ボールを動かす方法を変える
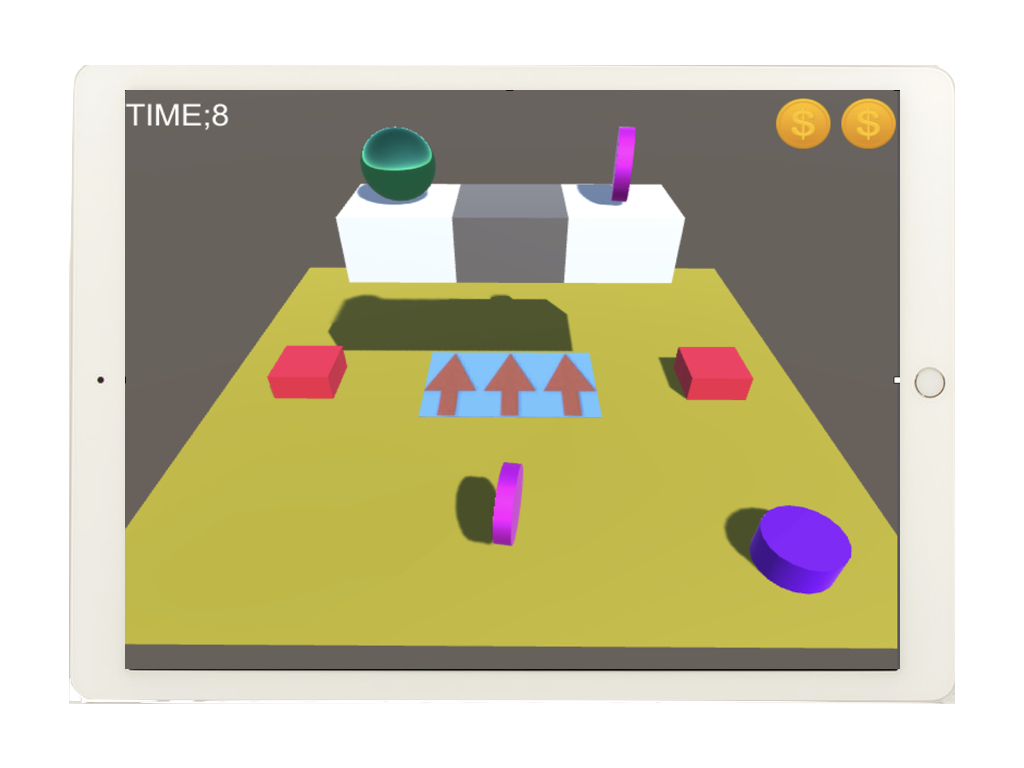
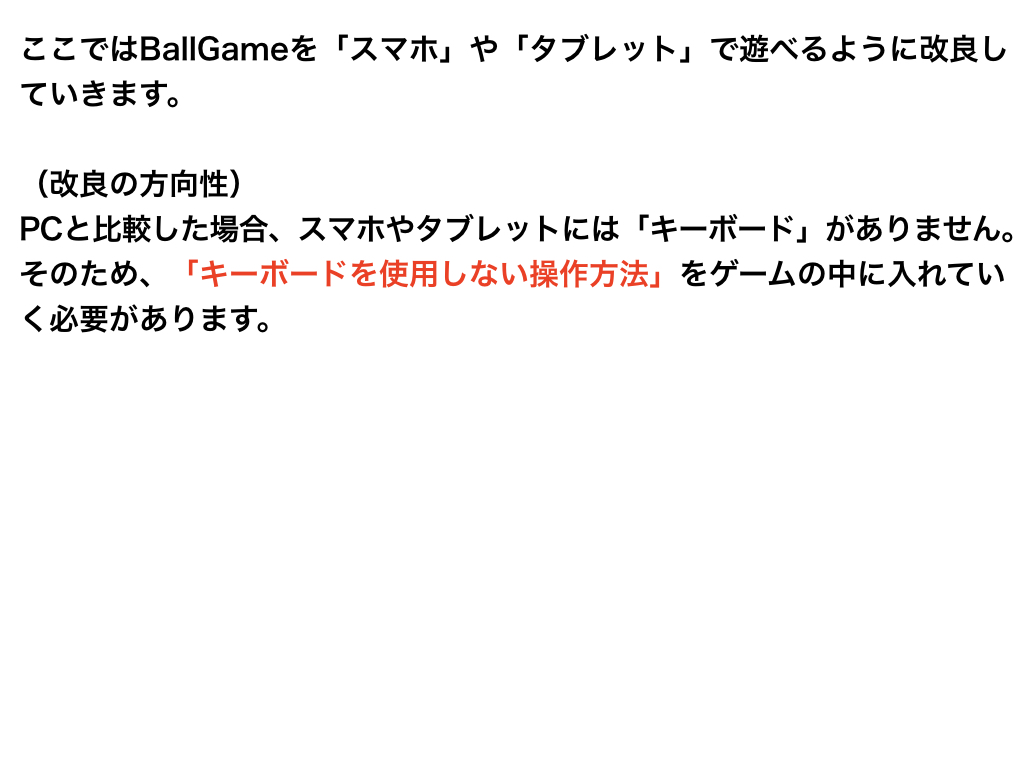
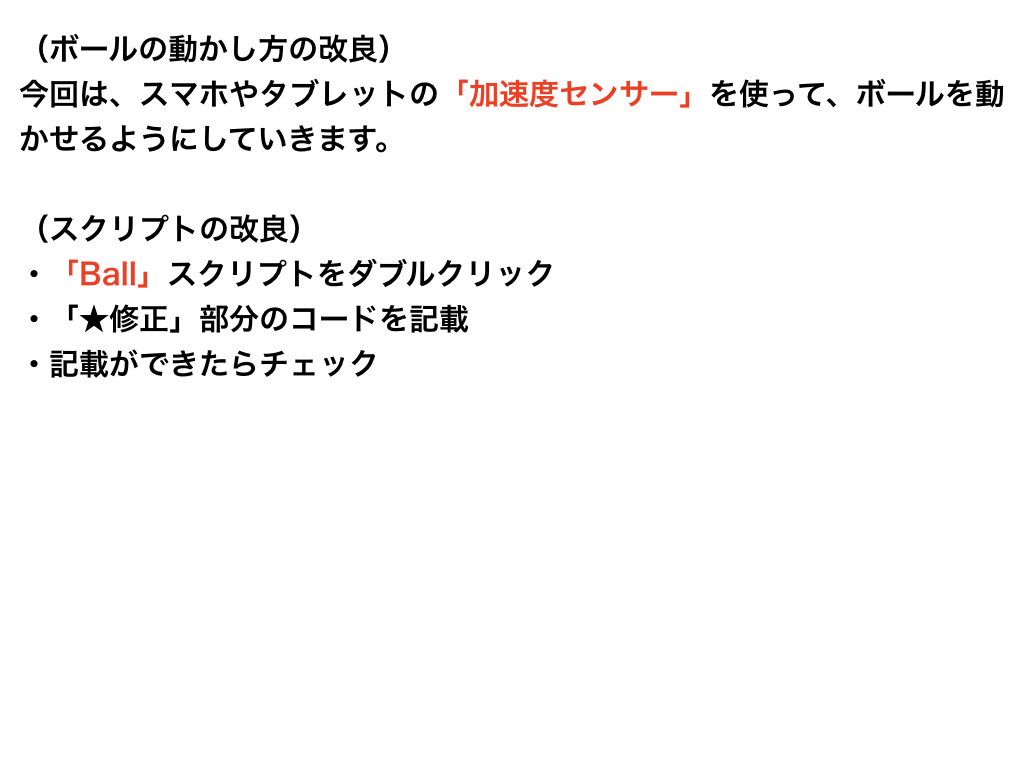
加速度センサーでボールを動かす
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
public class Ball : MonoBehaviour
{
public float moveSpeed;
private Rigidbody rb;
public AudioClip coinGet;
public float jumpSpeed;
private bool isJumping = false;
private int coinCount = 0;
public GameObject target;
void Start()
{
rb = GetComponent<Rigidbody>();
}
void Update()
{
// ★ボールの操作方法の修正
if (Application.isEditor)
{
float moveH = Input.GetAxis("Horizontal");
float moveV = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(moveH, 0, moveV);
rb.AddForce(movement * moveSpeed);
}
else
{
// スマホやタブレットの加速度センサーを活用する。
float moveH = Input.acceleration.x;
float moveV = Input.acceleration.y; // ここがポイント!
