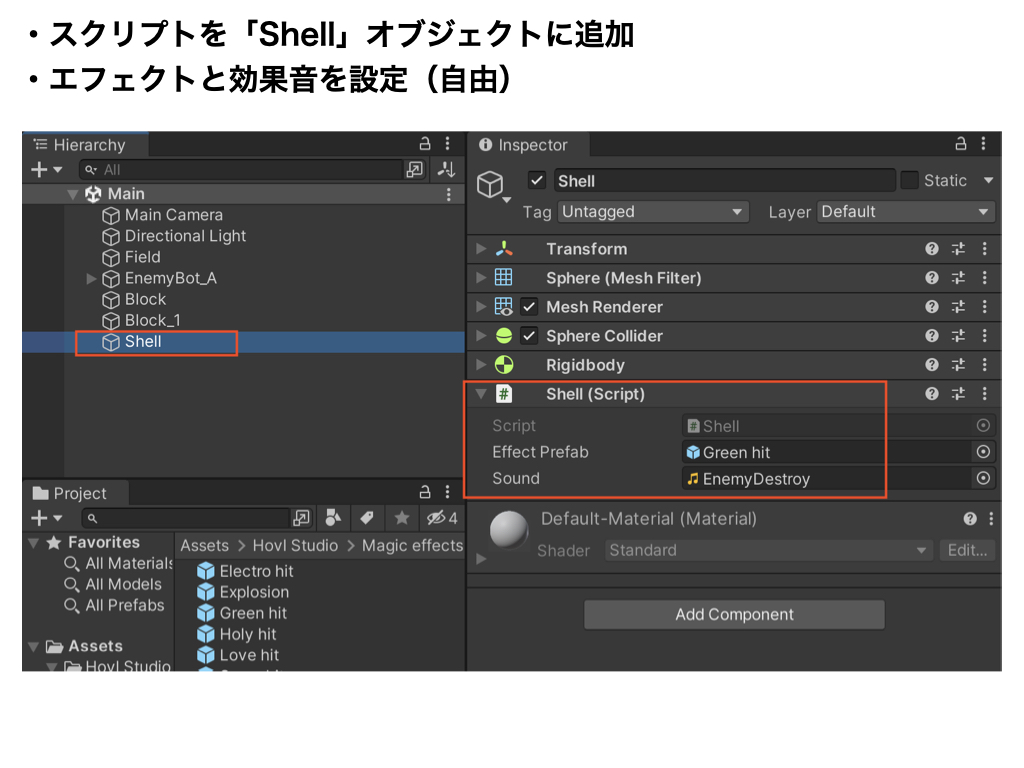
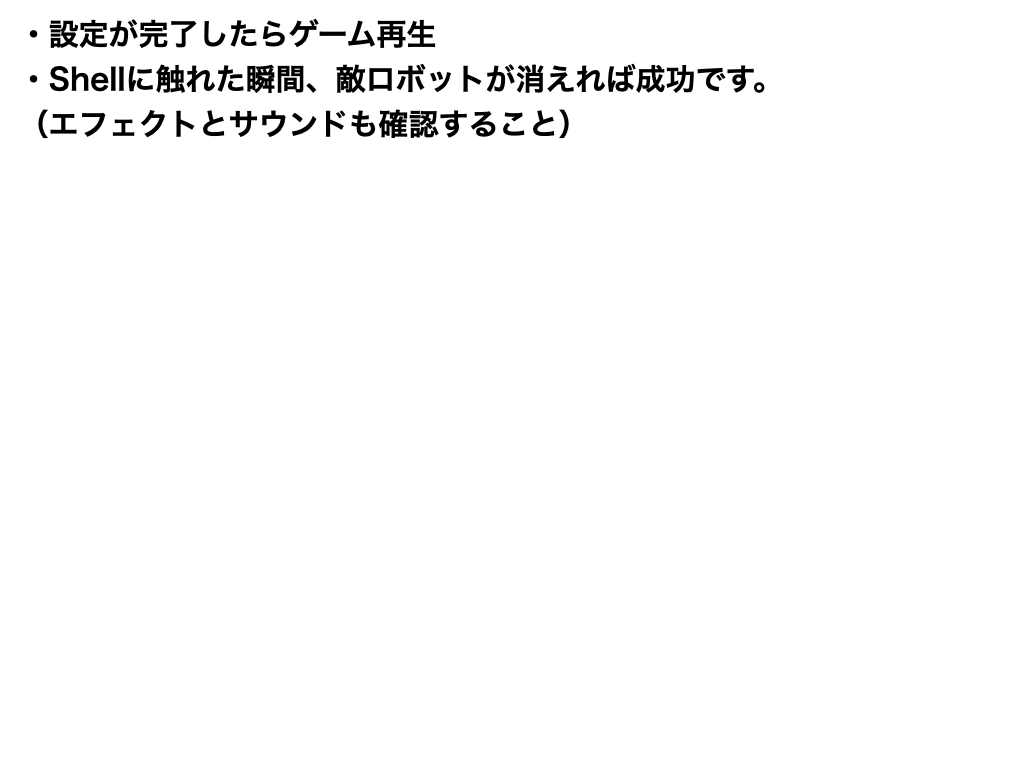
敵ロボットにHPをつける