Vector3 movement = new Vector3(moveH, 0, moveV);
rb.AddForce(movement * moveSpeed);
}
if (Input.GetButtonDown("Jump") && isJumping == false)
{
rb.velocity = Vector3.up * jumpSpeed;
isJumping = true;
}
}
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("Coin"))
{
Destroy(other.gameObject);
AudioSource.PlayClipAtPoint(coinGet, transform.position);
coinCount += 1;
if (coinCount == 1)
{
target.SetActive(true);
}
if (coinCount == 2)
{
SceneManager.LoadScene("GameClear");
}
}
}
public int Coin()
{
return coinCount;
}
private void OnCollisionEnter(Collision other)
{
if (other.gameObject.CompareTag("Floor"))
{
isJumping = false;
}
}
}
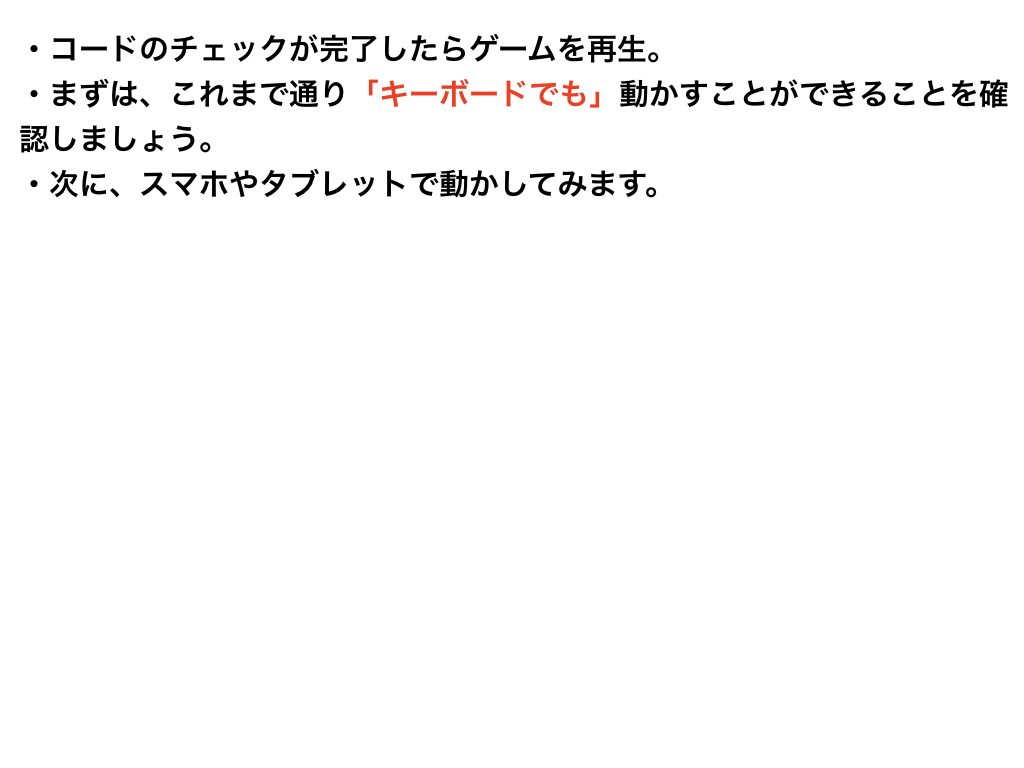
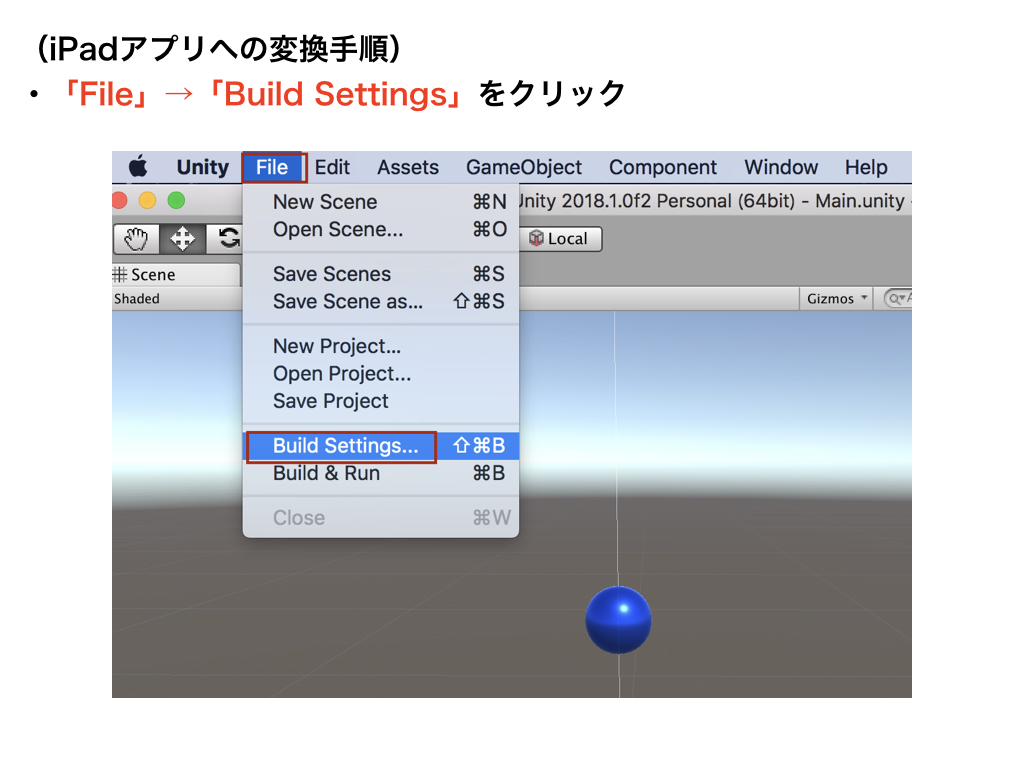
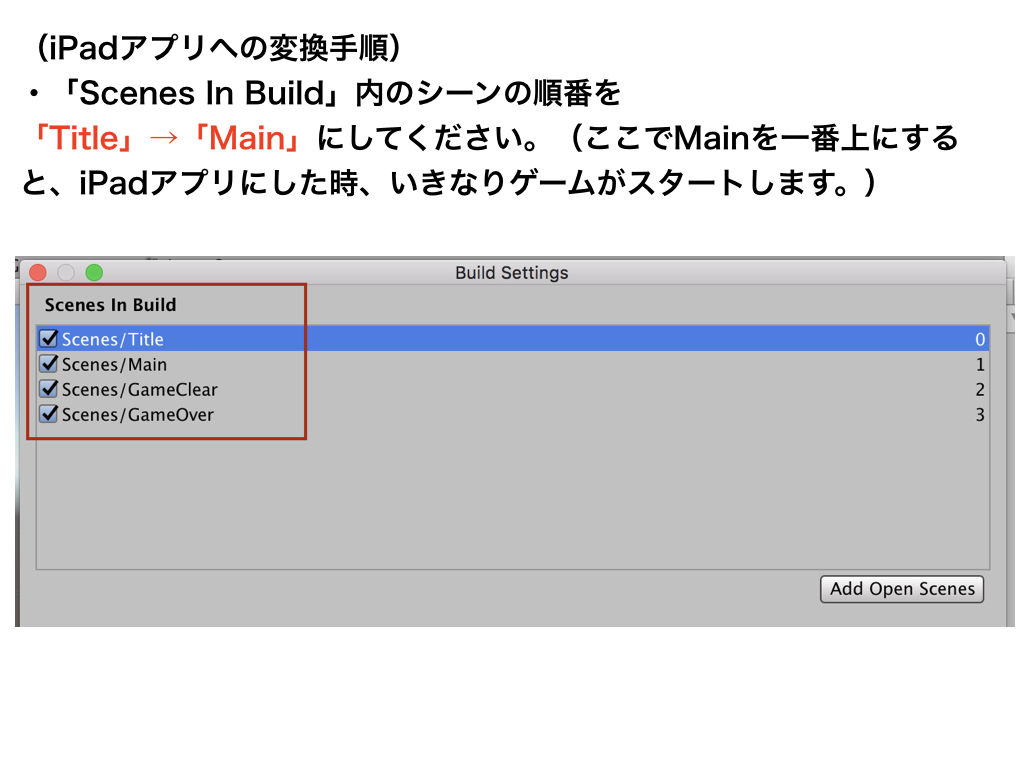
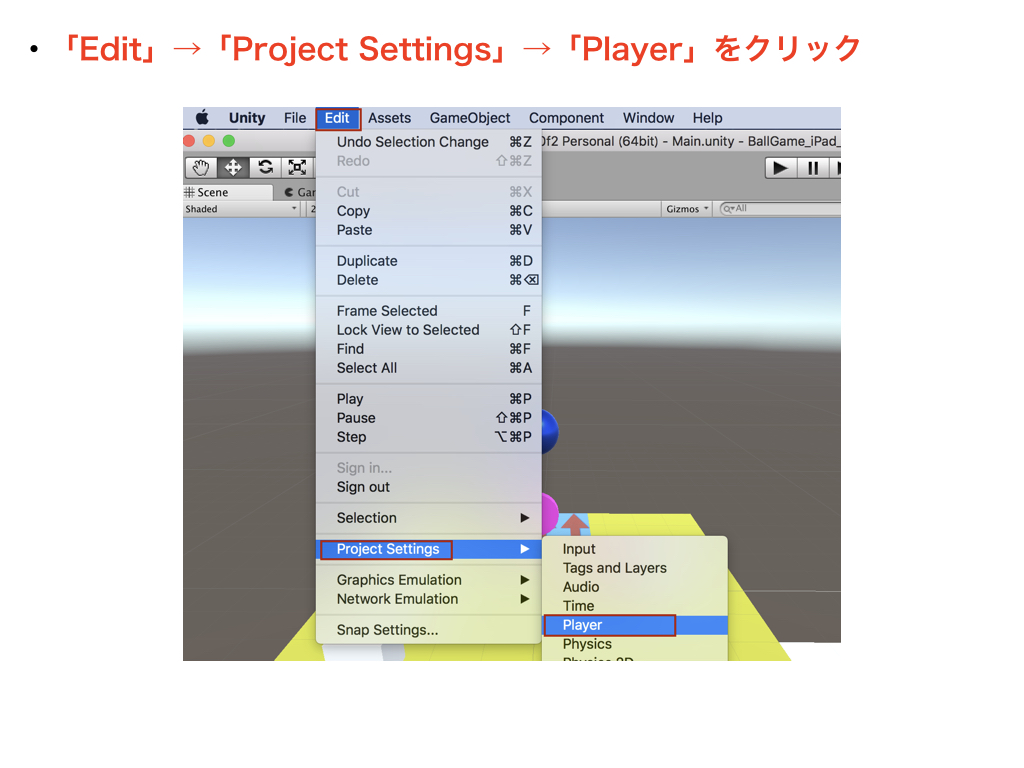
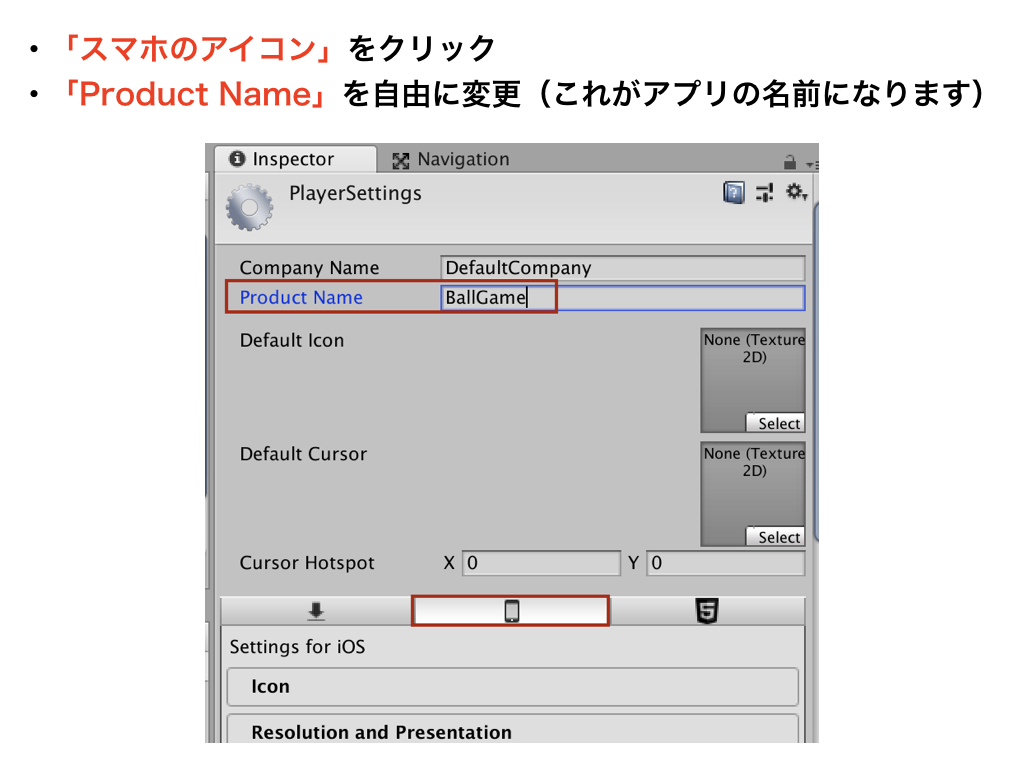
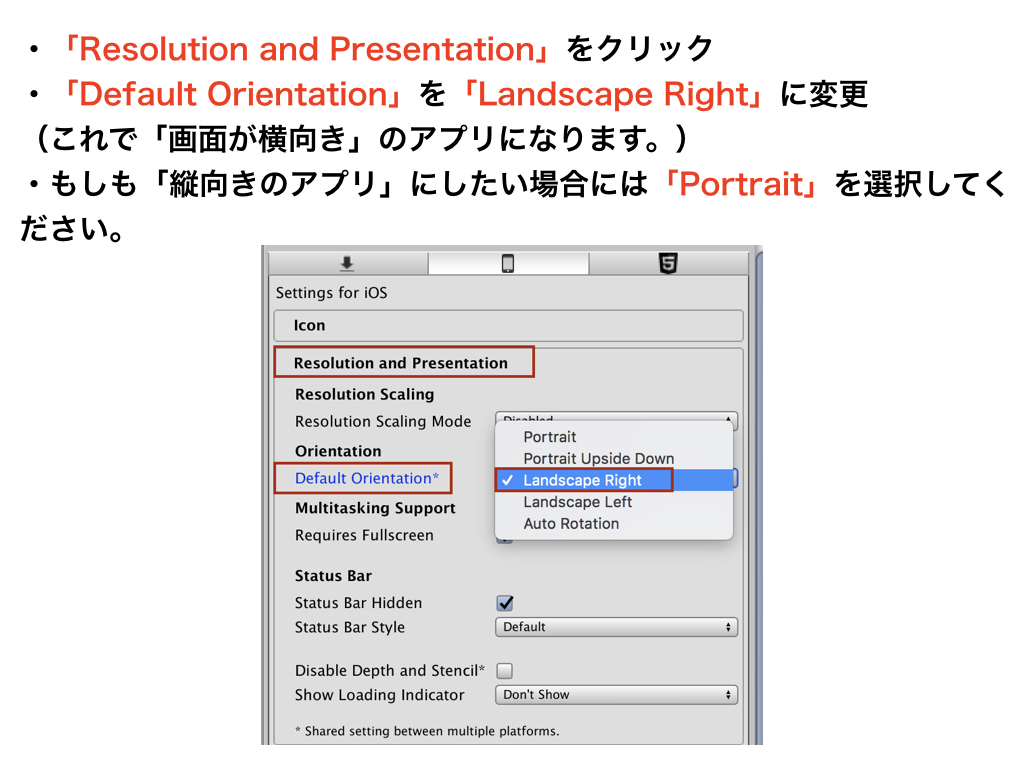
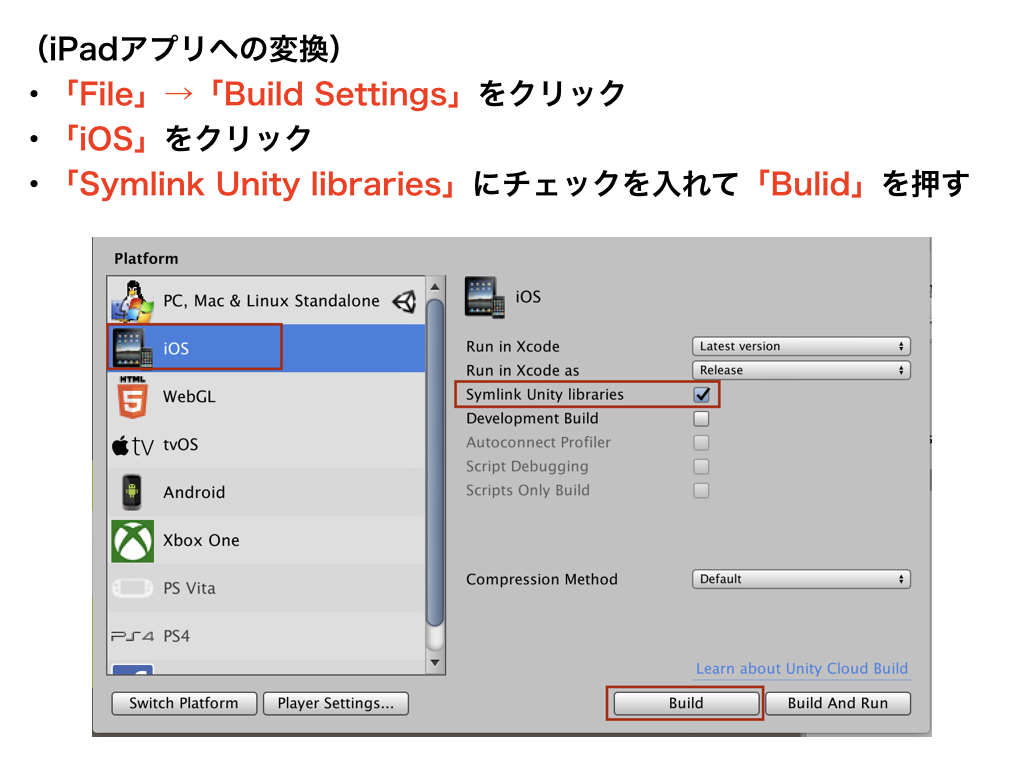
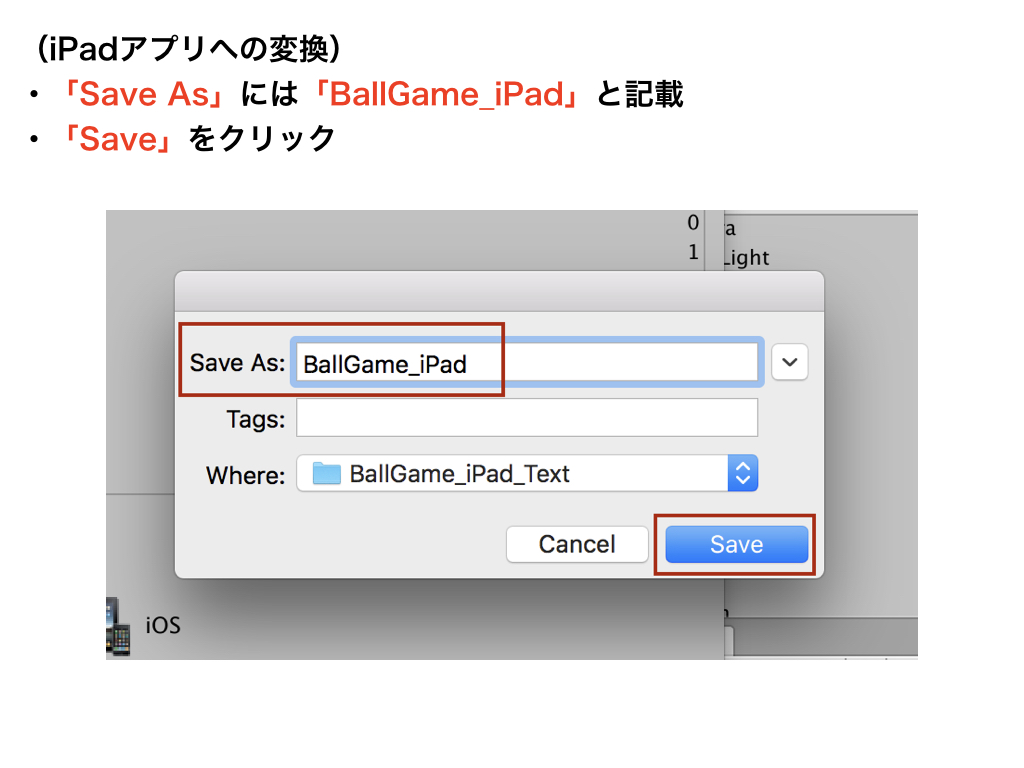
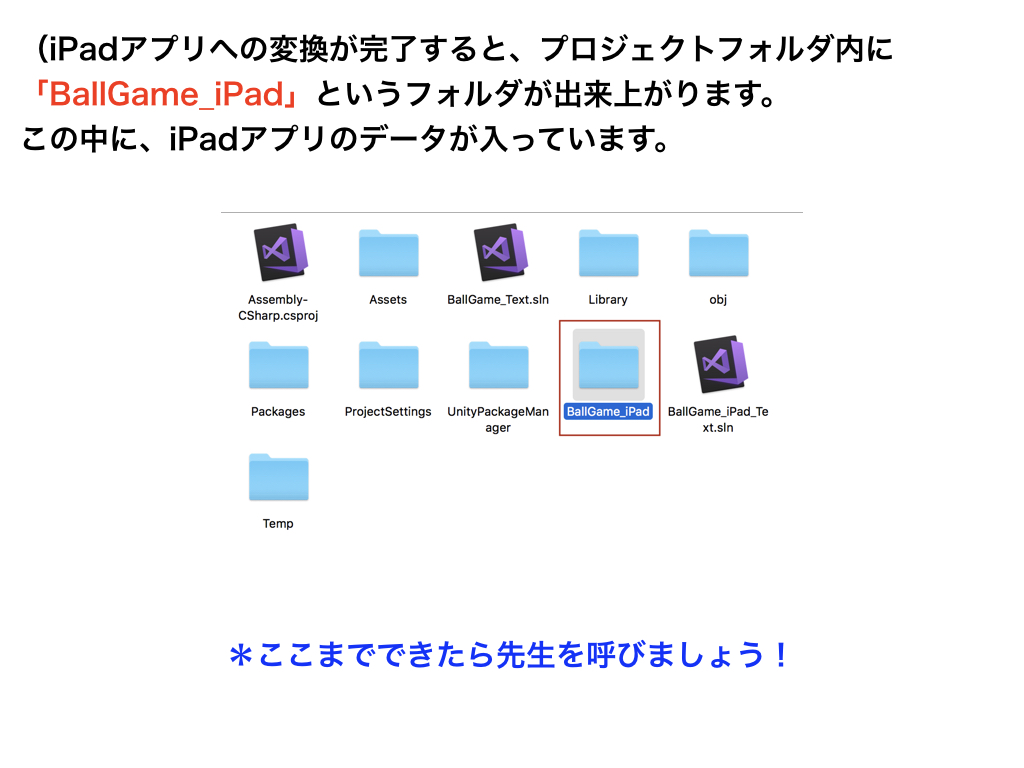
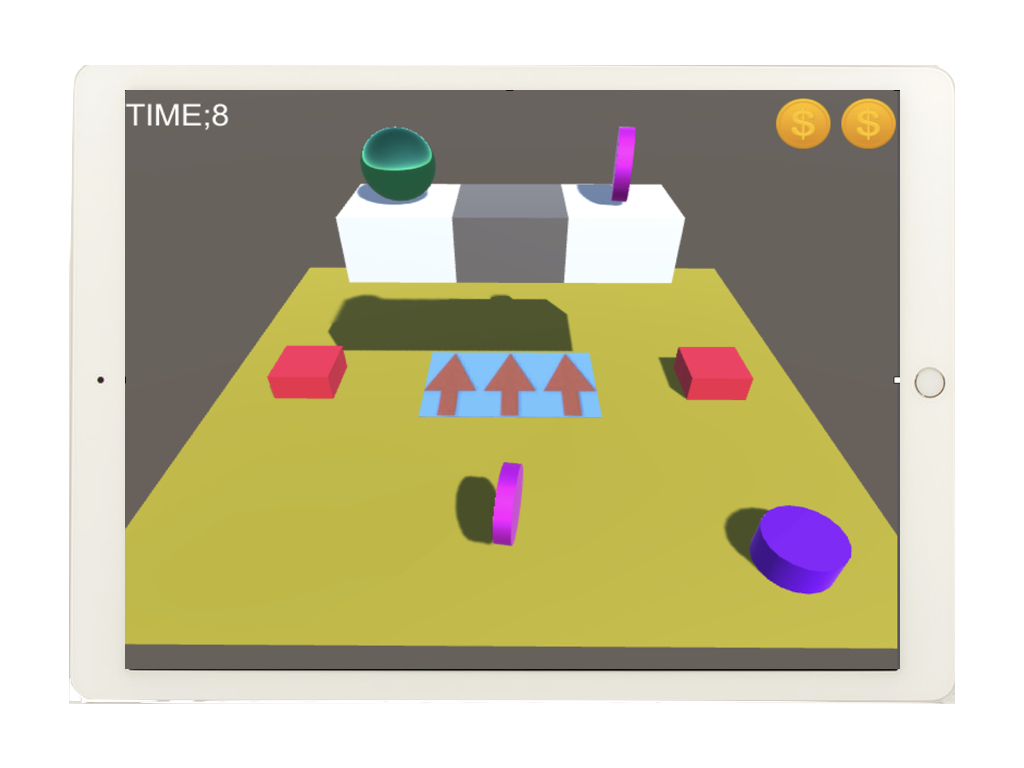
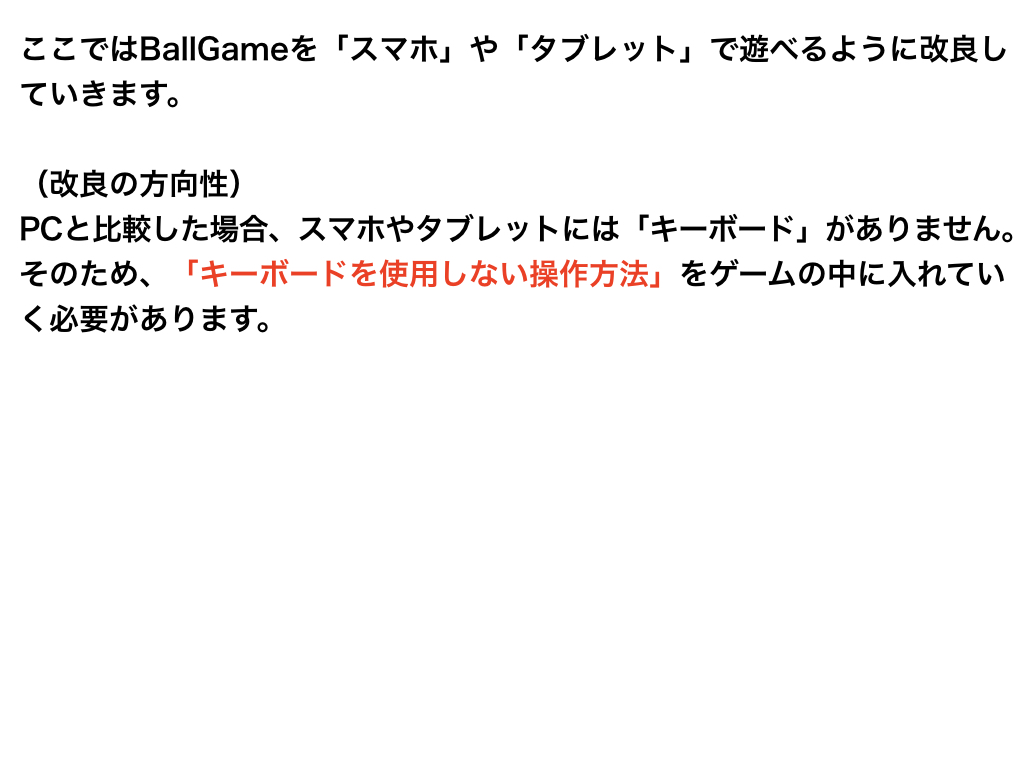
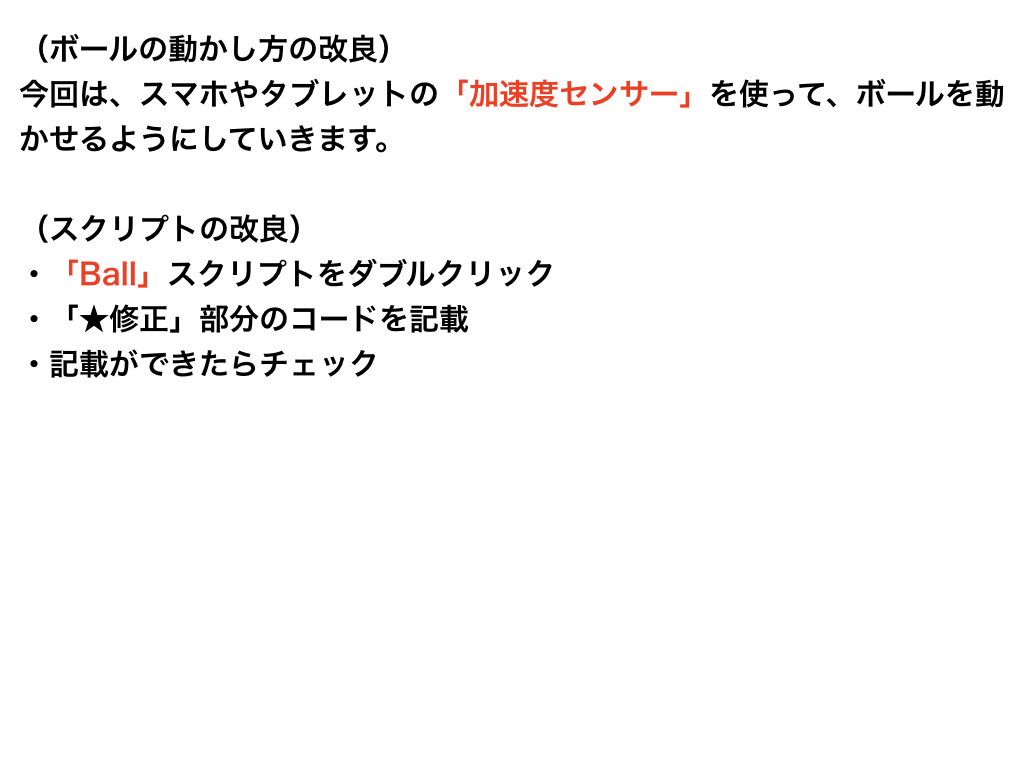
加速度センサーでボールを動かす
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
public class Ball : MonoBehaviour
{
public float moveSpeed;
private Rigidbody rb;
public AudioClip coinGet;
public float jumpSpeed;
private bool isJumping = false;
private int coinCount = 0;
public GameObject target;
void Start()
{
rb = GetComponent<Rigidbody>();
}
void Update()
{
// ★ボールの操作方法の修正
if (Application.isEditor)
{
float moveH = Input.GetAxis("Horizontal");
float moveV = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(moveH, 0, moveV);
rb.AddForce(movement * moveSpeed);
}
else
{
// スマホやタブレットの加速度センサーを活用する。
float moveH = Input.acceleration.x;
float moveV = Input.acceleration.y; // ここがポイント!
Vector3 movement = new Vector3(moveH, 0, moveV);
rb.AddForce(movement * moveSpeed);
}
if (Input.GetButtonDown("Jump") && isJumping == false)
{
rb.velocity = Vector3.up * jumpSpeed;
isJumping = true;
}
}
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("Coin"))
{
Destroy(other.gameObject);
AudioSource.PlayClipAtPoint(coinGet, transform.position);
coinCount += 1;
if (coinCount == 1)
{
target.SetActive(true);
}
if (coinCount == 2)
{
SceneManager.LoadScene("GameClear");
}
}
}
public int Coin()
{
return coinCount;
}
private void OnCollisionEnter(Collision other)
{
if (other.gameObject.CompareTag("Floor"))
{
isJumping = false;
}
}
}
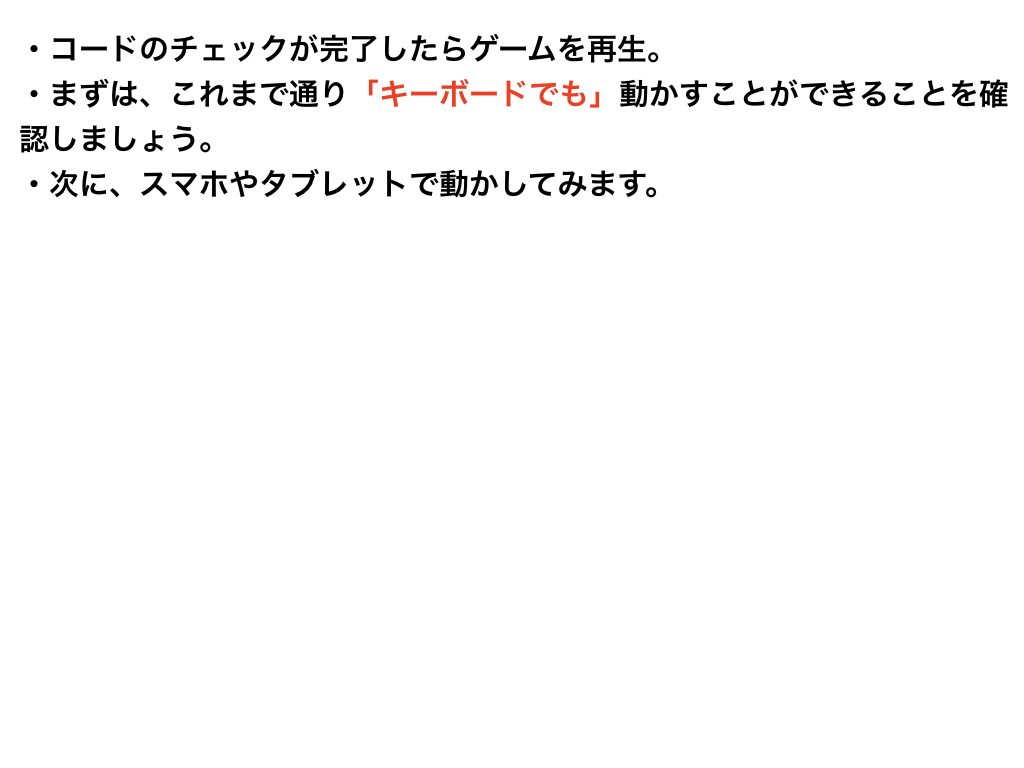
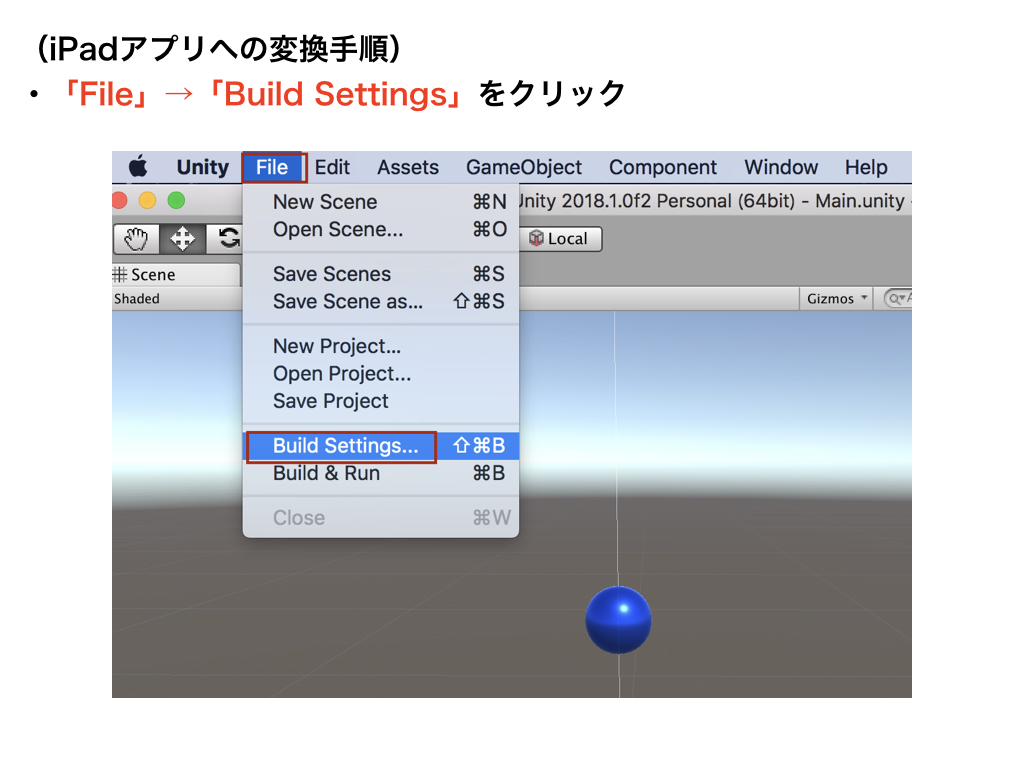
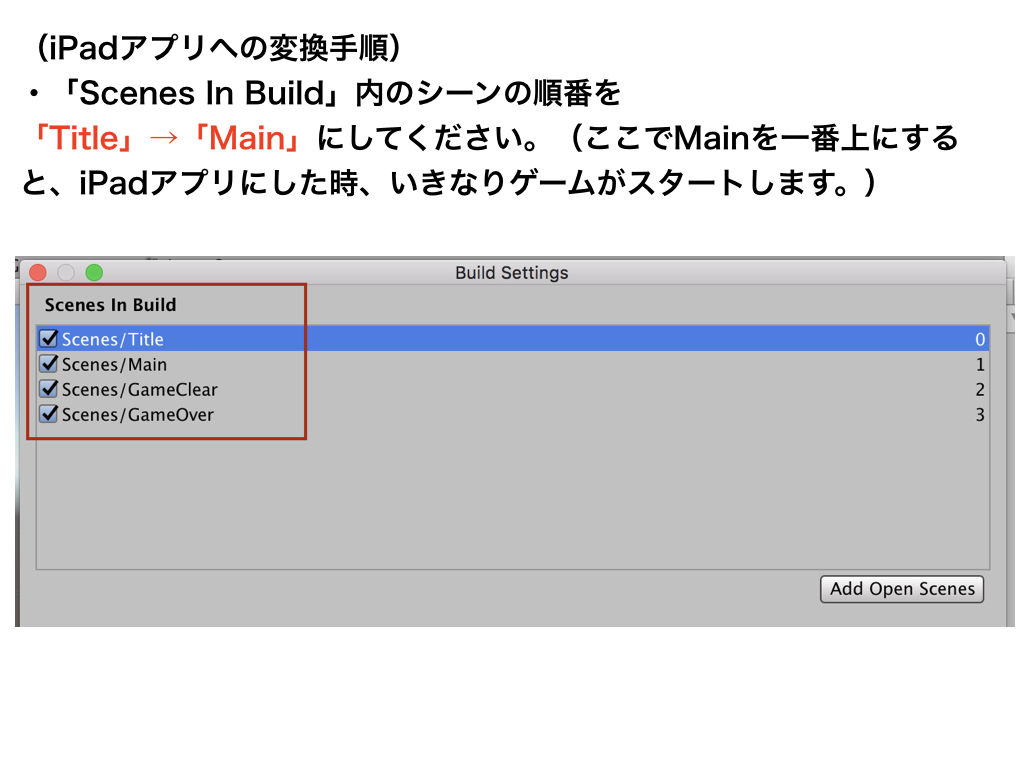
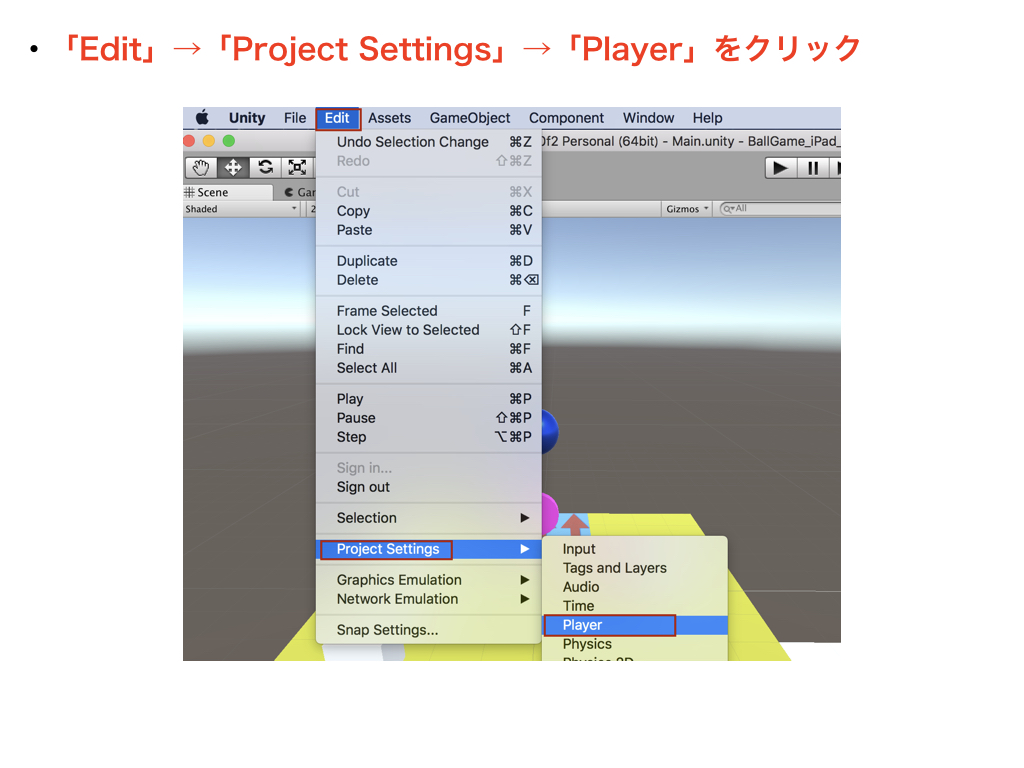
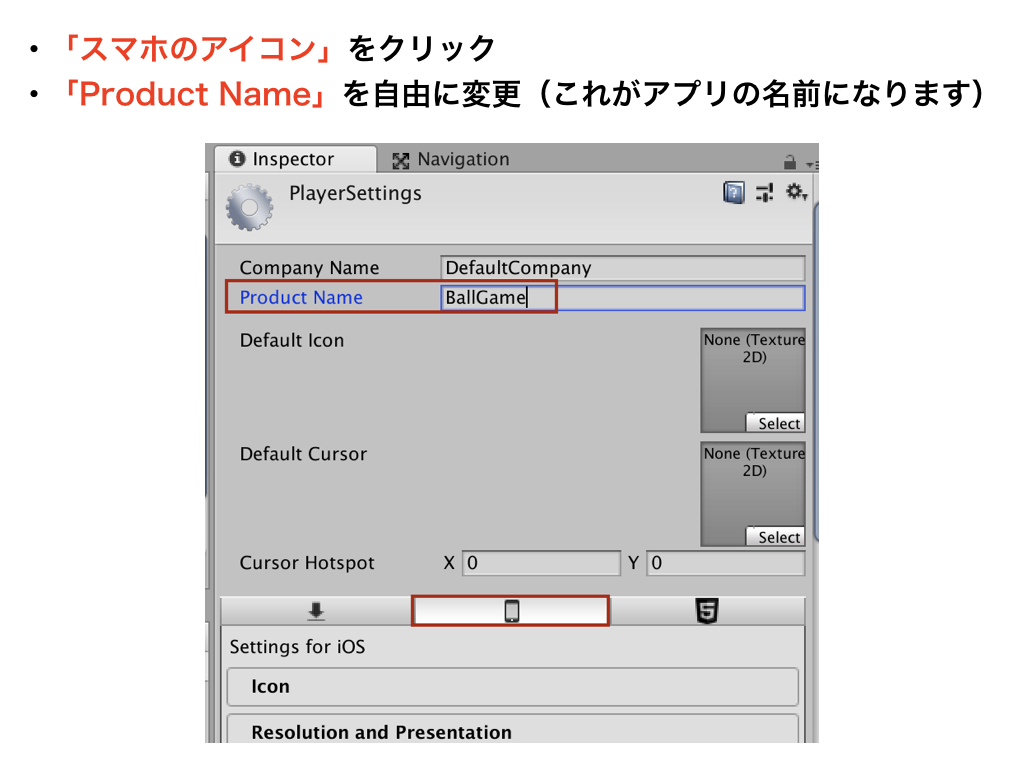
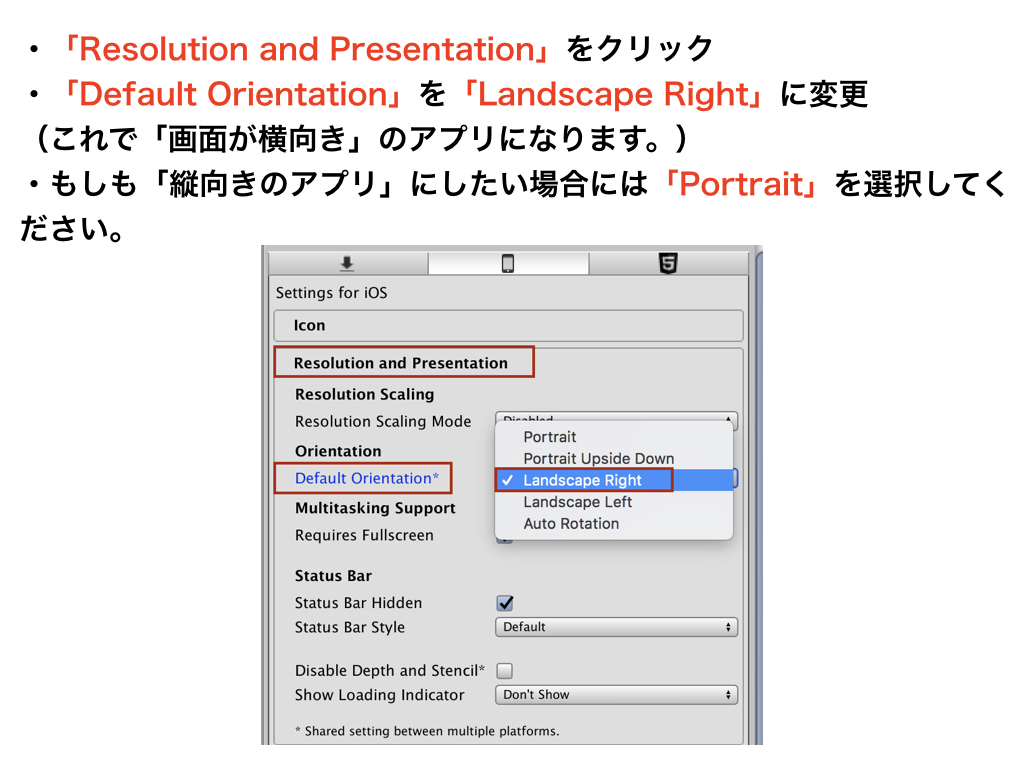
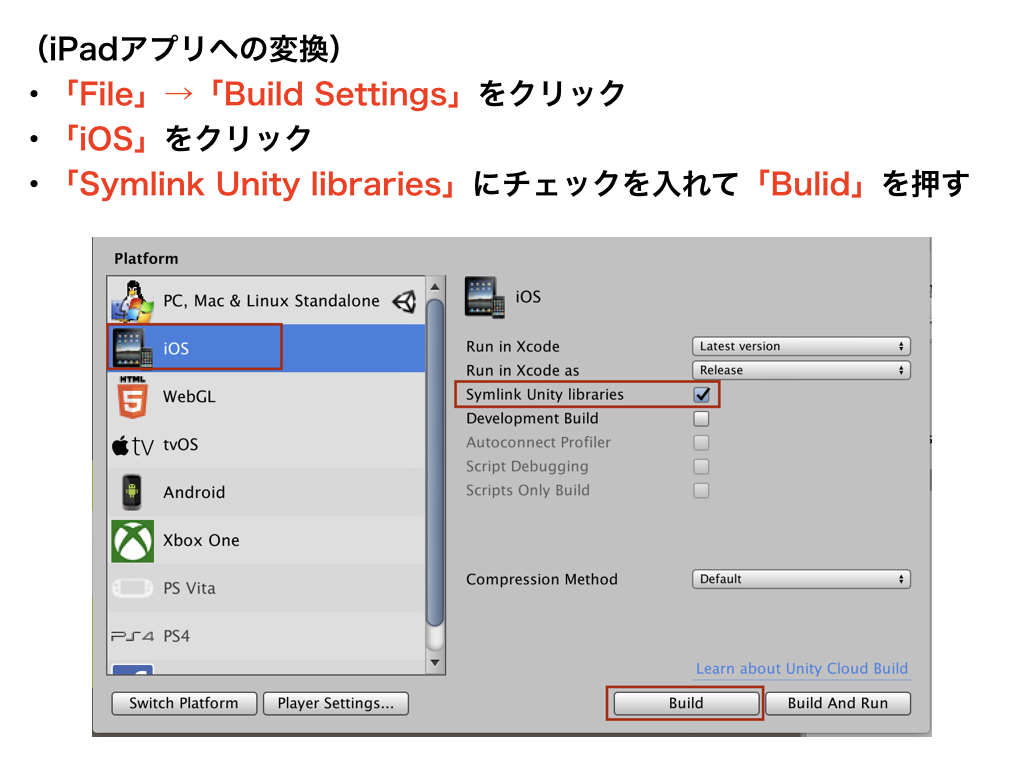
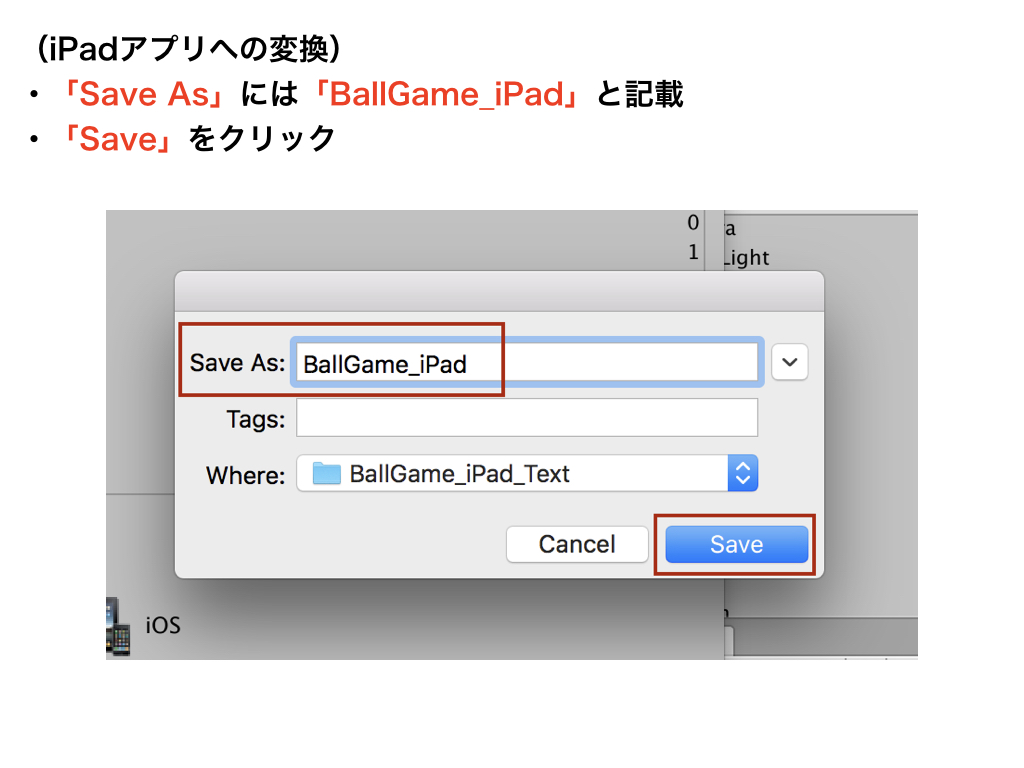
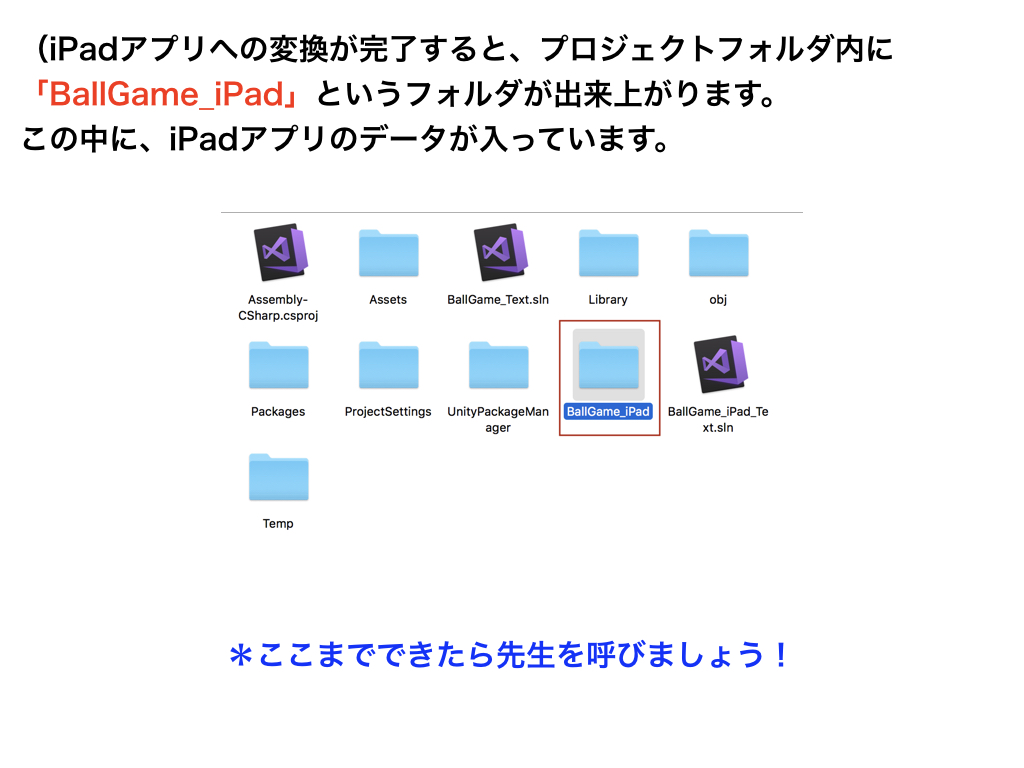
ボールを動かす方法を変える